Understanding JSON Serialization of NumPy Data Types
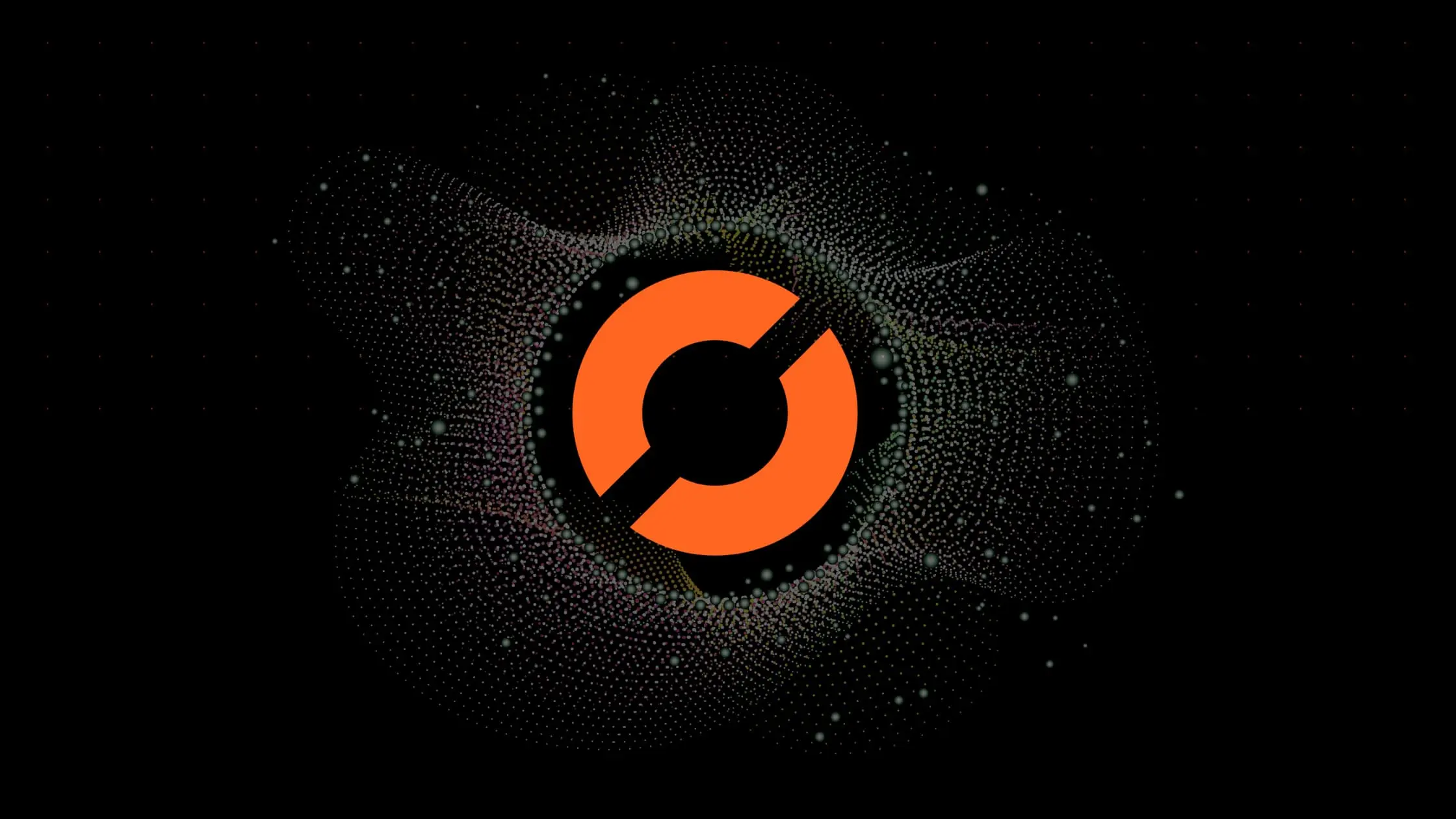
In the world of data science, the ability to serialize and deserialize data is crucial. It allows us to store and transmit data in a format that can be easily read and written by both humans and machines. One of the most popular formats for serialization is JSON (JavaScript Object Notation). However, when working with NumPy, a powerful library for numerical computations in Python, you might have noticed that not all data types are JSON serializable. In this blog post, we’ll delve into why this is the case.
Table of Contents
- What is JSON Serialization?
- NumPy and JSON Serialization
- Why Some NumPy Data Types are JSON Serializable and Others are Not
- Common Errors and How to Handle Them
- Conclusion
What is JSON Serialization?
Before we dive into the specifics of NumPy and JSON, let’s briefly discuss what JSON serialization is. JSON is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. Serialization, on the other hand, is the process of converting an object’s state to a byte stream, which can then be stored or transmitted and reconstructed later.
In Python, JSON serialization is handled by the json
module. This module provides methods for converting Python objects into JSON strings (json.dumps()
) and for converting JSON strings back into Python objects (json.loads()
).
NumPy and JSON Serialization
NumPy is a fundamental package for scientific computing in Python. It provides a high-performance multidimensional array object and tools for working with these arrays. However, when it comes to JSON serialization, not all NumPy data types are created equal.
The reason for this lies in the difference between native Python data types and NumPy data types. The json
module in Python can natively serialize basic data types like integers, floating-point numbers, strings, lists, and dictionaries. However, it doesn’t understand NumPy data types out of the box.
For example, if you try to serialize a NumPy array or a NumPy integer, you’ll get a TypeError
:
import json
import numpy as np
# NumPy array
arr = np.array([1, 2, 3])
json.dumps(arr)
# TypeError: Object of type ndarray is not JSON serializable
# NumPy integer
num = np.int64(10)
json.dumps(num)
# TypeError: Object of type int64 is not JSON serializable
Why Some NumPy Data Types are JSON Serializable and Others are Not
The reason behind the selective JSON serializability of NumPy data types stems from the json module’s default behavior. The json.dumps()
method utilizes a default()
method to convert Python objects into JSON serializable formats. However, this default method only recognizes basic Python data types.
To address this limitation, customizing the default()
method allows for the serialization of NumPy data types. For instance, defining a custom default()
method that converts NumPy arrays into lists and NumPy integers into standard integers enables successful serialization.
def default(obj):
if isinstance(obj, np.ndarray):
return obj.tolist()
elif isinstance(obj, np.integer):
return int(obj)
else:
return super().default(obj)
json.dumps(arr, default=default) # '[1, 2, 3]'
json.dumps(num, default=default) # '10'
This customization empowers the json module to understand and serialize NumPy data types effectively.
Common Errors and How to Handle Them
NumPy Object Arrays:
Error: NumPy object arrays (arrays with dtype object) may cause issues during serialization.
Handling: Convert the object array to a more suitable type or extract the elements into a homogeneous array.
# Example handling for object array
numpy_object_array = np.array(['a', 1, 2.5], dtype=object)
converted_array = np.array(numpy_object_array.tolist())
json_data = json.dumps(converted_array.tolist())
User-Defined Data Types:
- Error: User-defined data types in NumPy may not be directly serializable to JSON.
- Handling: Convert the data type to a JSON-compatible type or provide a custom serialization function.
# Example handling for user-defined data type
dtype_with_function = np.dtype([('name', 'S10'), ('value', 'f8')])
def serialize_custom_dtype(obj):
return {'name': obj['name'].decode('utf-8'), 'value': float(obj['value'])}
numpy_custom_dtype_array = np.array([(b'foo', 1.23)], dtype=dtype_with_function)
json_data = json.dumps(numpy_custom_dtype_array.tolist(), default=serialize_custom_dtype)
Large Arrays:
- Error: Serializing large NumPy arrays can lead to performance issues and high memory consumption.
- Handling: Consider alternative serialization formats like HDF5 for large datasets or implement chunked serialization.
# Example handling for large arrays
large_numpy_array = np.random.rand(10000, 10000)
json_data = json.dumps(large_numpy_array.tolist()) # Performance warning for large arrays
# Consider using HDF5 for large datasets
large_numpy_array.tofile('large_array.h5', format='h5')
Conclusion
In conclusion, the reason why some NumPy data types are JSON serializable and others are not is because the json
module in Python only knows how to serialize basic Python data types by default. However, by defining a custom default()
method, you can teach it how to serialize other data types, including NumPy data types.
Understanding this can help you avoid errors when trying to serialize NumPy data and can give you more flexibility in how you store and transmit your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.