Understanding and Solving the ''numpy.ndarray' object is not callable' Error in Python
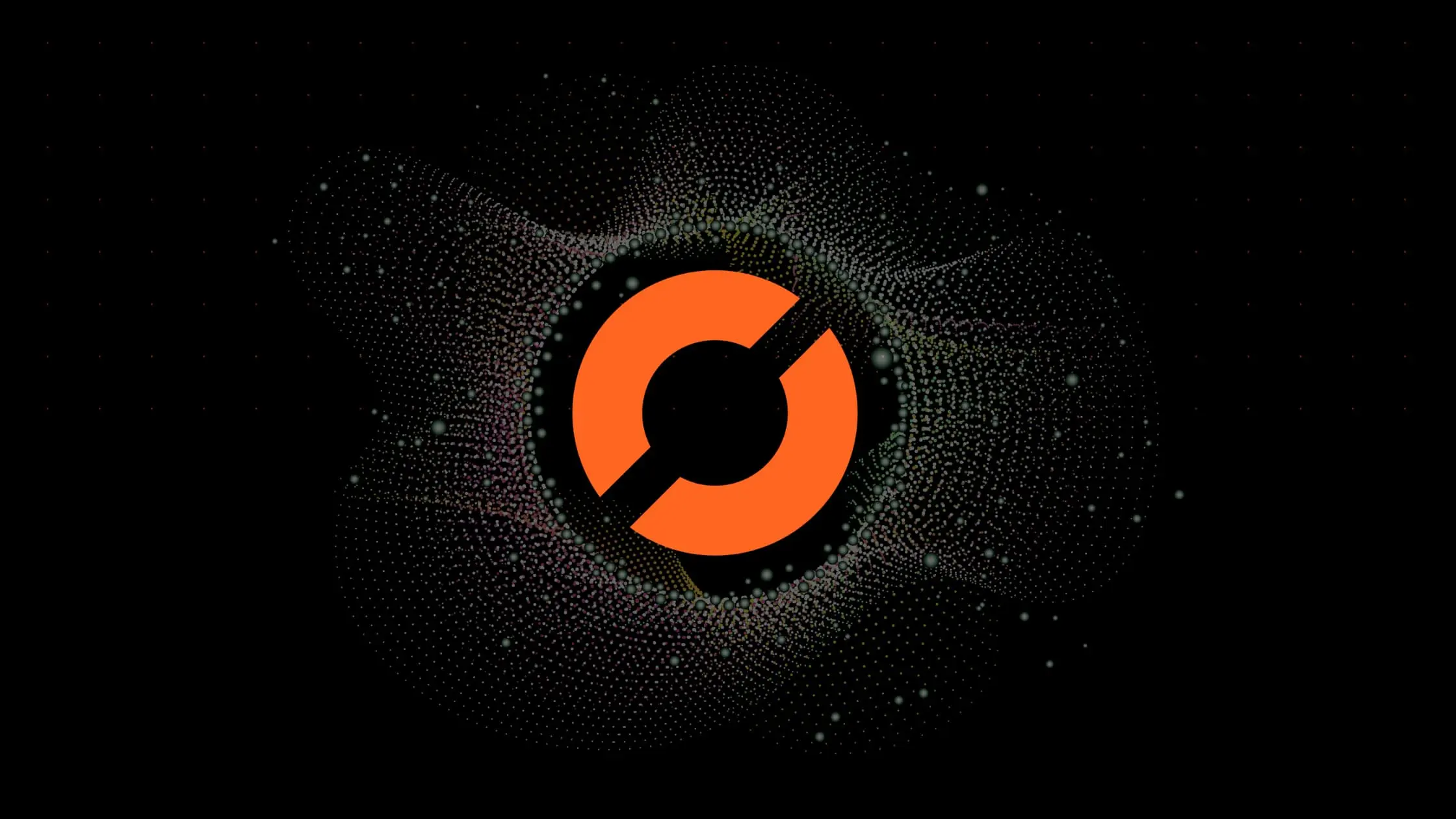
Python is a versatile language widely used in data science due to its simplicity and the vast array of libraries it offers. One such library is NumPy, which provides a powerful object: the n-dimensional array, or ndarray. However, a common error that data scientists encounter when working with ndarrays is the “‘numpy.ndarray’ object is not callable” error. This blog post will delve into the root cause of this error and provide solutions to fix it.
Understanding the Error
Before we dive into the solutions, let’s first understand the error message. The “‘numpy.ndarray’ object is not callable” error typically arises when you try to call an ndarray object as if it were a function. In Python, “callable” means that an object can be “called” like a function. However, an ndarray is not a function, but a data structure, hence it cannot be called.
Here’s an example of a situation that would trigger this error:
import numpy as np
# Create a numpy array
arr = np.array([1, 2, 3, 4, 5])
# Attempt to call the array as a function
result = arr(0)
In the above code, arr
is a numpy array, not a function. Therefore, trying to call arr(0)
will result in the 'numpy.ndarray' object is not callable
error.
Common Causes and Solutions
Cause 1: Misuse of Parentheses
One of the most common causes of this error is the misuse of parentheses. When you want to access an element in a numpy array, you should use square brackets, not parentheses.
Solution: Replace parentheses with square brackets.
# Correct way to access an element in a numpy array
result = arr[0]
Cause 2: Overwriting a Function Name
Another common cause is overwriting a function name with a numpy array. For instance, if you create a numpy array and name it np
, you will overwrite the numpy module.
import numpy as np
def example():
return 'Saturn Cloud!'
example = np.array([1, 2, 3, 4])
example() # -> this will throw an error
Solution: Avoid using function names as variable names.
import numpy as np
def example():
return 'Saturn Cloud!'
# Correct way to name a numpy array
arr = np.array([1, 2, 3, 4, 5])
print(example())
Cause 3: Overriding a built-in function by mistake
Sometimes we declared a list
variable and set it to a NumPy array.
In the following example, Tthe variable name clashes with the built-in list
function, so when we try to call the function later on in our code, we actually call the NumPy array.
import numpy as np
# Create an array that has a name overriding a built-in function
list = np.array([1, 2, 3, 4])
list('abc')
Solution: Give the variable a different name that doesn’t clash with built-in functions
import numpy as np
# Create an array that has a name overriding a built-in function
list_a = np.array([1, 2, 3, 4])
print(list('abc'))
Cause 4: Error in Accessing DataFrame Values
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
data = df['A'].values() # -> this will throw an error
Sometimes, the error occurs when you attempts to access the values of the DataFrame using df['A'].values()
. However, the correct attribute to access the underlying NumPy array is df['A'].values
without the parentheses. The values attribute is not callable.
Solution: Use values
without parentheses. So, the corrected code looks like this:
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
data = df['A'].values
Output:
[1 2 3]
Conclusion
The 'numpy.ndarray' object is not callable
error in Python is a common stumbling block for data scientists. However, by understanding the root cause of the error and applying the solutions provided in this post, you can avoid this pitfall and work more efficiently with numpy arrays.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.