Understanding and Resolving ValueError: The Truth Value of a DataFrame is Ambiguous
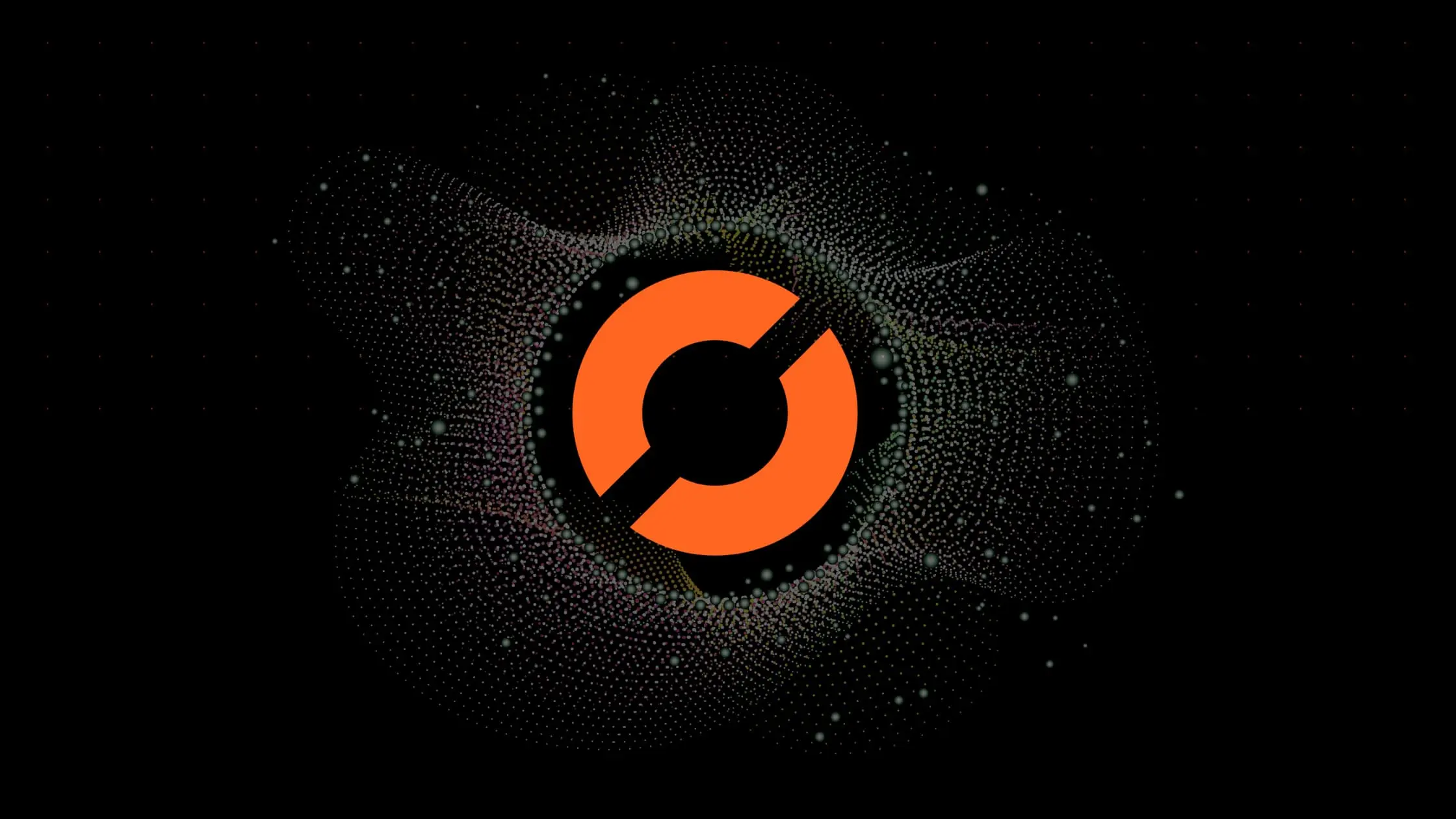
Understanding and Resolving ValueError: The Truth Value of a DataFrame is Ambiguous
In this blog post, we’ll delve into the root cause of this error and explore how to resolve it using a.empty
, a.any()
, a.item()
, or a.all()
, keeping in mind that the relevance of the information will depend on the version of the Pandas library used, as there may be updates or changes in later versions.
The Root Cause
The ValueError: The truth value of a DataFrame is ambiguous
error is thrown when you’re trying to use a DataFrame in a context where a boolean is expected. This is because a DataFrame is a two-dimensional structure, and Python doesn’t know how to implicitly convert it into a single boolean value.
For instance, consider the following code:
import pandas as pd
df = pd.DataFrame({'A': ['foo', 'bar', 'baz'], 'B': ['qux', 'quux', 'quuz']})
if df['A'] == 'foo':
print("Found 'foo'")
This code will throw the ValueError
because it’s trying to compare a Series (the result of df['A']
) with a string (‘foo’). Python doesn’t know how to interpret this comparison.
The Solution
To resolve this error, you need to use one of the following methods: a.empty
, a.any()
, a.item()
, or a.all()
. These methods allow you to explicitly define how Python should interpret the DataFrame in a boolean context.
a.empty
If you want to check that the DataFrame is not empty first you can use a.empty
The a.empty
attribute returns True
if the DataFrame is empty and False
otherwise:
if not df.empty:
if (df['A'] == 'foo').any():
print("Found 'foo'")
a.any()
The a.any()
method returns True
if any element in the DataFrame is True
and False
otherwise. You can use it to check if any element in a DataFrame meets a certain condition:
if (df['A'] == 'foo').any():
print("Found 'foo'")
a.item()
The a.item()
method converts a single-element DataFrame or Series into a scalar. If the DataFrame or Series has more than one element, it will throw a ValueError
. Here’s how you can use it:
if (df['A'] == 'foo').any().item():
print("Found 'foo'")
a.all()
The a.all()
method returns True
if all elements in the DataFrame are True
and False
otherwise. You can use it to check if all elements in a DataFrame meet a certain condition:
if (df['A'] == 'foo').all():
print("All elements are 'foo'")
Conclusion
The ValueError: The truth value of a DataFrame is ambiguous
error can be a bit confusing at first, but once you understand its root cause, it’s easy to resolve. By using a.empty
, a.any()
, a.item()
, or a.all()
, you can explicitly define how Python should interpret a DataFrame in a boolean context, thereby avoiding this error.
Remember, when working with DataFrames, always be explicit about your intentions. This will not only prevent errors but also make your code easier to understand and maintain.
Happy coding!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.