TensorFlow How to Predict from a SavedModel
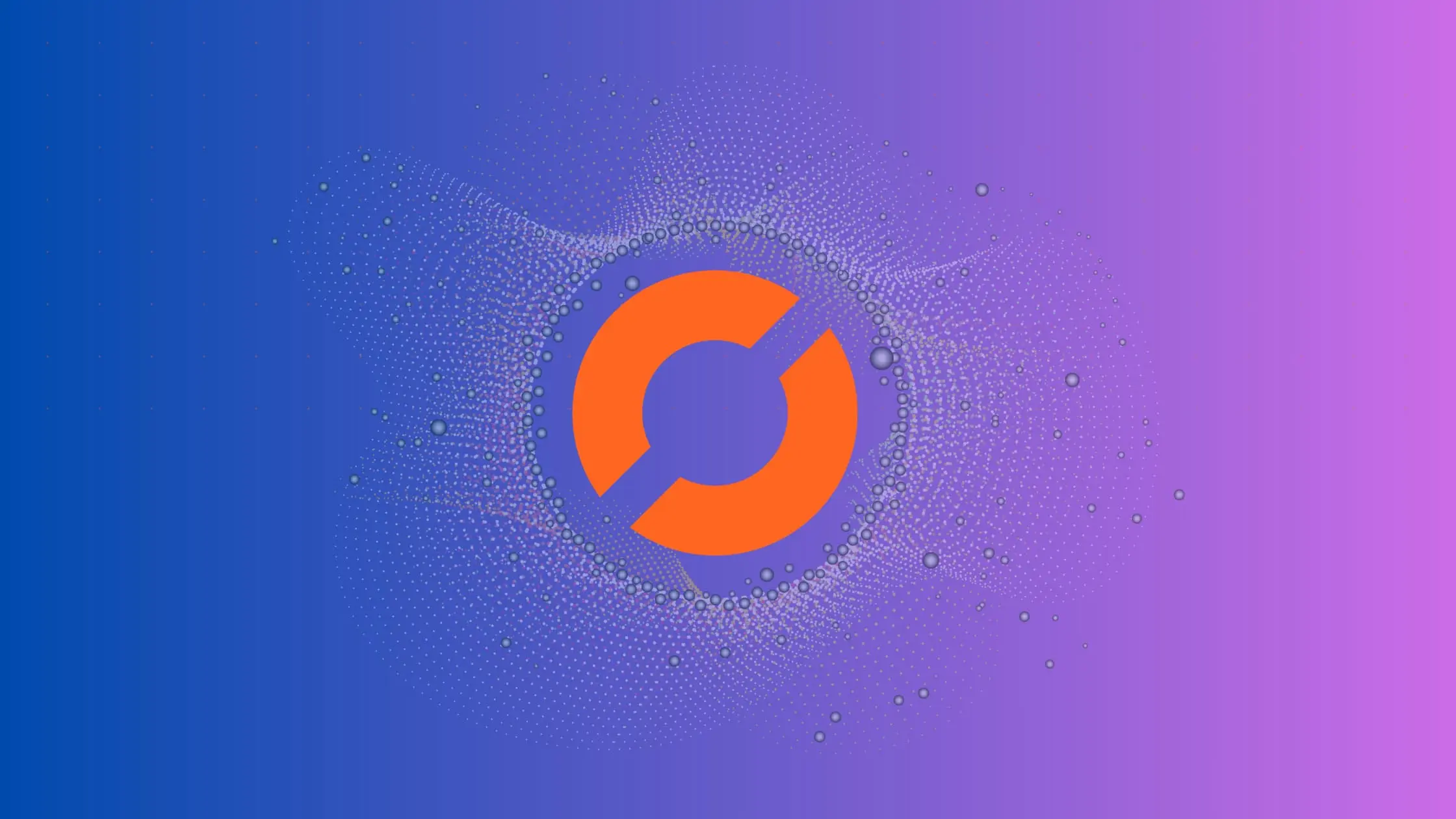
As a data scientist, you know the importance of creating accurate and efficient predictive models. TensorFlow is one of the most popular open-source machine learning frameworks that can help you achieve this goal. In this article, we will discuss how to predict from a SavedModel using TensorFlow.
Table of Contents
- What is TensorFlow?
- What is a SavedModel?
- How to Predict from a SavedModel?
- Pros and Cons of Predicting from a SavedModel
- Common Errors and How to Handle Them
- Conclusion
What is TensorFlow?
TensorFlow is an open-source machine learning framework developed by Google. It allows you to build, train, and deploy machine learning models for a wide range of applications. TensorFlow offers a high level of flexibility and scalability, making it suitable for both small and large-scale projects.
What is a SavedModel?
A SavedModel is a serialized TensorFlow model that can be loaded and used for inference. It contains both the model architecture and the trained weights, making it easy to deploy and use on different platforms. SavedModels are saved in a standard format that can be used with a variety of programming languages, including Python and C++.
Saving a Model in TensorFlow
To save a trained model in TensorFlow, you can use the tf.saved_model.save() function. This function exports the model to the SavedModel format, making it easy to reload later. Here’s a simple example:
import tensorflow as tf
# Assume 'model' is your trained TensorFlow model
model.save("path/to/saved_model")
How to Predict from a SavedModel?
Predicting from a SavedModel is a straightforward process. You can load the SavedModel and use it to make predictions on new data. The following steps will guide you through the process of predicting from a SavedModel using TensorFlow.
Step 1: Load the SavedModel
The first step is to load the SavedModel into your Python environment. This can be done using the tf.saved_model.load
function. The tf.saved_model.load
function takes the path to the SavedModel directory and returns a tf.saved_model.load
object.
import tensorflow as tf
# Load the SavedModel
model = tf.saved_model.load('path/to/saved/model')
Step 2: Prepare the Input Data
Once you have loaded the SavedModel, you need to prepare the input data for prediction. The input data should be in the same format as the data used to train the model. You can use the tf.data.Dataset
API to prepare the input data.
import numpy as np
# Prepare the input data
input_data = np.array([[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12]], dtype=np.float32)
dataset = tf.data.Dataset.from_tensor_slices(input_data).batch(1)
Step 3: Make Predictions
Once you have loaded the SavedModel and prepared the input data, you can use the model
object to make predictions on the input data.
# Make predictions
for data in dataset:
predictions = model(data)
print(predictions)
The model
object takes the input data as input and returns the predictions as output. In this example, we are using a simple loop to iterate over the input data and make predictions batch by batch.
Step 4: Interpret the Predictions
The final step is to interpret the predictions and use them for your application. The format of the predictions will depend on the model architecture and the problem you are trying to solve. You can use various evaluation metrics to measure the performance of the model and adjust the model parameters accordingly.
Pros and Cons of Predicting from a SavedModel
Pros | Cons |
---|---|
Easy deployment | Model size can be large |
Fast inference speed | Limited support for dynamic architectures |
Platform-independent | Can be challenging to interpret errors |
Supports TensorFlow Serving | May require additional dependencies |
Common Errors and How to Handle Them
Error 1: Model Not Found
If you encounter a “Model not found” error, double-check the path to your SavedModel:
try:
loaded_model = tf.keras.models.load_model("incorrect/path/to/saved_model")
except OSError:
print("Model not found. Please provide the correct path.")
Error 2: Incompatible TensorFlow Versions
Ensure compatibility between the TensorFlow version used for training and prediction:
import tensorflow as tf
tf_version = tf.__version__
if not tf_version.startswith("2."):
raise ValueError(f"Unsupported TensorFlow version: {tf_version}. Use TensorFlow 2.x.")
Conclusion
In this article, we have discussed how to predict from a SavedModel using TensorFlow. Predicting from a SavedModel is a simple and straightforward process that can be done in just a few lines of code. TensorFlow offers a high level of flexibility and scalability, making it suitable for a wide range of machine learning applications. With the knowledge gained from this article, you can start building accurate and efficient predictive models using TensorFlow.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.