Substring Algorithm: A Data Scientist's Guide to Efficient String Searching
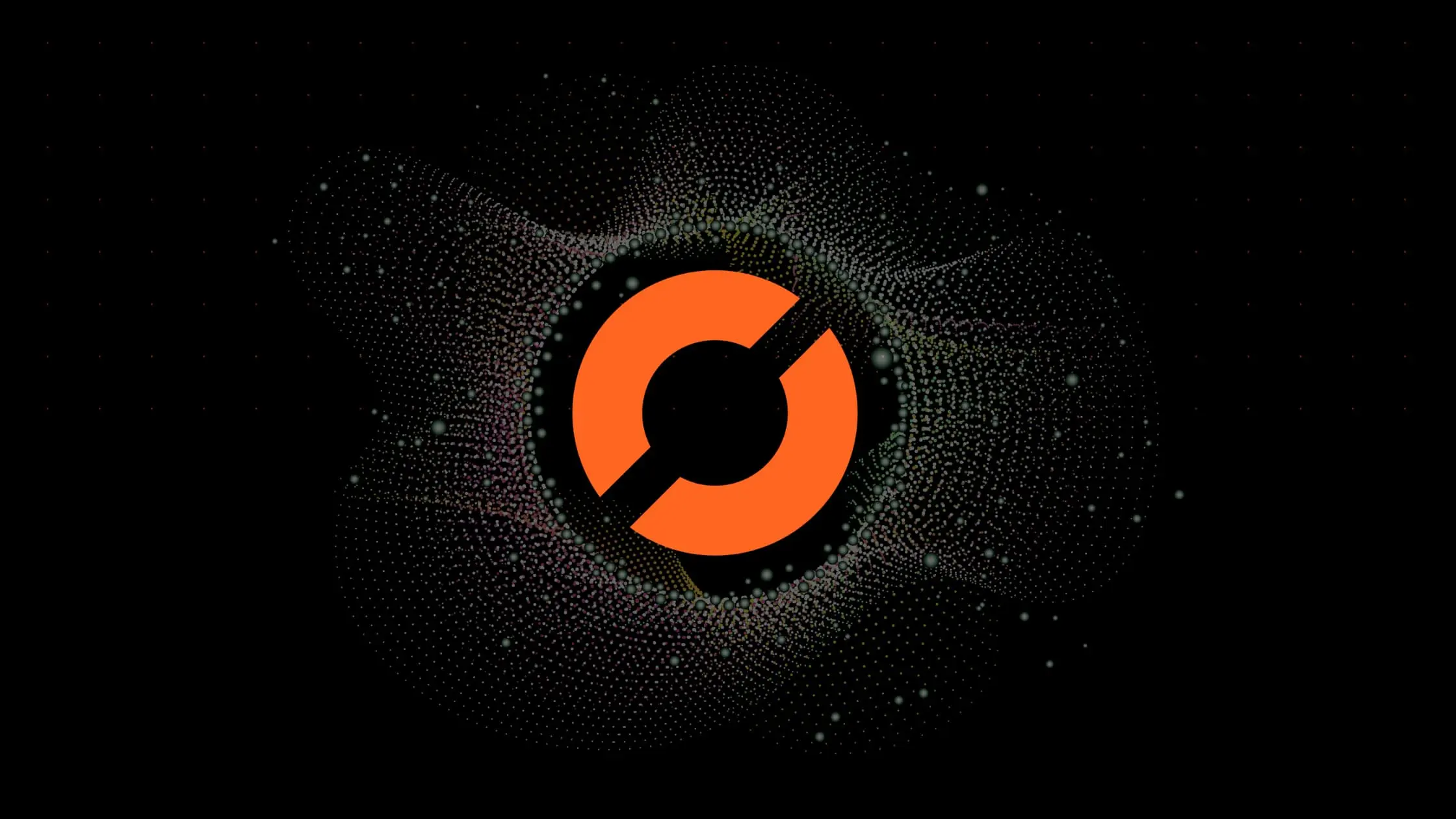
Table of Contents:
Table of Contents
- Introduction
- The Naive Approach: Brute Force
- Knuth-Morris-Pratt (KMP) Algorithm
- Boyer-Moore Algorithm
- Rabin-Karp Algorithm
- Choosing the Right Substring Algorithm
- Conclusion
Introduction
As a data scientist or software engineer, you often encounter situations where you need to search for specific patterns or substrings within a given string. Efficiently finding substrings is a crucial task in various domains, such as natural language processing, text mining, and data analysis. In this article, we will explore different substring algorithms, their advantages, and how to choose the most suitable algorithm for your specific use case. So let’s dive into the world of substring algorithms and enhance our string searching capabilities!
What is a Substring?
Before we dive into the algorithms, let’s define what a substring is. In simple terms, a substring is a contiguous sequence of characters within a larger string. For example, in the string “Hello, World!”, “World” is a substring.
The Naive Approach: Brute Force
The most straightforward approach to find a substring within a string is to use a brute force algorithm. This algorithm involves checking each character of the string against the first character of the substring, and if a match is found, comparing subsequent characters until either a mismatch occurs or the complete substring is found. While simple to implement, this approach can be inefficient for large strings or complex substrings.
def brute_force_search(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
return i # Match found at position i
return -1 # Pattern not found
Knuth-Morris-Pratt (KMP) Algorithm
The Knuth-Morris-Pratt (KMP) algorithm is a more efficient approach for substring searching. It utilizes the concept of prefix matching to avoid unnecessary character comparisons. The algorithm preprocesses the substring and constructs a lookup table, called the “failure function,” which helps determine the next character to compare in case of a mismatch.
The KMP algorithm has a time complexity of O(n), where n is the length of the string being searched. This makes it significantly faster than the brute force approach, especially for large strings or complex substrings.
def compute_lps_array(pattern):
m = len(pattern)
lps = [0] * m
j = 0
for i in range(1, m):
while j > 0 and pattern[i] != pattern[j]:
j = lps[j - 1]
if pattern[i] == pattern[j]:
j += 1
lps[i] = j
return lps
def kmp_search(text, pattern):
n = len(text)
m = len(pattern)
lps = compute_lps_array(pattern)
i = j = 0
while i < n:
if pattern[j] == text[i]:
i += 1
j += 1
if j == m:
return i - j # Match found at position i-j
else:
if j != 0:
j = lps[j - 1]
else:
i += 1
return -1 # Pattern not found
Boyer-Moore Algorithm
The Boyer-Moore algorithm is another popular and efficient substring search algorithm. It leverages two rules: the “bad character rule” and the “good suffix rule.” The bad character rule allows skipping comparisons by analyzing the mismatched character, while the good suffix rule skips ahead if a suffix of the substring matches the string.
Boyer-Moore is especially useful when searching for substrings with a relatively small alphabet size. It has an average-case time complexity of O(n/m), where n is the length of the string and m is the length of the substring.
def boyer_moore_search(text, pattern):
n = len(text)
m = len(pattern)
last_occurrence = {pattern[i]: i for i in range(m)}
i = m - 1
j = m - 1
while i < n:
if pattern[j] == text[i]:
if j == 0:
return i # Match found at position i
i -= 1
j -= 1
else:
i += m - min(j, 1 + last_occurrence.get(text[i], -1))
j = m - 1
return -1 # Pattern not found
Rabin-Karp Algorithm
The Rabin-Karp algorithm is a rolling hash-based algorithm that provides a probabilistic approach to substring search. It computes a hash value for the substring and compares it with the hash values of potential matching substrings in the string. If the hash values match, it performs a character-by-character comparison to confirm the match.
The advantage of the Rabin-Karp algorithm is that it can efficiently handle multiple pattern searches within a single string. However, it may produce false positives due to hash collisions and requires additional checks to eliminate them. The time complexity of the Rabin-Karp algorithm is O((n-m+1)m), where n is the length of the string and m is the length of the substring.
def rabin_karp_search(text, pattern):
n = len(text)
m = len(pattern)
d = 256 # Number of characters in the input alphabet
q = 101 # A prime number
def hash_function(string):
h = 0
for i in range(m):
h = (h * d + ord(string[i])) % q
return h
pattern_hash = hash_function(pattern)
text_hash = hash_function(text[:m])
for i in range(n - m + 1):
if pattern_hash == text_hash and pattern == text[i:i + m]:
return i # Match found at position i
if i < n - m:
text_hash = (d * (text_hash - ord(text[i]) * (d ** (m - 1))) + ord(text[i + m])) % q
if text_hash < 0:
text_hash += q
return -1 # Pattern not found
Let’s see an example:
# Sample text and patterns
sample_text = "ABABCABABABCABCABABC"
patterns = ["ABABC", "ABCAB", "XYZ"]
# Test the Brute Force algorithm
print("Brute Force:")
for pattern in patterns:
result = brute_force_search(sample_text, pattern)
print(f"Pattern '{pattern}' found at position {result}" if result != -1 else f"Pattern '{pattern}' not found")
# Test the KMP algorithm
print("\nKnuth-Morris-Pratt (KMP):")
for pattern in patterns:
result = kmp_search(sample_text, pattern)
print(f"Pattern '{pattern}' found at position {result}" if result != -1 else f"Pattern '{pattern}' not found")
# Test the Boyer-Moore algorithm
print("\nBoyer-Moore:")
for pattern in patterns:
result = boyer_moore_search(sample_text, pattern)
print(f"Pattern '{pattern}' found at position {result}" if result != -1 else f"Pattern '{pattern}' not found")
# Test the Rabin-Karp algorithm
print("\nRabin-Karp:")
for pattern in patterns:
result = rabin_karp_search(sample_text, pattern)
print(f"Pattern '{pattern}' found at position {result}" if result != -1 else f"Pattern '{pattern}' not found")
Output:
Brute Force:
Pattern 'ABABC' found at position 0
Pattern 'ABCAB' found at position 2
Pattern 'XYZ' not found
Knuth-Morris-Pratt (KMP):
Pattern 'ABABC' found at position 0
Pattern 'ABCAB' found at position 2
Pattern 'XYZ' not found
Boyer-Moore:
Pattern 'ABABC' found at position 0
Pattern 'ABCAB' found at position 2
Pattern 'XYZ' not found
Rabin-Karp:
Pattern 'ABABC' found at position 0
Pattern 'ABCAB' found at position 2
Pattern 'XYZ' not found
Choosing the Right Substring Algorithm
The choice of a substring algorithm depends on various factors, including the size of the string, the complexity of the substring, and the expected search frequency. Here are some guidelines to help you make an informed decision:
- For small strings or simple substrings, the brute force approach may suffice due to its simplicity.
- If you are dealing with large strings or complex substrings, consider using the KMP or Boyer-Moore algorithms for improved performance.
- If you need to search for multiple patterns within a single string, the Rabin-Karp algorithm can be a suitable choice.
Conclusion
Efficient substring searching is a fundamental task in data science and software engineering. By utilizing advanced substring algorithms like KMP, Boyer-Moore, or Rabin-Karp, you can significantly enhance the performance of your string search operations. Understanding the strengths and weaknesses of each algorithm will enable you to choose the most appropriate solution based on your specific requirements. So go ahead, apply these algorithms to your projects, and unlock the power of efficient string searching!
The choice of the substring algorithm depends on the context and the specific requirements of your problem. By considering factors such as string size, substring complexity, and search frequency, you can make an informed decision and optimize your string searching operations.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.