Spark: Understanding Salting and Its Role in Handling Skewed Data
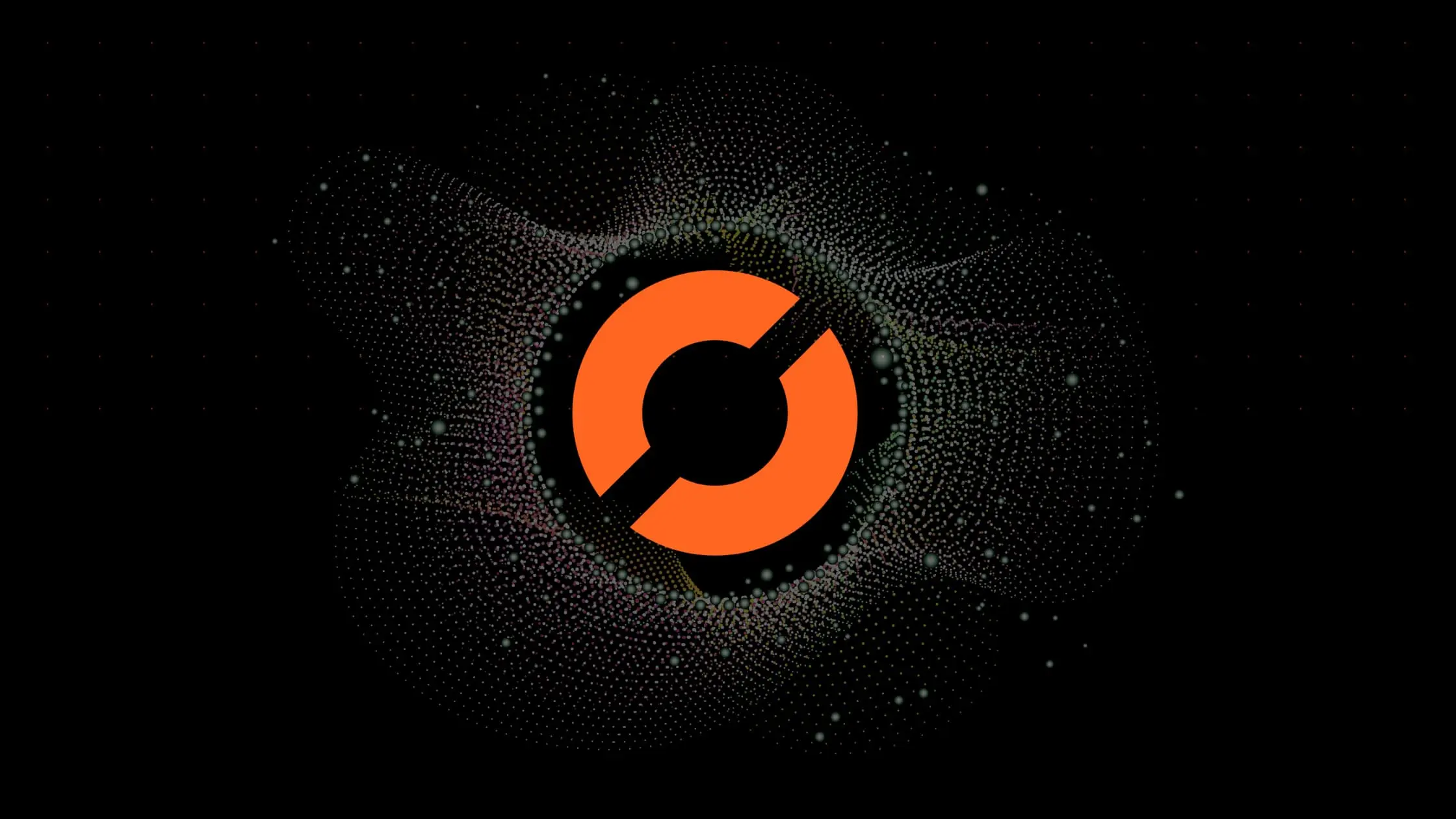
Data skewness is a common problem in big data processing. It can lead to inefficient resource utilization and longer processing times. Apache Spark, a popular big data processing framework, provides a technique known as ‘salting’ to handle skewed data. This blog post will delve into the concept of salting and how it helps in dealing with skewed data in Spark.
Table of Contents
What is Skewed Data?
Before we dive into salting, let’s understand what skewed data is. In a distributed system like Spark, data is divided into partitions for parallel processing. Ideally, each partition should have an equal amount of data. However, in real-world scenarios, this is rarely the case. Some partitions may have more data than others, leading to data skewness. This imbalance can cause certain tasks to take longer than others, leading to inefficient resource utilization and increased processing time.
Salting: An Overview
Salting is a technique used to mitigate the effects of skewed data. It involves adding a random component, or ‘salt’, to the data to distribute it more evenly across partitions. This results in a more balanced workload and improved performance.
How Does Salting Work in Spark?
Let’s break down the process of salting in Spark:
Identify the skewed key: The first step is to identify the key causing data skewness. This is usually the key with a disproportionately high frequency.
Add a random salt to the skewed key: Once the skewed key is identified, a random salt is added to it. This is done by appending a random number to the key. The salted key helps in distributing the data more evenly across partitions.
Repartition the data: After salting, the data is repartitioned based on the new salted key. This results in a more balanced distribution of data across partitions.
Join the data: Finally, the data is joined based on the salted key. This join operation is more efficient as the data is now evenly distributed.
Here’s a simple code snippet to illustrate the process:
from pyspark.sql import SparkSession
from pyspark.sql import functions as F
from pyspark.sql.types import StringType
# Create a Spark session
spark = SparkSession.builder.appName("skewed_data_example").getOrCreate()
# Create a list with skewed data
skewed_data = ['A'] * 1000 + ['B'] * 100 + ['C'] * 10 + ['D']
# Create a DataFrame with skewed data
data = [(key,) for key in skewed_data]
df = spark.createDataFrame(data, ["key"])
# Show the initial distribution of the 'key' column
print("Initial Distribution:")
df.groupBy("key").count().show()
# Identify the skewed key
skewed_key = df.groupBy('key').count().orderBy('count', ascending=False).first()[0]
# Add a random salt to the skewed key
df = df.withColumn('salted_key', F.when(F.col('key') == skewed_key, F.concat(F.col('key'), F.lit('_'), F.rand())).otherwise(F.col('key')))
# Repartition the data based on the salted key
df = df.repartition('salted_key')
# Show the final distribution after salting
print("Distribution After Salting:")
df.groupBy("salted_key").count().show()
# Stop the Spark session
spark.stop()
Output:
Initial Distribution:
+---+-----+
|key|count|
+---+-----+
| A| 1000|
| B| 100|
| D| 1|
| C| 10|
+---+-----+
Distribution After Salting:
+--------------------+-----+
| salted_key|count|
+--------------------+-----+
|A_0.6085120871038752| 1|
|A_0.5473367783267937| 1|
|A_0.6851350396669373| 1|
|A_0.2110229418229...| 1|
|A_0.3773916484016...| 1|
|A_0.5400136484058142| 1|
|A_0.8891590315157115| 1|
|A_0.4378450723511...| 1|
|A_0.5724962061492399| 1|
|A_0.4490142260480622| 1|
|A_0.5068562849582069| 1|
| A_0.388177896360719| 1|
| A_0.068895976982016| 1|
|A_0.8582788474823554| 1|
|A_0.6914243379635382| 1|
|A_0.3271411577598...| 1|
|A_0.6135472028121775| 1|
|A_0.7981622472957371| 1|
|A_0.0924879519401...| 1|
|A_0.0888750417089...| 1|
+--------------------+-----+
only showing top 20 rows
In this example, we create a DataFrame with a skewed distribution of the ‘key’ column. We then identify the skewed key, add a random salt to it, and finally, repartition the data based on the salted key. The final distribution should demonstrate a more balanced workload across partitions.
In the initial distribution, the ‘key’ column shows that ‘A’ is highly dominant, with 1000 occurrences, followed by ‘B’ with 100 occurrences, ‘C’ with 10 occurrences, and ‘D’ with only 1 occurrence. This confirms a skewed dataset, with ‘A’ contributing significantly to the imbalance.
After applying the salting technique, the ‘salted_key’ column represents the modified keys. Each salted key has a count of 1, indicating that the original data has been partitioned and redistributed with the addition of random salts. This process aims to achieve a more balanced workload across partitions, addressing the initial data skewness issue.
The presence of random numbers in the salted keys ensures a diverse distribution of data, mitigating the impact of skewed data on the performance of Spark jobs.
Benefits of Salting
Salting offers several benefits:
Improved performance: By ensuring a more balanced distribution of data, salting can significantly improve the performance of Spark jobs.
Better resource utilization: Salting helps in better utilization of resources by preventing certain tasks from becoming bottlenecks.
Scalability: Salting allows Spark to handle larger datasets by mitigating the effects of data skewness.
Conclusion
Data skewness is a common challenge in big data processing. Salting is a powerful technique in Spark that helps in dealing with skewed data by ensuring a more balanced distribution of data across partitions. By understanding and effectively using salting, data scientists can improve the performance and scalability of their Spark jobs.
Remember, while salting can be highly beneficial, it’s not a silver bullet for all data skewness problems. It’s important to understand your data and use salting judiciously to get the best results.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.