Solving the TypeError: Unhashable Type: 'numpy.ndarray' in Python
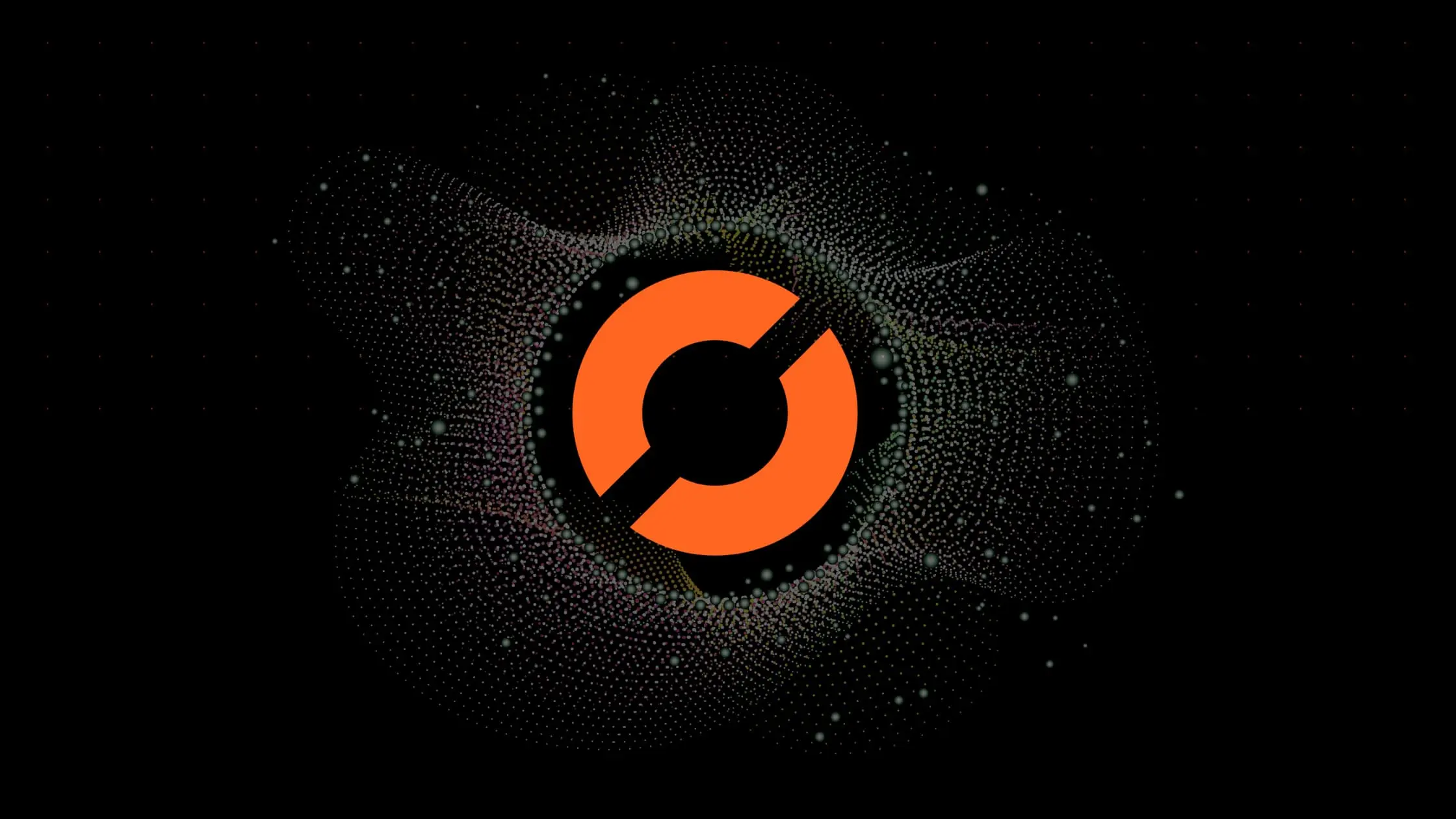
Python is a versatile language widely used in data science. However, it’s not uncommon to encounter errors that can be a bit puzzling. One such error is TypeError: unhashable type: 'numpy.ndarray'
. This blog post will guide you through understanding and solving this error.
Table of Contents
Understanding the Error
Before we dive into the solution, let’s understand the error. The TypeError: unhashable type: 'numpy.ndarray'
typically occurs when you try to use a mutable object, like a numpy array, as a dictionary key or a set member.
In Python, only immutable objects can be hashed, which means they can be used as keys in a dictionary or elements in a set. Mutable objects, on the other hand, cannot be hashed because their content can change over time.
Numpy arrays are mutable, and hence, they are unhashable.
Another reason is when you are trying to convert a 2D numpy array to a set. For a one-dimensional NumPy array, it works well since individual numbers are hashable. However, when dealing with a two-dimensional array, this error occurs due to the fact that the nested arrays within it are not inherently hashable.
The Scenarios and The Solutions
Using an array as a dictionary’s key
Let’s consider a scenario where you might encounter this error. Suppose you have a numpy array and you’re trying to use it as a key in a dictionary:
import numpy as np
my_array = np.array([1, 2, 3])
my_dict = {my_array: 'value'}
Running this code will result in TypeError: unhashable type: 'numpy.ndarray'
.
The Solution
There are several ways to solve this error, depending on your specific use case.
1. Convert the Numpy Array to a Tuple
One common solution is to convert the numpy array to a tuple, which is an immutable object:
my_array = tuple(my_array)
my_dict = {my_array: 'value'}
This code will run without errors because tuples are hashable.
2. Convert the Numpy Array to a String
Another solution is to convert the numpy array to a string:
my_array = str(my_array)
my_dict = {my_array: 'value'}
This code will also run without errors because strings are hashable.
3. Use the Numpy Array as a Value, Not a Key
If you don’t need to use the numpy array as a key, you can use it as a value in the dictionary:
my_dict = {'key': my_array}
This code will run without errors because numpy arrays can be used as values in a dictionary.
Converting 2D Array to a set
import numpy as np
array_2d = np.array([[1,2,3], [1,2,3], [1,2,3]])
set(array_2d)
The Solution
Use map
to map the array to sets and use set.union
import numpy as np
array_2d = np.array([[1,2,3], [1,2,3], [1,2,3]])
out_set = set.union(*map(set,array_2d))
print(out_set)
Output:
{1, 2, 3}
Conclusion
The TypeError: unhashable type: 'numpy.ndarray'
error is a common issue that data scientists encounter when working with Python and numpy. Understanding the difference between mutable and immutable objects, and how they can be used in dictionaries and sets, is key to solving this error.
Remember, if you need to use a numpy array as a key in a dictionary or a set, you’ll need to convert it to an immutable object, like a tuple or a string. Alternatively, you can use the numpy array as a value in the dictionary.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.