Solving the TypeError: 'Column' Object is Not Callable in PySpark Text Lemmatization
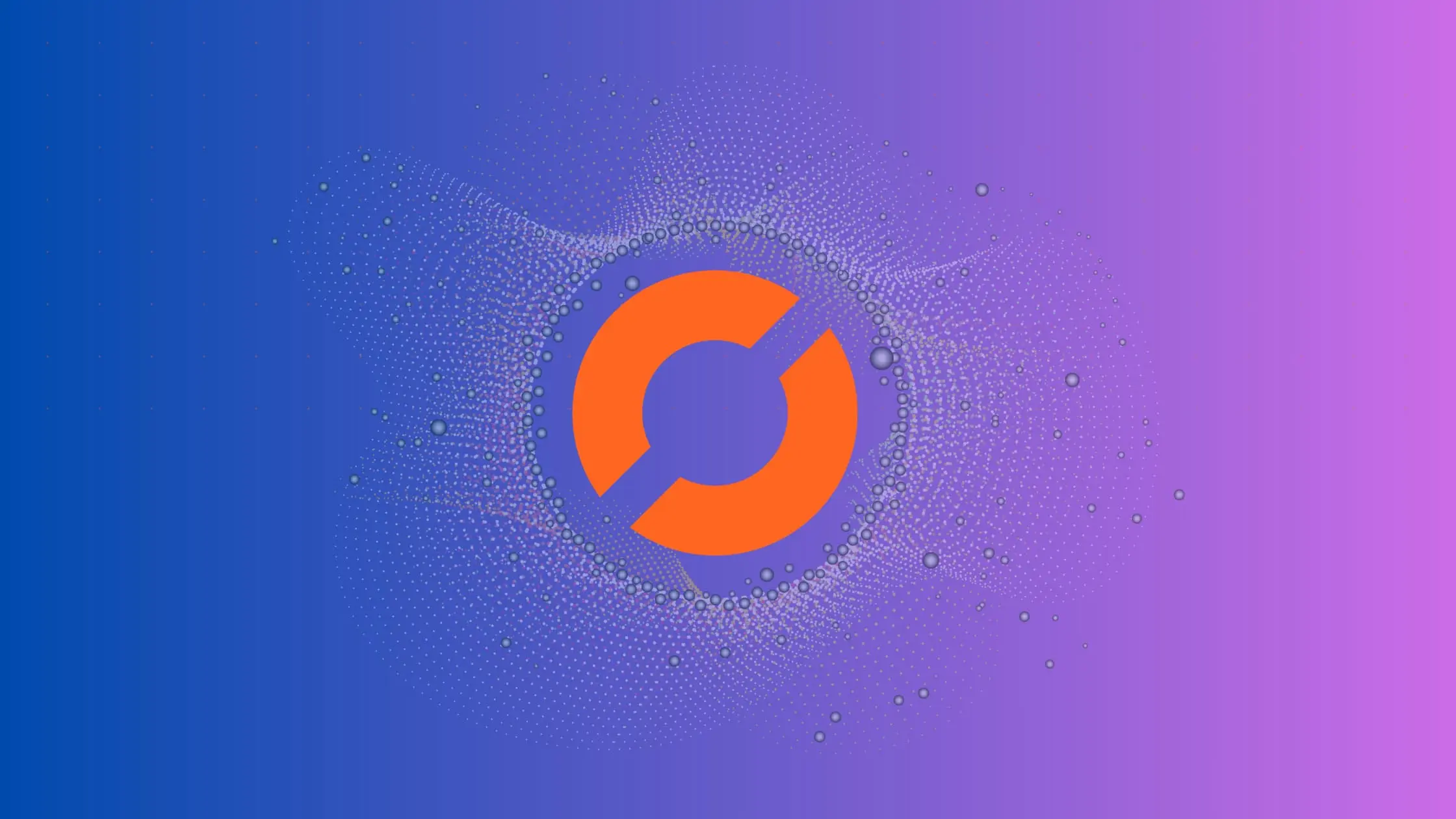
PySpark is a powerful tool for handling big data, but it can sometimes throw errors that are difficult to debug. One such error is the TypeError: 'Column' object is not callable
that you might encounter while performing text lemmatization. This blog post will guide you through the process of resolving this issue.
Understanding the Problem
Before we dive into the solution, let’s understand the problem. Text lemmatization is a common preprocessing step in Natural Language Processing (NLP). It involves reducing words to their base or dictionary form, known as a lemma. For example, the words “running”, “runs”, and “ran” would all be reduced to “run”.
In PySpark, you might try to perform lemmatization using a User Defined Function (UDF) that applies a lemmatization function from the NLTK library to a column in a DataFrame. However, this can result in a TypeError: 'Column' object is not callable
error. This error occurs because PySpark tries to apply the function to the column object itself, rather than to the values within the column.
from pyspark.sql import SparkSession
from pyspark.sql.functions import col, udf
from nltk.stem import WordNetLemmatizer
# Create a Spark session
spark = SparkSession.builder.appName("LemmatizationExample").getOrCreate()
# Sample DataFrame
data = [("I love programming",), ("Python is amazing",), ("Spark is powerful",)]
columns = ["text_data"]
df = spark.createDataFrame(data, columns)
# Define a lemmatization function using nltk
def lemmatize_text(text):
lemmatizer = WordNetLemmatizer()
return ' '.join([lemmatizer.lemmatize(word) for word in text.split()])
# Apply lemmatization to the 'text_data' column
df = df.withColumn("lemmatized_text", lemmatize_text(col("text_data")))
In this code, we first import the necessary modules and initialize the WordNetLemmatizer
. We then define a function lemmatize_word
that takes a word as input and returns its lemma. This code will result in the TypeError: 'Column' object is not callable
error because the lemmatize_text function is being applied directly to the col("text_data")
column.
The Solution
To resolve this issue, we need to use the udf
(user-defined function) functionality in PySpark. This allows us to apply a custom function to a DataFrame column element-wise. Here’s how you can modify the code to avoid the error:
from pyspark.sql.types import StringType
# Define a lemmatization function using nltk and wrap it with a udf
lemmatize_udf = udf(lambda text: lemmatize_text(text), StringType())
# Apply lemmatization using the udf
df = df.withColumn("lemmatized_text", lemmatize_udf(col("text_data")))
Best Practices
While the above solution works, there are a few best practices you should follow when working with PySpark and NLTK:
Broadcasting: NLTK data like the WordNet corpus can be quite large. To avoid sending this data over the network multiple times, you can use PySpark’s broadcasting feature to send it once and have it available on all nodes.
Vectorized UDFs: PySpark 2.3 introduced vectorized UDFs, which can significantly improve performance by leveraging Apache Arrow to avoid serialization and deserialization between Python and JVM.
Error Handling: Always include error handling in your UDFs. This can help you catch and debug any issues that arise during the lemmatization process.
Conclusion
PySpark is a powerful tool for big data processing, but it can sometimes throw confusing errors. The TypeError: 'Column' object is not callable
error in text lemmatization is one such example. By understanding how PySpark applies functions to DataFrame columns, you can easily resolve this issue and continue with your text preprocessing.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.