Solving the AttributeError: 'DataFrame' Object Has No Attribute 'concat' in Pandas
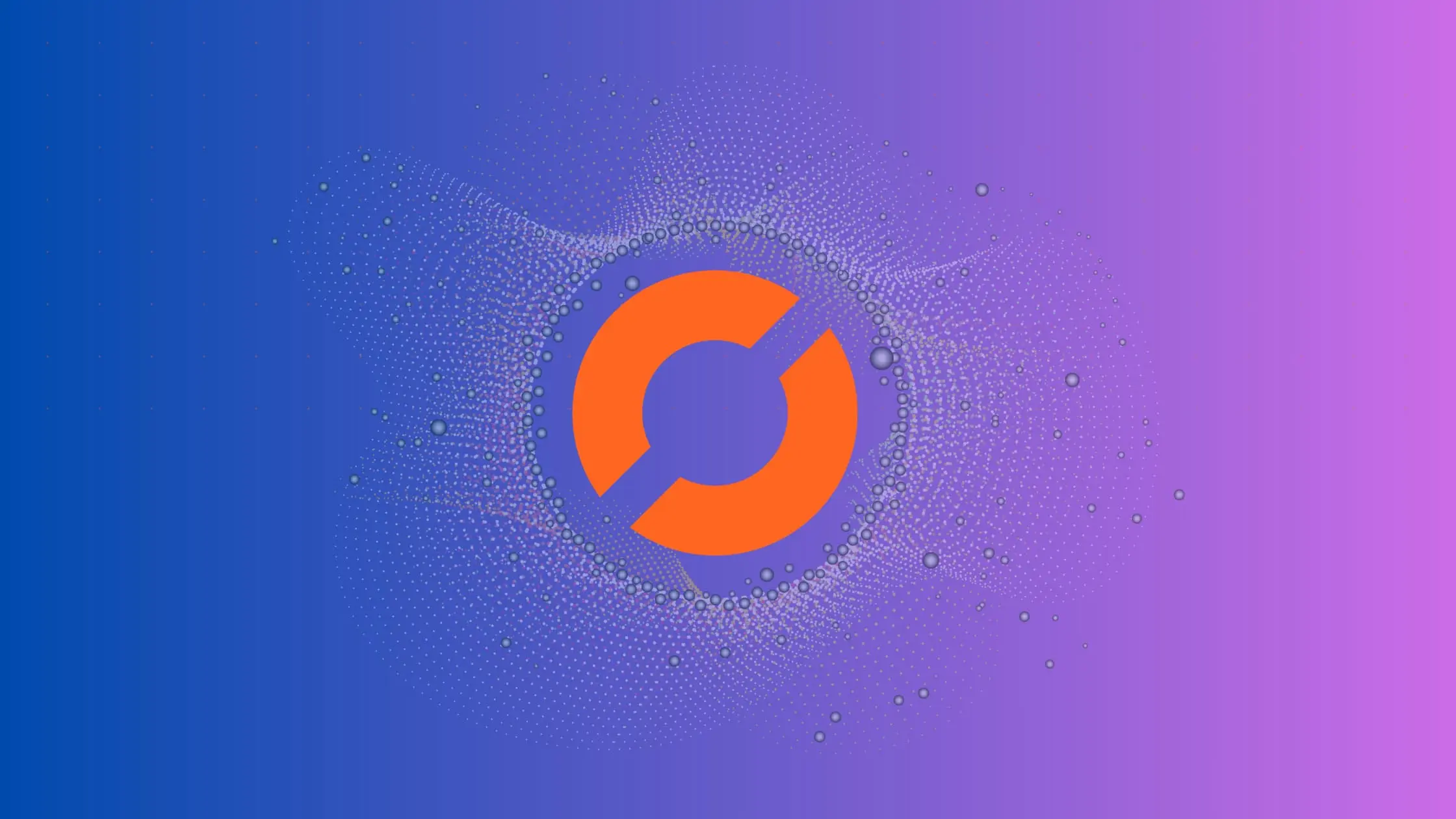
When working with data in Python, Pandas is an indispensable tool. It provides powerful data structures to perform data manipulation and analysis. However, it’s not uncommon to encounter errors when using Pandas, especially when trying to concatenate two columns. One such error is the AttributeError: 'DataFrame' object has no attribute 'concat'
. In this blog post, we’ll explore how to solve this issue and correctly concatenate two columns in a Pandas DataFrame.
Understanding the Error
Before we dive into the solution, let’s understand the error. The AttributeError: 'DataFrame' object has no attribute 'concat'
typically occurs when you try to use the concat
method directly on a DataFrame object. This is a common mistake because concat
is not a DataFrame method, but a Pandas function.
The common error when using concat
as a DataFrame method
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'A': ['Hello', 'Hi'],
'B': ['World', 'Everyone']
})
# Concatenate columns the wrong way
df['C'] = df.concat([df['A'], df['B']], axis=1)
print(df)
If you run the code above, it will throw an error: AttributeError: 'DataFrame' object has no attribute 'concat'
The Correct Way to Concatenate Columns in Pandas
To concatenate two columns in a Pandas DataFrame, you should use the +
operator or the str.cat()
method, not the concat
function. Here’s how you can do it:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'A': ['Hello', 'Hi'],
'B': ['World', 'Everyone']
})
# Concatenate columns using the + operator
df['C'] = df['A'] + ' ' + df['B']
print(df)
This will output:
A B C
0 Hello World Hello World
1 Hi Everyone Hi Everyone
If you want to use the str.cat()
method, you can do it like this:
# Concatenate columns using the str.cat() method
df['C'] = df['A'].str.cat(df['B'], sep=' ')
print(df)
This will output the same result.
When to Use the concat
Function
The concat
function is used to concatenate two or more Pandas objects along a particular axis. It’s typically used to concatenate two DataFrames or Series, not individual columns within a DataFrame. Here’s an example:
# Create two DataFrames
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']})
# Concatenate the DataFrames
result = pd.concat([df1, df2])
print(result)
This will output:
A B
0 A0 B0
1 A1 B1
0 A2 B2
1 A3 B3
Conclusion
In conclusion, the AttributeError: 'DataFrame' object has no attribute 'concat'
error occurs when you try to use the concat
function as a DataFrame method. To concatenate two columns in a Pandas DataFrame, you should use the +
operator or the str.cat()
method. The concat
function should be used to concatenate two or more Pandas objects along a particular axis.
Understanding the tools you’re working with is crucial in data science. It not only helps you avoid errors but also allows you to use these tools more effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.