Shift Data and Create New Column in Python DataFrames: A Guide
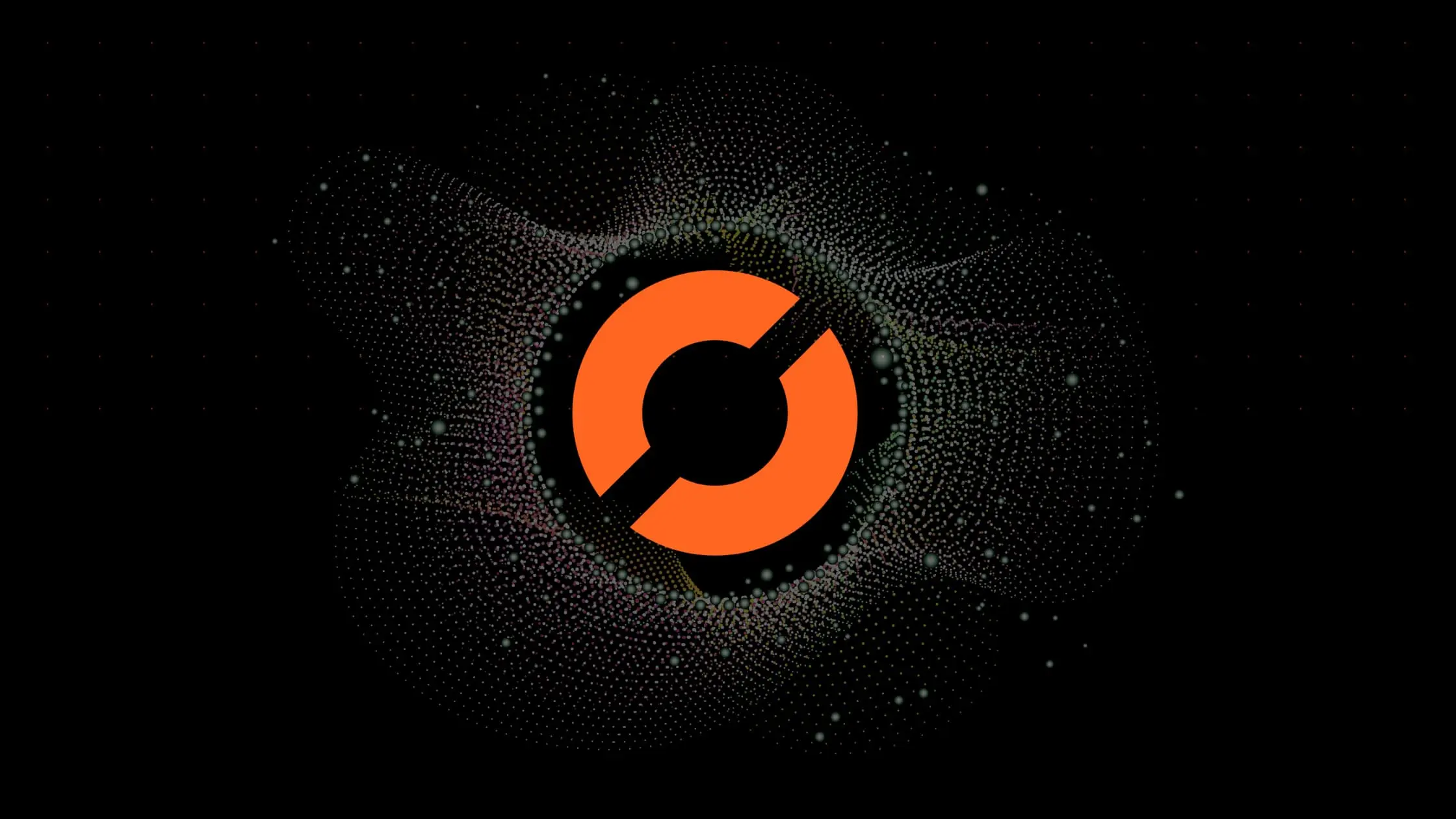
Data manipulation is a crucial part of data science. One common operation is shifting data and creating new columns in Python DataFrames. This guide will walk you through the process, using the powerful pandas library.
Table of Contents
- Introduction
- Shifting Data in Python DataFrames
- Creating New Columns in Python DataFrames
- Combining Shifting and Creating New Columns
- Pros and Cons
- Common Errors
- Conclusion
Introduction
Pandas is a popular Python library for data manipulation and analysis. It provides flexible and efficient data structures, including the DataFrame, which is a two-dimensional labeled data structure with columns of potentially different types.
In this guide, we’ll focus on shifting data and creating new columns in Python DataFrames. This operation is often used in time series analysis, machine learning feature engineering, and other data science tasks.
Shifting Data in Python DataFrames
Shifting data means moving data along the index. In pandas, the shift()
function is used for this purpose. Let’s see how it works.
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3, 4, 5]})
# Shift data
df['B'] = df['A'].shift(1)
print(df)
In this example, we shift the 'A'
column by one position. The output will be:
A B
0 1 NaN
1 2 1.0
2 3 2.0
3 4 3.0
4 5 4.0
The shift()
function moves data down by the specified number of periods (1 by default). A positive value shifts data down, while a negative value shifts data up.
Creating New Columns in Python DataFrames
Creating new columns in pandas is straightforward. You can create a new column by assigning a value or a series of values to a new column name. Here’s an example:
# Create a new column 'C' with a constant value
df['C'] = 10
# Create a new column 'D' with a series of values
df['D'] = pd.Series([6, 7, 8, 9, 10])
print(df)
The output will be:
A B C D
0 1 NaN 10 6
1 2 1.0 10 7
2 3 2.0 10 8
3 4 3.0 10 9
4 5 4.0 10 10
Combining Shifting and Creating New Columns
Now, let’s combine these two operations. Suppose we want to create a new column ‘E’ that contains the difference between the current and previous values in column ‘A’. Here’s how to do it:
# Create a new column 'E' with the difference between the current and previous values in 'A'
df['E'] = df['A'] - df['A'].shift(1)
print(df)
The output will be:
A B C D E
0 1 NaN 10 6 NaN
1 2 1.0 10 7 1.0
2 3 2.0 10 8 1.0
3 4 3.0 10 9 1.0
4 5 4.0 10 10 1.0
Pros and Cons
Pros:
- Time-Series Analysis: Ideal for time-series data to create lag features.
- Sequential Data Processing: Useful when dealing with sequences and dependencies.
Cons:
- Data Loss: Shifting may result in missing values at the beginning or end of the DataFrame.
- Memory Usage: Creating a new column increases memory usage.
Common Errors
Handling Missing Values
When shifting data, be aware that the first few rows will have missing values. Ensure proper handling to avoid issues in downstream analysis or modeling.
Incorrect Usage of Shift
Incorrectly applying the shift operation might lead to unexpected results. Always specify the correct column and number of periods.
Conclusion
Shifting data in Python DataFrames is a powerful technique for creating new columns based on existing values. While it comes with advantages such as facilitating time-series analysis, it’s crucial to be aware of potential pitfalls like data loss and increased memory usage. By understanding the shift operation, handling common errors, and exploring practical examples, you can enhance your data manipulation skills and make informed decisions when working with sequential data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.