Reshaping 3D Numpy Arrays to 2D: A Guide for Data Scientists
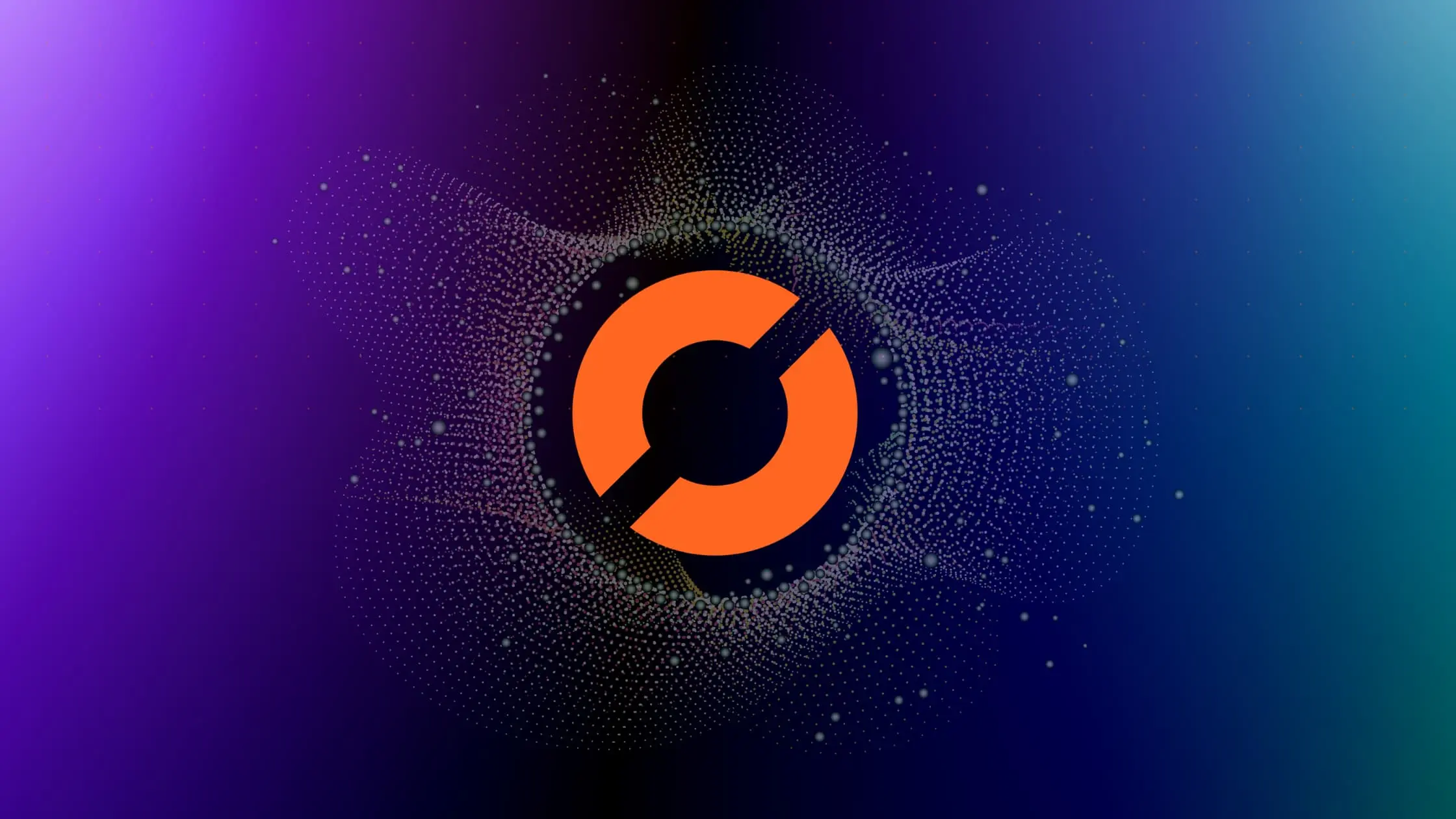
Numpy, a fundamental package for scientific computing in Python, is a powerful tool for data manipulation. One of its key features is the ability to reshape arrays. In this blog post, we’ll delve into the process of reshaping a 3D Numpy array into a 2D array, a common requirement in data science projects.
Table of Contents
- Why Reshape Arrays?
- Understanding Numpy Arrays
- Creating a 3D Numpy Array
- Reshaping a 3D Array to a 2D Array
- Understanding the Reshaped Array
- Pros and Cons of Reshaping 3D Numpy Arrays to 2D
- Common Errors and Solutions
- Conclusion
Why Reshape Arrays?
Reshaping arrays is a common operation in data science, particularly when preparing data for machine learning algorithms. It allows us to transform our data into the required format, making it compatible with various libraries and functions.
Understanding Numpy Arrays
Before we dive into reshaping, let’s briefly discuss Numpy arrays. A Numpy array is a grid of values, all of the same type, and is indexed by a tuple of non-negative integers. The dimensions are defined by its shape, a tuple of integers that give the size of the array along each dimension.
Creating a 3D Numpy Array
Let’s start by creating a 3D array. We can do this using the numpy.array()
function. Here’s an example:
import numpy as np
# Create a 3D array
array_3d = np.array([[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]])
print(array_3d)
This will create a 3D array with shape (2, 2, 3)
.
[[[ 1 2 3]
[ 4 5 6]]
[[ 7 8 9]
[10 11 12]]]
Reshaping a 3D Array to a 2D Array
Now, let’s reshape our 3D array to a 2D array. We can use the numpy.reshape()
function for this. The reshape()
function allows us to reorganize the array elements, given new dimensions.
Here’s how to do it:
# Reshape the 3D array to a 2D array
array_2d = array_3d.reshape(-1, array_3d.shape[-1])
print(array_2d)
Output:
[[ 1 2 3]
[ 4 5 6]
[ 7 8 9]
[10 11 12]]
In this example, -1
is used as a placeholder for the size of the first dimension, allowing Numpy to automatically calculate the appropriate size based on the total number of elements.. array_3d.shape[-1]
means we are keeping the size of the last dimension the same.
Understanding the Reshaped Array
The reshaped 2D array has a shape of (4, 3)
. The first dimension is calculated by multiplying the first two dimensions of the 3D array (2*2=4)
, and the second dimension is the same as the last dimension of the 3D array (3)
.
Pros and Cons of Reshaping 3D Numpy Arrays to 2D
Pros:
Simplified Structure: Reshaping to 2D can simplify the data structure, making it easier to apply certain machine learning algorithms.
Compatibility: Some machine learning libraries or models might expect 2D input, and reshaping helps ensure compatibility.
Cons:
Data Loss: Reshaping can lead to information loss, especially if the original 3D structure is crucial for analysis.
Increased Memory Usage: In some cases, reshaping can lead to increased memory usage, impacting performance.
Common Errors and Solutions
Error 1: ValueError: cannot reshape array
This error occurs when the total number of elements in the original array does not match the total number of elements in the target shape.
Solution: Ensure that the total number of elements in the original array is equal to the total number of elements in the desired shape.
# Example
arr_3d = np.random.rand(2, 3, 4)
reshaped_2d = arr_3d.reshape(-1, 5) # Incorrect target shape
# Corrected target shape
reshaped_2d_corrected = arr_3d.reshape(-1, arr_3d.shape[-1])
Error 2: ValueError: cannot reshape array of size x into shape (a, b, c)
This error occurs when the total number of elements in the original array is not divisible by the total number of elements in the target shape.
Solution: Adjust the target shape to ensure compatibility.
# Example
arr_3d = np.random.rand(2, 3, 4)
reshaped_2d = arr_3d.reshape(2, 2, 3) # Incorrect target shape
# Corrected target shape
reshaped_2d_corrected = arr_3d.reshape(-1, arr_3d.shape[-1])
Conclusion
Reshaping arrays is a fundamental operation in data science and machine learning. It allows us to transform our data into the required format, making it compatible with various libraries and functions. Numpy provides a simple and efficient way to reshape arrays, making it an essential tool for any data scientist.
We hope this guide has been helpful in understanding how to reshape a 3D Numpy array into a 2D array. Stay tuned for more guides on leveraging the power of Numpy for your data science projects!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.