Reading Large Text Files with Pandas
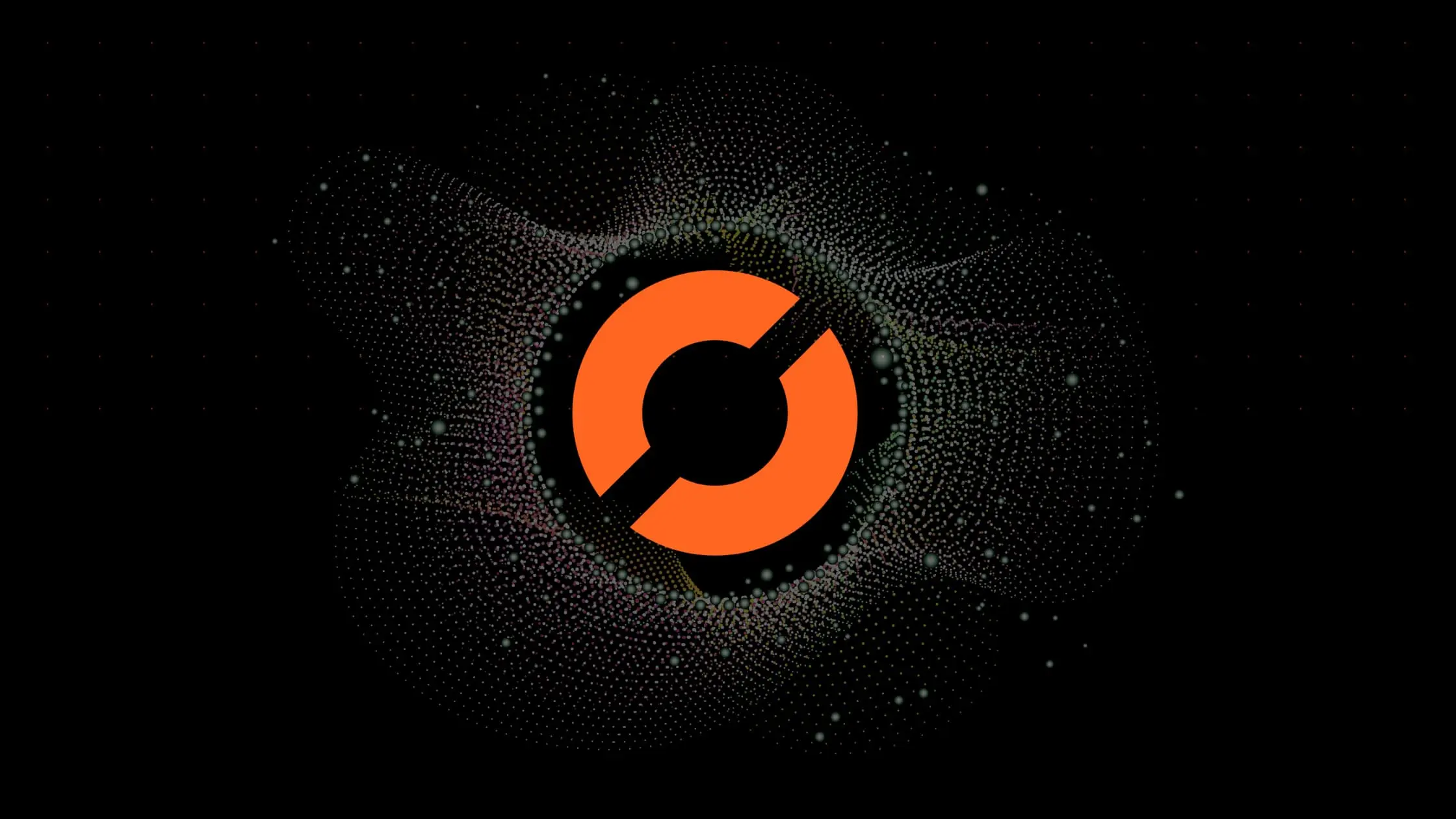
As a data scientist or software engineer, you may often find yourself working with large datasets that are saved in text files. These files can be challenging to read and manipulate, especially when they are too big to be loaded into memory at once. One of the most popular tools for working with data in Python is Pandas, which provides efficient and powerful data structures for data manipulation and analysis. In this article, we will explore how to use Pandas to read large text files efficiently and effectively.
Why Use Pandas to Read Large Text Files?
Pandas is a popular library for data analysis and manipulation in Python. It provides powerful and efficient data structures and functions that make it easy to work with large datasets. Pandas is especially useful when it comes to working with text files because it provides several functions that allow you to read and manipulate text data efficiently.
One of the main advantages of using Pandas to read large text files is that it allows you to load data in chunks. This means that you can read and process data in smaller pieces, rather than trying to load the entire file into memory at once. This is particularly useful when working with very large datasets that would otherwise be too big to fit into your computer’s memory.
Another advantage of using Pandas is that it provides a wide range of tools for data manipulation and analysis. Once you have loaded your data into a Pandas DataFrame, you can use Pandas' built-in functions to perform a wide range of tasks, including filtering, sorting, grouping, and aggregating your data.
How to Read Large Text Files with Pandas
Reading large text files with Pandas is a straightforward process that involves a few simple steps. Let’s take a look at how to do it.
Step 1: Import the Pandas Library
The first step is to import the Pandas library into your Python script. You can do this using the following code:
import pandas as pd
Step 2: Define the File Path
Next, you need to define the path to the text file that you want to read. You can do this using the following code:
file_path = "path/to/your/file.txt"
Replace path/to/your/file.txt
with the actual path to your text file.
Step 3: Define the Chunk Size
Now, you need to define the size of the chunks that you want to read from the file. You can do this using the following code:
chunk_size = 1000000
In this example, we have defined a chunk size of 1,000,000 rows. You can adjust this number depending on the size of your file and the amount of memory that you have available.
Step 4: Define the Column Names (Optional)
If your text file contains column headers, you can define them using the following code:
column_names = ["col1", "col2", "col3"]
Replace "col1", "col2", and "col3"
with the actual column names in your text file.
Step 5: Create a Pandas DataFrame
Now you are ready to create a Pandas DataFrame object that will hold your data. You can do this using the following code:
df_list = []
for chunk in pd.read_csv(file_path, chunksize=chunk_size, names=column_names):
df_list.append(chunk)
df = pd.concat(df_list)
Let’s break down this code:
- We start by creating an empty list called
df_list
. This list will hold each chunk of data that we read from the file. - We then use a for loop to read the file in chunks using the
pd.read_csv()
function. This function reads the file in chunks of the size that we defined earlier (chunk_size
) and returns a Pandas DataFrame object. - Each chunk of data is appended to the
df_list
. - Finally, we use the
pd.concat()
function to concatenate all of the data chunks into a single Pandas DataFrame object calleddf
.
Step 6: Manipulate and Analyze Your Data
Now that you have loaded your data into a Pandas DataFrame, you can use Pandas' built-in functions to manipulate and analyze your data. For example, you can filter your data using the df.loc[]
function, sort your data using the df.sort_values()
function, and group your data using the df.groupby()
function.
Common Errors and Troubleshooting
MemoryError: Handling Memory-Related Errors: When dealing with large files, a MemoryError may occur. To mitigate this, consider using the chunksize parameter to read the file in smaller portions and reducing it arcordingly to overcome the memory issue.
chunk_size = 10000 # reduce it arcordingly to the memory specs chunks = pd.read_csv(filepath, chunksize=chunk_size) # Process chunks for chunk in chunks: process(chunk)
DtypeWarning: Addressing Data Type Inference Issues: To handle DtypeWarning issues related to data type inference, explicitly specify the data types when reading the file:
df = pd.read_csv('large_file.csv', dtype={'column_name': 'desired_dtype'})
UnicodeDecodeError: Dealing with Character Encoding Problems: If encountering UnicodeDecodeError, explicitly specify the encoding of the file:
df = pd.read_csv('large_file.csv', encoding='utf-8')
Conclusion
Reading large text files with Pandas is a simple and efficient process that can save you time and memory when working with large datasets. By using Pandas' built-in functions to read and manipulate your data, you can quickly analyze and visualize your data and gain insights that would otherwise be difficult to obtain. With the steps outlined in this article, you should be well-equipped to start working with large text files in Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.