Python Pandas ValueError Arrays Must be All Same Length
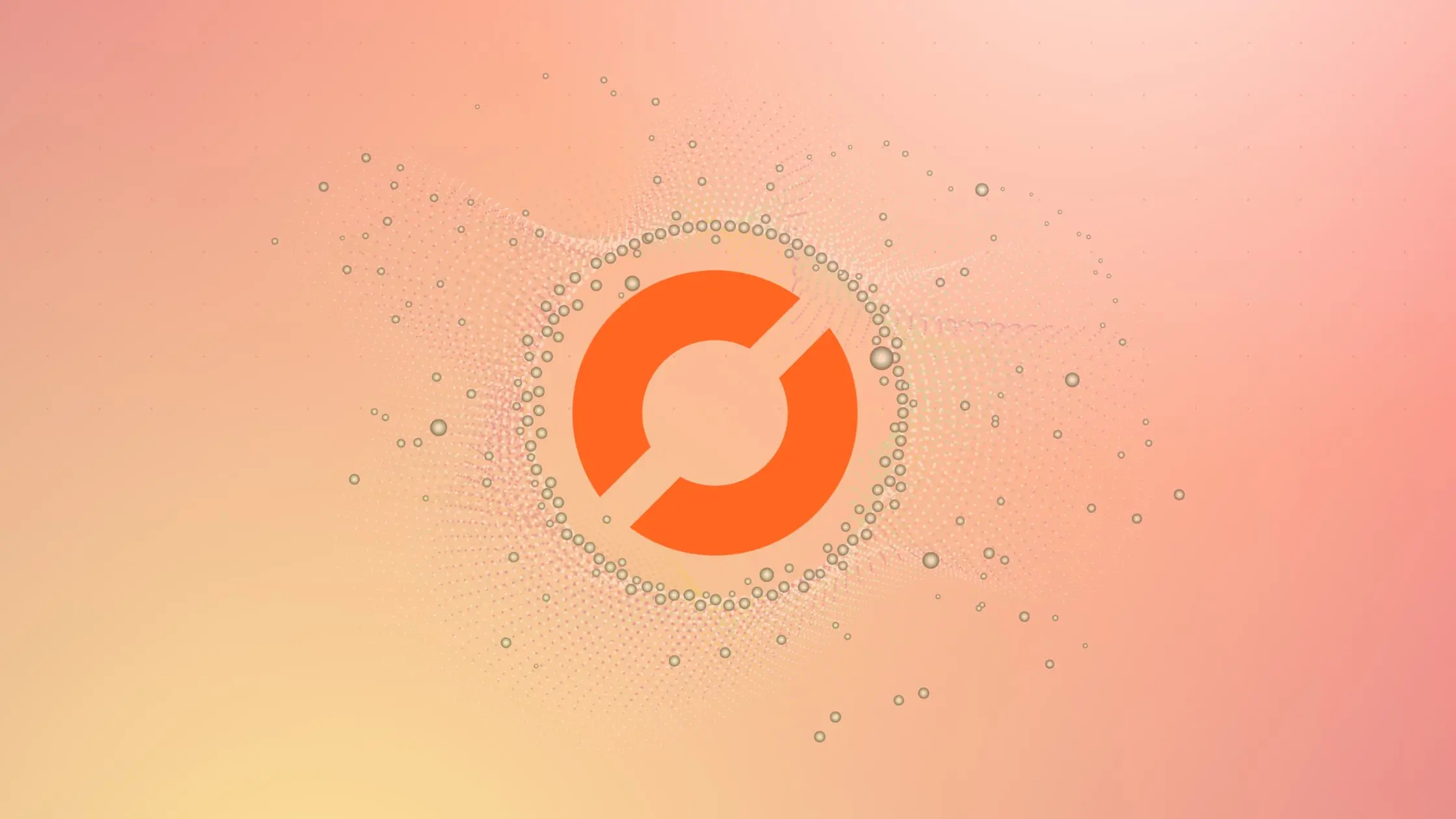
If you are a data scientist or software engineer working with Python and Pandas, you may have encountered the ValueError: Arrays must be all same length error. This error occurs when you try to concatenate two or more arrays of different lengths using the Pandas concat() function. In this blog post, we will explore what this error means, why it occurs, and how to fix it.
Table of Contents
- What is Pandas?
- What is the ValueError: Arrays must be all same length error?
- How to fix the ValueError: Arrays must be all same length error
- Best Practices
- Conclusion
What is Pandas?
Pandas is a popular library for data manipulation and analysis in Python. It provides data structures and functions for working with structured data, including tables, time series, and numerical arrays. Pandas is built on top of NumPy, another popular numerical library in Python.
What is the ValueError: Arrays must be all same length error?
The ValueError: Arrays must be all same length error occurs when you try to concatenate two or more arrays of different lengths using the Pandas concat() function. For example, consider the following code:
import pandas as pd
# Error: Unequal length lists
array1 = [1, 2, 3, 4]
array2 = ['a', 'b', 'c', 'd', 'e']
# Attempting to create a DataFrame
df = pd.DataFrame({'Column1': array1, 'Column2': array2})
# ValueError: Arrays Must be All Same Length
The goal is to create a Pandas DataFrame named df
using the pd.DataFrame()
constructor. Two arrays, array1
and array2
, are provided as data for the DataFrame. The keys of the dictionary passed to pd.DataFrame()
are intended to be column names.
However, the code encounters a ValueError
because the lengths of array1
and array2
are not the same. The array1
has four elements, while array2
has five elements. In Pandas, when creating a DataFrame from a dictionary, all the arrays (values in the dictionary) must be of the same length.
How to fix the ValueError: Arrays must be all same length error
There are several ways to fix the ValueError: Arrays must be all same length error, depending on your use case. Here are some common solutions:
Method 1: Explicit Length Check
One of the straightforward ways to handle this error is by explicitly checking the length of your arrays before creating the DataFrame. This method ensures that all arrays have the same length, preventing the ValueError.
import pandas as pd
# Example arrays
array1 = [1, 2, 3, 4]
array2 = ['a', 'b', 'c']
# Explicit length check
if len(array1) == len(array2):
df = pd.DataFrame({'Column1': array1, 'Column2': array2})
else:
print("Arrays must be of the same length.")
Method 2: Zip Method
Using the zip
function is another elegant way to synchronize multiple arrays and create a DataFrame. It automatically stops pairing when the shortest array ends.
import pandas as pd
# Example arrays
array1 = [1, 2, 3, 4]
array2 = ['a', 'b', 'c']
# Creating DataFrame using zip
df = pd.DataFrame(list(zip(array1, array2)), columns=['Column1', 'Column2'])
Method 3: DataFrame from Dictionary
Constructing a DataFrame from a dictionary is a concise approach, ensuring keys and values have the same length.
import pandas as pd
# Example arrays
array1 = [1, 2, 3, 4]
array2 = ['a', 'b', 'c']
# Creating DataFrame from a dictionary
df = pd.DataFrame({'Column1': array1, 'Column2': array2})
Best Practices
- Consistent Data Collection: Ensure that data is collected and structured consistently to avoid length discrepancies.
- Use Explicit Checks: Implement explicit length checks before creating DataFrames to catch potential errors early.
- Utilize Zip for Simplicity: The
zip
function is a concise way to synchronize arrays when their lengths may vary.
Conclusion
The ValueError: Arrays must be all same length error is a common error in Pandas when trying to concatenate arrays of different lengths. In this blog post, we explained what this error means, why it occurs, and how to fix it. We hope this post helps you avoid this error in your future Pandas projects. Remember to always check the length of your arrays and use the appropriate function for your use case.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.