Python Pandas Json to DataFrame
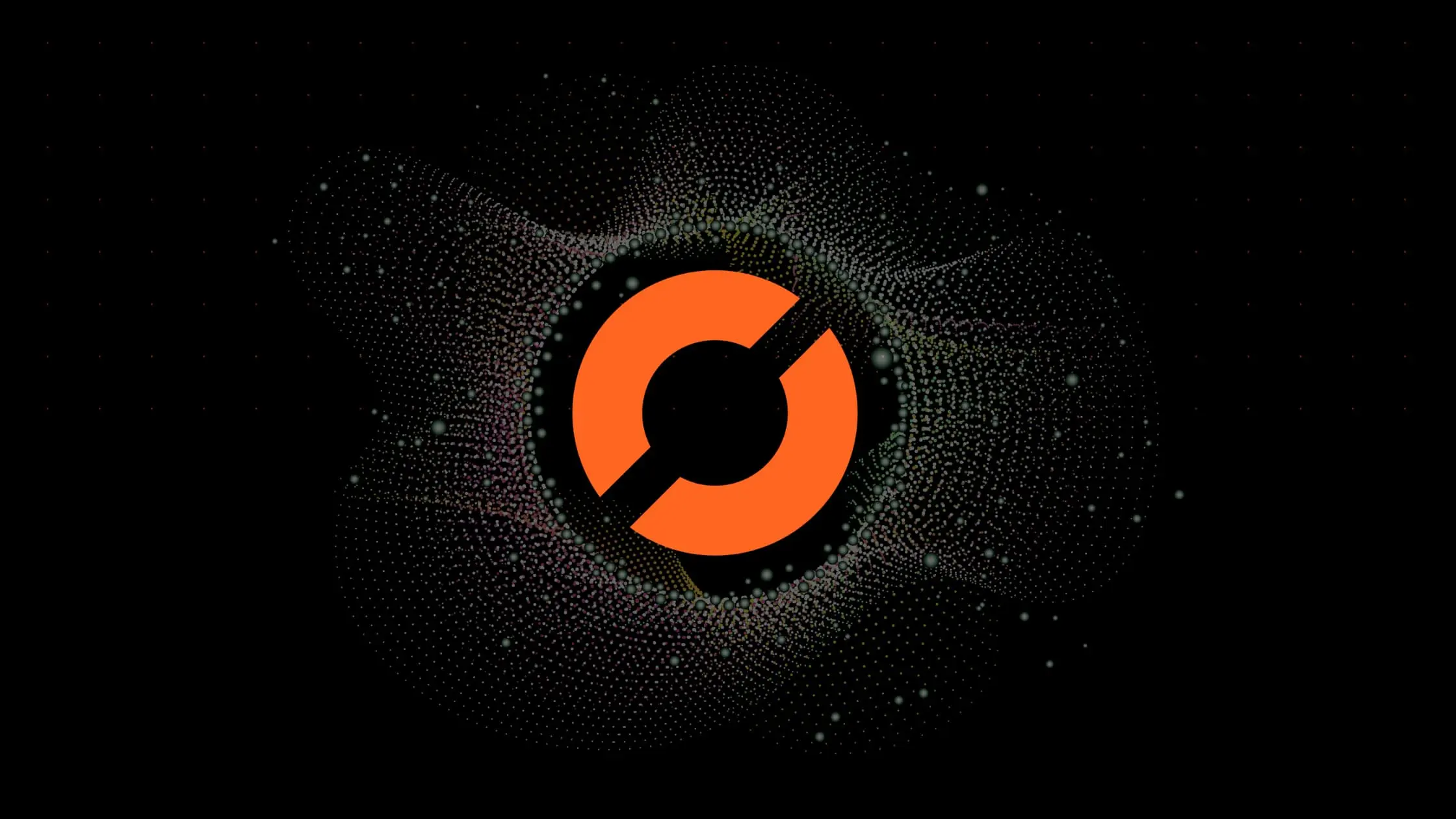
As a data scientist or software engineer working with data, you might come across situations where you need to convert JSON data to a Pandas DataFrame. In this article, we will discuss the process of converting JSON data to a Pandas DataFrame using Python’s Pandas library.
Table of Contents
- Introduction
- What is JSON?
- What is Pandas?
- Converting JSON to Pandas DataFrame
- Handling Nested JSON Data
- Flattening Nested JSON Data in Pandas
- Pros and Cons of Converting JSON to Pandas
- Error Handling
- Conclusion
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data interchange format. It is easy for humans to read and write and easy for machines to parse and generate. JSON is a text format that is completely language-independent, making it an ideal choice for data exchange between systems.
JSON data is represented as key-value pairs, where the key is a string and the value can be of any data type. JSON supports basic data types such as strings, numbers, and booleans, as well as complex data types such as arrays and objects.
What is Pandas?
Pandas is a Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for data cleaning, filtering, and transformation. Pandas is built on top of the NumPy library and is designed to work seamlessly with other Python libraries.
Converting JSON to Pandas DataFrame
Pandas provides a convenient function called read_json()
that can be used to convert JSON data to a Pandas DataFrame. The read_json()
function can read JSON data from a file or a URL and return a DataFrame object.
Here is an example of how to use the read_json()
function to convert JSON data to a Pandas DataFrame:
import pandas as pd
import json
# JSON data
json_data = {
"name": ["John", "Jane", "Bob"],
"age": [25, 30, 35],
"city": ["New York", "San Francisco", "Chicago"]
}
# Convert dictionary to JSON string
json_string = json.dumps(json_data)
# Convert JSON string to Pandas DataFrame
df = pd.read_json(json_string)
# Print DataFrame
print(df)
Output:
name age city
0 John 25 New York
1 Jane 30 San Francisco
2 Bob 35 Chicago
In the above example, we define a JSON object json_data
that contains three key-value pairs representing the name, age, and city of three individuals. We then pass this JSON object to the read_json()
function, which reads the JSON data and returns a Pandas DataFrame object.
Handling Nested JSON Data
JSON data can also contain nested objects and arrays. In such cases, the read_json()
function can be used with some additional parameters to handle the nested data.
Here is an example of how to use the read_json()
function to handle nested JSON data:
import pandas as pd
import json
# Nested JSON data
json_data = {
"name": ["John", "Jane", "Bob"],
"age": [25, 30, 35],
"city": ["New York", "San Francisco", "Chicago"],
"children": [
{"child_name": "Mary", "child_age": 5},
{"child_name": "Tom", "child_age": 3},
{"child_name": "Kate", "child_age": 2}
]
}
# Convert dictionary to JSON string
json_string = json.dumps(json_data)
# Convert nested JSON data to Pandas DataFrame
df = pd.read_json(json_string, orient='columns')
# Print DataFrame
print(df)
Output:
name age city children
0 John 25 New York {'child_name': 'Mary', 'child_age': 5}
1 Jane 30 San Francisco {'child_name': 'Tom', 'child_age': 3}
2 Bob 35 Chicago {'child_name': 'Kate', 'child_age': 2}
In the above example, we define a JSON object json_data
that contains a nested object children
, which itself contains three key-value pairs representing the name and age of three children. We pass this JSON object to the read_json()
function, which reads the JSON data and returns a Pandas DataFrame object.
Flattening Nested JSON Data in Pandas
In data analysis and manipulation, you often encounter nested JSON structures that need to be flattened for better representation in a tabular format. Pandas provides a convenient way to achieve this using the json_normalize
function. Let’s explore how to flatten nested JSON data with a practical example.
Consider the following nested JSON data:
import pandas as pd
import json
from pandas import json_normalize
# Nested JSON data
json_data = {
"name": ["John", "Jane", "Bob"],
"age": [25, 30, 35],
"city": ["New York", "San Francisco", "Chicago"],
"children": [
{"child_name": "Mary", "child_age": 5},
{"child_name": "Tom", "child_age": 3},
{"child_name": "Kate", "child_age": 2}
]
}
# Convert dictionary to JSON string
json_string = json.dumps(json_data)
# Convert nested JSON data to Pandas DataFrame
df = pd.read_json(json_string, orient='columns')
# Normalize the 'children' column
children_df = json_normalize(df['children'])
# Concatenate the normalized 'children' DataFrame with the original DataFrame
df = pd.concat([df, children_df], axis=1)
# Drop the original 'children' column
df = df.drop('children', axis=1)
# Print DataFrame
print(df)
Output:
name age city child_name child_age
0 John 25 New York Mary 5
1 Jane 30 San Francisco Tom 3
2 Bob 35 Chicago Kate 2
In this example, we use the json_normalize
function to flatten the ‘children’ column, which contains nested dictionaries. This creates new columns for each nested key, resulting in a more structured and tabular representation of the data.
Pros and Cons of Converting JSON to Pandas
Pros
Ease of Use: The
read_json()
function in Pandas simplifies the process of converting JSON data to a Pandas DataFrame, requiring just a single line of code.Versatility: The function can read JSON data from both files and URLs, providing flexibility in data source selection.
Integration with Python Ecosystem: Pandas seamlessly integrates with other popular Python libraries like NumPy, making it a powerful tool for data manipulation and analysis.
Handling Nested Data: The
read_json()
function includes parameters to handle nested JSON structures, allowing users to efficiently work with complex data formats.Flattening Nested Data: The
json_normalize
function in Pandas provides an elegant solution for flattening nested JSON structures, making the data more suitable for tabular representation and analysis.
Cons
Limited JSON Schema Support: While Pandas handles basic JSON structures well, it might face challenges with complex JSON schemas or those with irregularities.
Dependency on External Libraries: The reliance on the
json_normalize
function for handling nested structures may introduce dependencies on external libraries beyond Pandas.Data Size Consideration: For extremely large JSON datasets, the entire dataset is loaded into memory, potentially leading to performance issues or memory constraints.
Error Handling Complexity: When dealing with diverse JSON datasets, error handling can become complex, especially in cases where the data format varies or contains inconsistencies.
Error Handling:
Invalid JSON Format: Ensure the JSON data is in a valid format; otherwise, the
read_json()
function may raise aValueError
. Validate the JSON structure before attempting to convert it.Handling Missing or Mismatched Keys: Implement checks to handle situations where keys or key-value pairs expected by the DataFrame are missing or don’t match the provided JSON data.
Memory Error Handling: Consider implementing measures to handle memory errors, especially when working with large datasets. This may involve processing the data in chunks or optimizing memory usage.
Network Errors for URL Sources: Address potential network errors when reading JSON from URLs. Implement mechanisms to handle connection issues or timeouts.
Handle NaN Values: When flattening nested data, be aware of potential NaN values that might arise from missing or inconsistent data in nested structures. Implement strategies for handling these NaN values based on the specific use case.
Conclusion
Converting JSON data to a Pandas DataFrame is a common task in data science and software engineering. The read_json()
function provided by the Pandas library makes this task easy and straightforward. In this article, we discussed how to use the read_json()
function to convert JSON data to a Pandas DataFrame and how to handle nested JSON data. With this knowledge, you can now easily work with JSON data in your data analysis and manipulation tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.