Python Pandas Find difference between two data frames
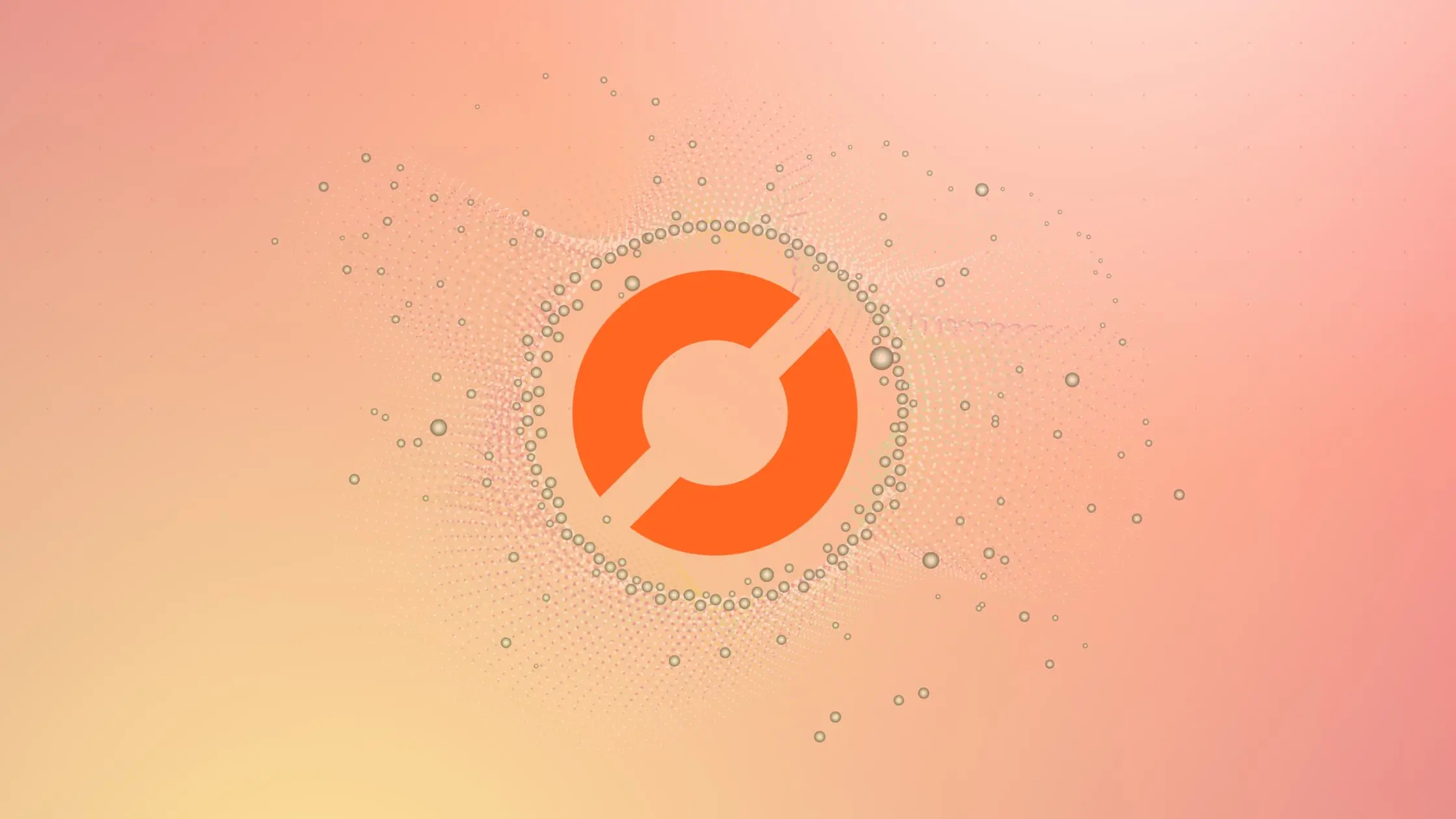
As a data scientist or software engineer, you may often need to compare two data frames to identify the differences between them. This is a common task in data analysis, where you need to identify changes in your data over time or between different datasets. Python’s Pandas library provides powerful tools for working with data frames, including functions for comparing and merging data frames. In this article, we will explore how to find the difference between two data frames using Pandas.
Table of Contents
What is Pandas?
Pandas is a popular Python library for data manipulation and analysis. It provides data structures for efficiently storing and manipulating tabular data, such as Excel spreadsheets or SQL tables. Pandas also provides powerful functions for data cleaning, transformation, and analysis, making it a popular choice for data scientists and analysts.
How to compare two data frames in Pandas
Using pd.DataFrame.equals() Method
The equals()
method is a straightforward way to check if two DataFrames are equal. It returns a boolean value, indicating whether the two DataFrames are identical or not.
Pros
- Simple and easy to use.
- Suitable for quick checks on small to medium-sized DataFrames.
Cons
- May not provide detailed information about specific differences.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
result = df1.equals(df2)
print(result) # Output: True
Using pd.DataFrame.compare() Method
Introduced in Pandas version 1.1.0 and later, the compare()
method performs an element-wise comparison of two DataFrames. It returns a new DataFrame highlighting the differences between the two.
Pros
- Provides a detailed breakdown of differences at the element level.
- Suitable for exploring specific variations in values.
Cons
- Requires Pandas version 1.1.0 or later.
- May result in a large output for DataFrames with many differences.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 4], 'B': [4, 5, 7]})
df_diff = df1.compare(df2)
print(df_diff)
Output:
A B
self other self other
2 3.0 4.0 6.0 7.0
Using pd.concat() and drop_duplicates()
Concatenating two DataFrames and dropping duplicates allows you to retain only the rows unique to each DataFrame, effectively highlighting the differences.
Pros
- Simple and efficient for identifying differing rows.
- Suitable for comparing entire rows.
Cons
- Ignores differences within rows and focuses on entire row mismatches.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 4], 'B': [4, 5, 7]})
df_diff = pd.concat([df1, df2]).drop_duplicates(keep=False)
print(df_diff)
Output:
A B
2 3 6
2 4 7
Using merge() with Indicator
Merging DataFrames with the merge()
function and specifying indicator=True
creates a special column indicating the source of each row (left_only, right_only, or both). This method is useful for identifying the source of discrepancies.
Pros
- Clearly indicates the origin of each differing row.
- Allows customization of merge options.
Cons
Requires additional steps to filter and extract differing rows.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 4], 'B': [4, 5, 7]})
df_diff = pd.merge(df1, df2, how='outer', indicator=True).query('_merge != "both"').drop('_merge', axis=1)
print(df_diff)
Output:
A B
2 3 6
3 4 7
Using Boolean Indexing
Creating boolean masks based on whether rows are present in one DataFrame but not the other allows you to extract the differing rows.
Pros
- Offers fine-grained control over which rows are considered different.
- Can be customized for specific comparison criteria.
Cons
- Requires additional boolean masking steps.
- May be less intuitive for beginners.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 4], 'B': [4, 5, 7]})
mask1 = ~df1.isin(df2).all(axis=1)
mask2 = ~df2.isin(df1).all(axis=1)
df_diff = df1[mask1]._append(df2[mask2])
print(df_diff)
Output:
A B
2 3 6
3 4 7
Error Handling
Missing Columns: When creating data frames for comparison, it’s crucial to ensure that both data frames have a common column for merging. Failure to do so will result in a KeyError.
Mismatched Data Types: Ensure that the common column used for merging has the same data type in both data frames. Mismatched data types may lead to unexpected results or errors during the merge operation.
Memory Usage: Large data frames may exceed system memory during the merge operation, leading to MemoryError. Consider using chunking or more memory-efficient strategies for handling substantial datasets.
Conclusion
In conclusion, Pandas offers a variety of methods for comparing and finding differences between two DataFrames. The choice of method depends on factors such as the specific use case, DataFrame size, and desired output format. By understanding the pros and cons of each technique, you can efficiently identify and analyze discrepancies in your data, facilitating a more comprehensive exploration of your datasets.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.