Python Pandas Convert valuecounts Output to Dataframe
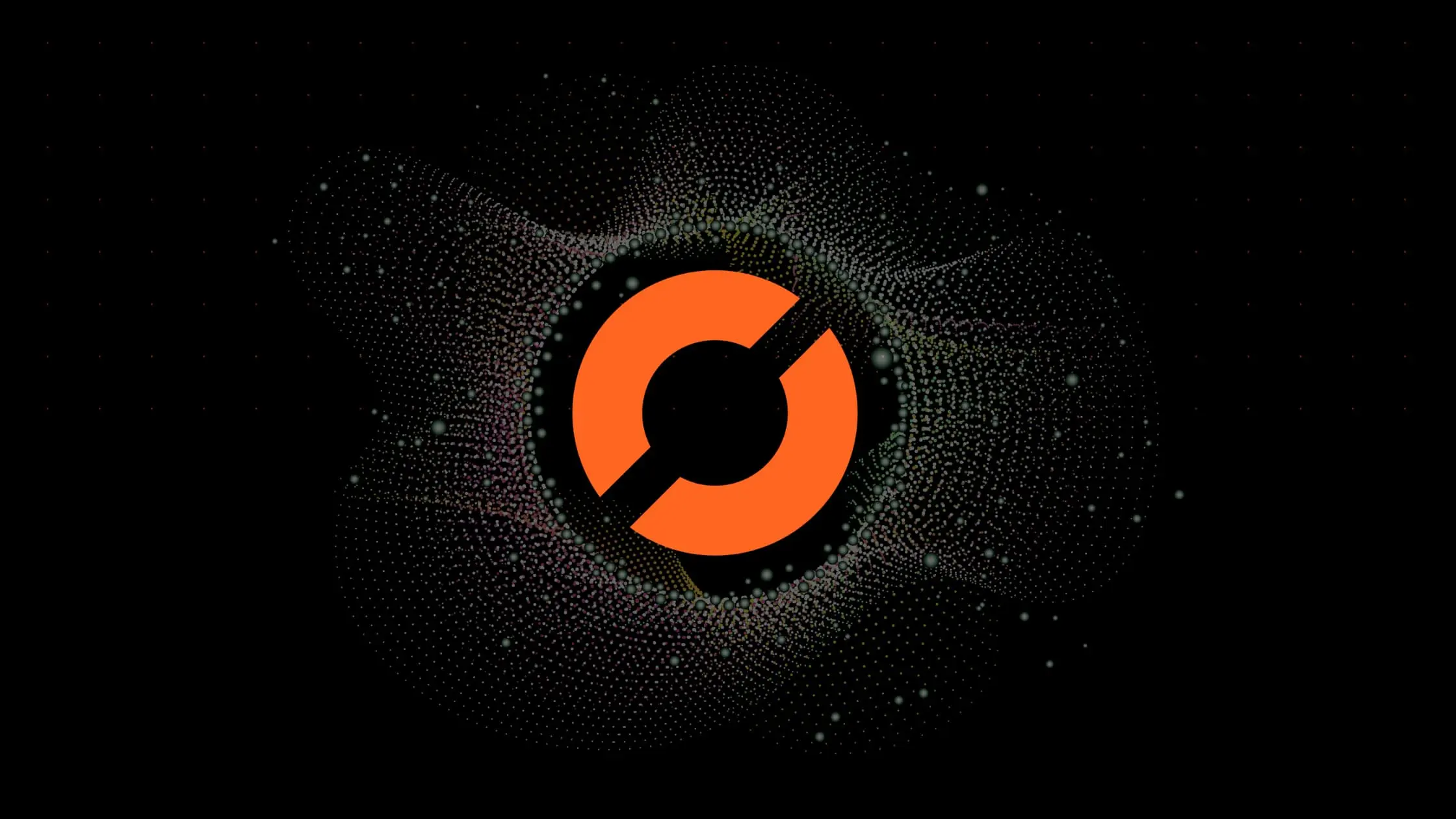
Table of Contents
- What is .value_counts() in Pandas?
- Converting .value_counts() Output to DataFrame
- Use Cases for Converting .value_counts() Output to DataFrame
- Conclusion
As a data scientist or software engineer, you might often encounter the need to analyze data using Python. One of the most popular libraries for data analysis in Python is Pandas. It offers powerful tools to manipulate and analyze data, including methods to count the occurrences of values in a pandas Series or DataFrame.
In this article, we will explore how to convert the output of the .value_counts()
method in Pandas to a DataFrame. We will also discuss some use cases where this conversion can be helpful.
What is .value_counts() in Pandas?
The .value_counts()
method is a convenient way to count the number of occurrences of each unique value in a pandas Series or DataFrame column. It returns a pandas Series object, where each unique value is an index label and its count is the corresponding value.
Here is an example of how to use .value_counts()
:
import pandas as pd
data = pd.Series(["A", "B", "A", "C", "B", "A"])
counts = data.value_counts()
print(counts)
Output:
A 3
B 2
C 1
dtype: int64
As you can see, the output of .value_counts()
is a pandas Series with the unique values as index labels and their counts as the corresponding values.
Converting .value_counts() Output to DataFrame
While the output of .value_counts()
is useful, sometimes you might need to convert it to a pandas DataFrame for further analysis. In particular, DataFrame format is more flexible and can be easier to manipulate than Series format in many cases.
To convert .value_counts()
output to a DataFrame, you can use the .reset_index()
method followed by the .rename()
method. Here is an example:
import pandas as pd
data = pd.Series(["A", "B", "A", "C", "B", "A"])
counts = data.value_counts().reset_index().rename(columns={"index": "value", 0: "count"})
print(counts)
Output:
value count
0 A 3
1 B 2
2 C 1
As you can see, the output is now a pandas DataFrame, where the unique values are in the “value” column and their counts are in the “count” column.
Use Cases for Converting .value_counts() Output to DataFrame
Converting .value_counts()
output to a DataFrame can be helpful in various scenarios. Here are some use cases:
1. Plotting the results
If you want to visualize the results of .value_counts()
, converting the output to a DataFrame can be helpful. You can use the DataFrame to create different types of plots, such as bar charts or pie charts.
Here is an example of how to create a bar chart from the DataFrame created earlier:
import matplotlib.pyplot as plt
plt.bar(counts["value"], counts["count"])
plt.show()
2. Combining with other DataFrames
If you have multiple DataFrames with similar columns, you might want to combine them into one DataFrame. In this case, converting the .value_counts()
output to a DataFrame can be helpful to ensure consistency in the column names.
Here is an example of how to combine two DataFrames using the .merge()
method:
import pandas as pd
df1 = pd.DataFrame({"value": ["A", "B", "C"], "count": [1, 2, 3]})
df2 = pd.DataFrame({"value": ["D", "E", "F"], "count": [4, 5, 6]})
counts = pd.concat([df1, df2], ignore_index=True).groupby("value")["count"].sum().reset_index()
print(counts)
Output:
value count
0 A 1
1 B 2
2 C 3
3 D 4
4 E 5
5 F 6
As you can see, the .value_counts()
output has been converted to a DataFrame with the same column names as the other DataFrames.
Conclusion
In this article, we have explored how to convert the output of the .value_counts()
method in Pandas to a DataFrame. We have also discussed some use cases where this conversion can be helpful.
Converting .value_counts()
output to a DataFrame can be useful in various scenarios, such as plotting the results or combining with other DataFrames. By following the steps outlined in this article, you can easily convert the output to a Pandas DataFrame and continue your data analysis journey.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.