Python Pandas: Conditionally Delete Rows
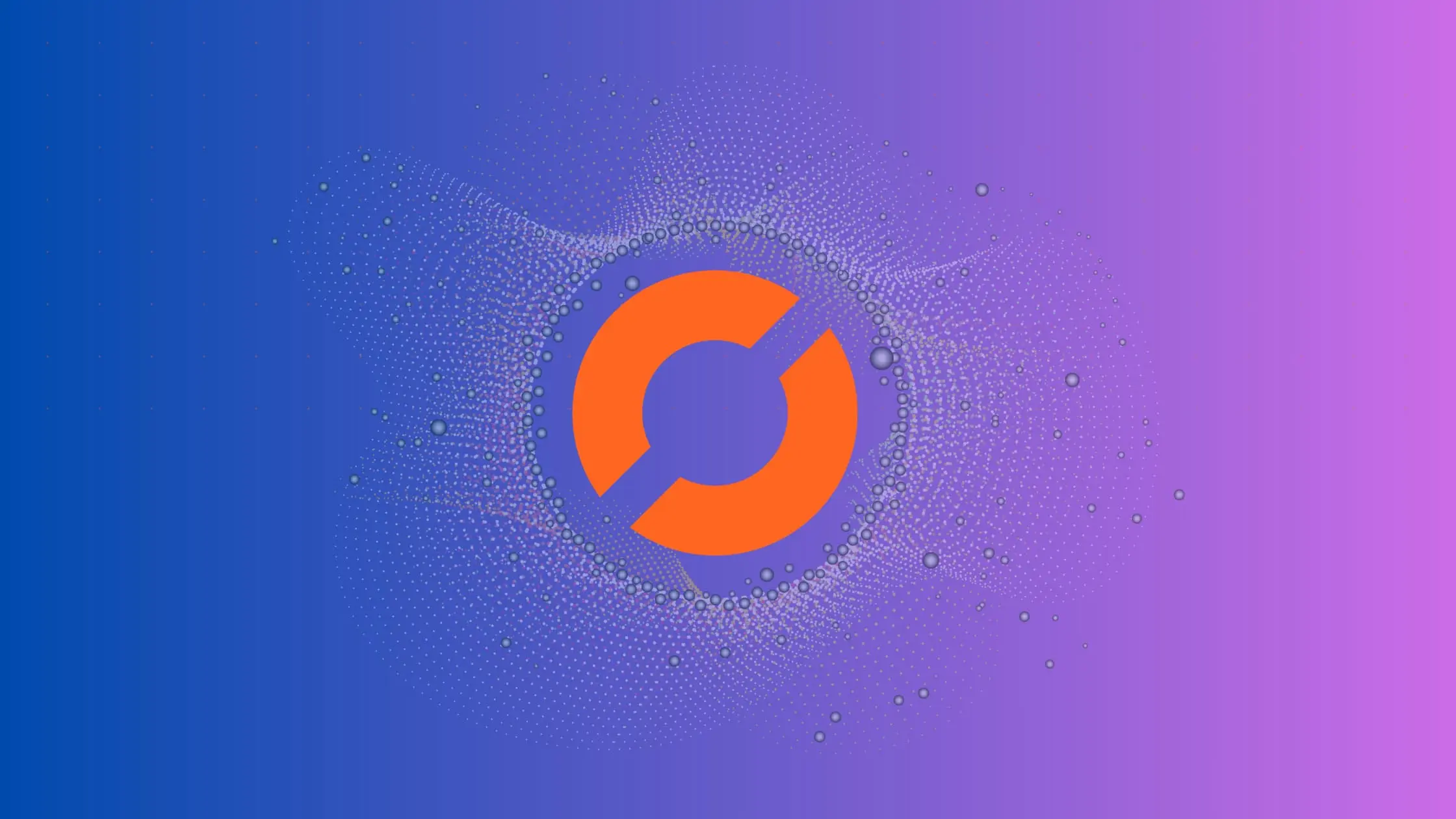
Python Pandas: Conditionally Delete Rows
As a data scientist or software engineer, you’re likely to work with large datasets that require cleaning and pre-processing before they can be used for analysis and modeling. One common task is to delete rows that meet certain conditions, such as those with missing or irrelevant data. In this article, we’ll explore how to conditionally delete rows in Python Pandas, a powerful data manipulation library.
What is Python Pandas?
Python Pandas is a popular data analysis library that provides easy-to-use data structures and functions for manipulating and analyzing tabular data. It is built on top of NumPy, another popular scientific computing library, and provides additional functionality for data manipulation, cleaning, and visualization.
One of the key features of Pandas is the DataFrame, a two-dimensional table-like data structure that can store heterogeneous data types. It provides many functions for working with data frames, including filtering, sorting, merging, and grouping.
How to Conditionally Delete Rows in Pandas
To conditionally delete rows in Pandas, the easiest way is to use boolean indexing. We can aslo use the drop()
function which removes rows or columns based on their labels or positions, query()
function which allows you to filter rows using a SQL-like syntax, or loc
functions, which lets you select rows where a condition is met, similar to boolean indexing.
Here’s an example of how to conditionally delete rows based on a condition in a Pandas data frame. Let’s say we need to remove rows where the age is greater than 30`:
import pandas as pd
# create a sample data frame
data = {'name': ['Alice', 'Bob', 'Charlie', 'Dave'],
'age': [25, 30, 35, 40],
'gender': ['F', 'M', 'M', 'M']}
df = pd.DataFrame(data)
Using boolean indexing:
# conditionally delete rows where age is greater than 30
df_new = df[df['age'] <= 30]
print(df_new)
Using drop()
:
# conditionally delete rows where age is greater than 30
df_new = df.drop(df[df['age'] > 30].index)
print(df_new)
Using query()
:
# conditionally delete rows where age is greater than 30
df_new = df.query('age <= 30')
print(df_new)
Using loc
:
# conditionally delete rows where age is greater than 30
df_new = df.loc[df['age'] <= 30]
print(df_new)
Each of the methods described above will yield the same outcome as follows:
name age gender
0 Alice 25 F
1 Bob 30 M
It’s important to note that these operations create a new DataFrame or modify the existing one, so make sure to assign the result back to your DataFrame if you want to keep the changes.
Conclusion
In this article, we’ve explored how to conditionally delete rows in a Pandas DataFrame, a crucial skill for data cleaning and preparation in data analysis and manipulation. Python’s Pandas library offers various methods, such as boolean indexing, the query
method, drop
, and loc
, to filter and delete rows based on specific conditions. Choosing the right method depends on your specific use case and your preference for coding style.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.