Python - Transforming Lists into Pandas DataFrames
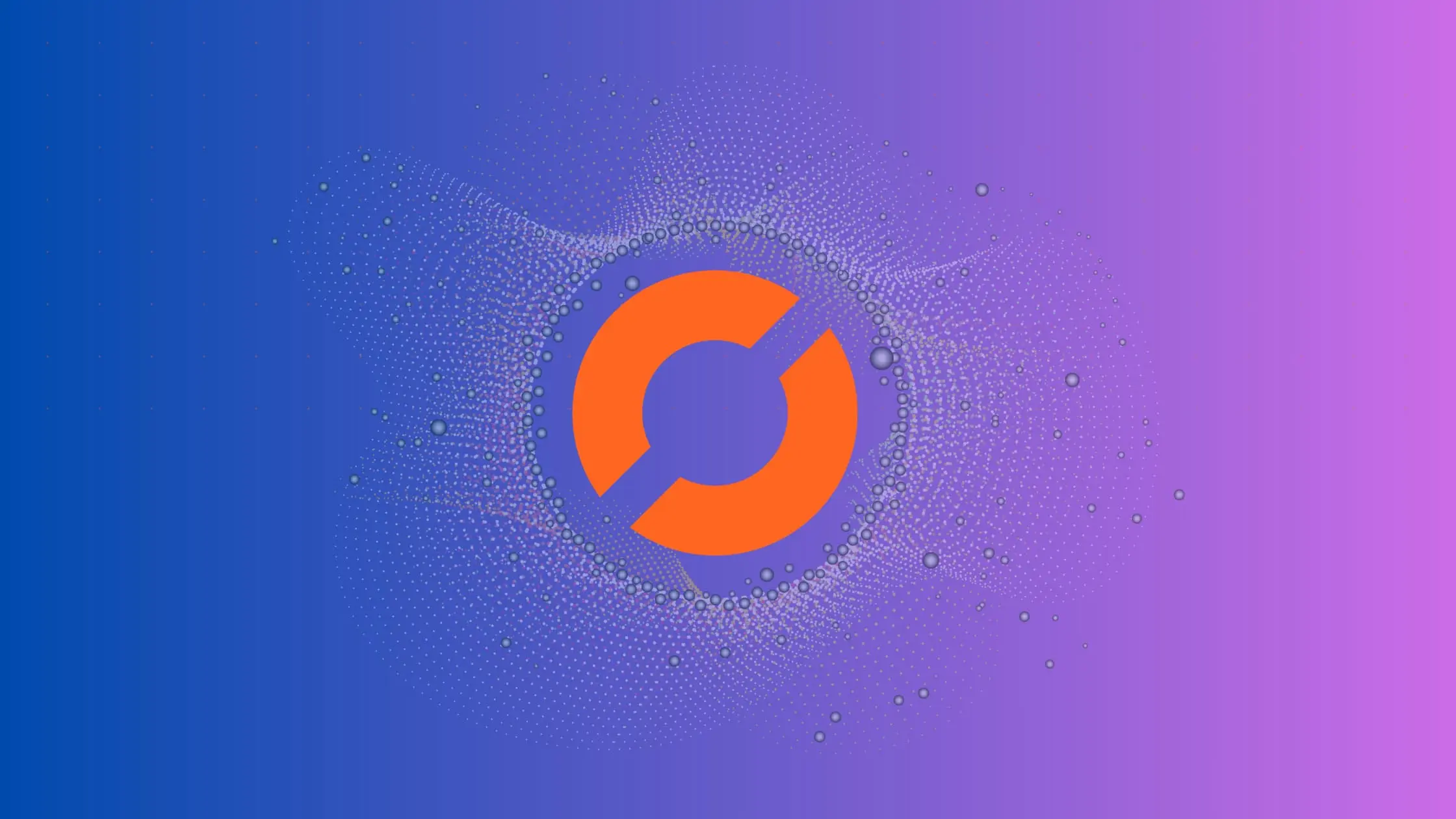
In this article, we’ll explore different methods to achieve this transformation, providing step-by-step guidance and code examples. Additionally, we’ll delve into the advantages of utilizing Pandas DataFrames to enhance your data analysis workflows.
Table of Contents
Understanding Pandas DataFrame
Before delving into the conversion process, let’s grasp what a Pandas DataFrame is and why it proves beneficial. A Pandas DataFrame is a two-dimensional tabular data structure used for storing and manipulating data in Python. Resembling a spreadsheet or SQL table, it consists of rows and columns that can be indexed and labeled.
Pandas DataFrames are widely employed in data analysis and manipulation due to their flexibility and potency. They excel in handling large datasets, supporting various data formats, and offering a plethora of functions and methods for data analysis and manipulation.
Methods of Converting Python Lists to Pandas DataFrames
Now that we comprehend the essence of a Pandas DataFrame, let’s explore multiple approaches to convert a Python list into one. The process involves the following steps:
Method 1: Direct Conversion
You can directly convert a list of lists to a DataFrame using pd.DataFrame()
:
data = [['John Smith', 50000, 25], ['Jane Doe', 60000, 30], ['Bob Johnson', 55000, 28]]
df = pd.DataFrame(data, columns=['Name', 'Salary', 'Age'])
print(df)
Output:
Name Salary Age
0 John Smith 50000 25
1 Jane Doe 60000 30
2 Bob Johnson 55000 28
Method 2: Using Dictionaries
- create a Python list that contains your data. For example, let’s say you have a list of employee names, salaries, and ages:
employee_names = ['John Smith', 'Jane Doe', 'Bob Johnson']
employee_salaries = [50000, 60000, 55000]
employee_ages = [25, 30, 28]
- Create a Dictionary from the Python List
After creating the Python list, the next step is to convert it into a dictionary. A dictionary is a key-value data structure that allows you to store and access data using keys rather than indices.
In our example, we can create a dictionary that maps each employee attribute to its corresponding list:
employee_data = {'Name': employee_names, 'Salary': employee_salaries, 'Age': employee_ages}
- Convert the Dictionary to a Pandas DataFrame
Finally, we can convert the dictionary into a Pandas DataFrame using the pd.DataFrame()
function:
df = pd.DataFrame(employee_data)
print(df)
Name Salary Age
0 John Smith 50000 25
1 Jane Doe 60000 30
2 Bob Johnson 55000 28
This code creates a new Pandas DataFrame called df
that contains the employee data in tabular form.
Method 3: Using NumPy Arrays:
Leverage NumPy arrays to create a DataFrame:
import numpy as np
data_array = np.array([employee_names, employee_salaries, employee_ages]).T
df = pd.DataFrame(data_array, columns=['Name', 'Salary', 'Age'])
print(df)
Output:
Name Salary Age
0 John Smith 50000 25
1 Jane Doe 60000 30
2 Bob Johnson 55000 28
Benefits of Working with Pandas DataFrames
Now that we have seen how to convert a Python list to a Pandas DataFrame, let’s briefly discuss some of the benefits of working with Pandas DataFrames.
Seamless Data Manipulation: Pandas DataFrames offer an array of functions for data manipulation, facilitating tasks like filtering, sorting, grouping, and aggregation.
Robust Data Analysis: With built-in statistical and mathematical functions, Pandas DataFrames empower you with tools for analysis, including mean, median, standard deviation, and correlation.
Integration with Other Libraries: Pandas DataFrames easily integrate with other Python libraries like Matplotlib for data visualization and Scikit-Learn for machine learning, enabling comprehensive data analysis workflows.
Pros and Cons
Method 1: Direct Conversion (pd.DataFrame())
Pros: simple and concise, good for small datasets, and allows specifying columns with a list.
Cons: only handles lists of lists or single-dimensional lists and less readable.
Method 2: Using Dictionaries
Pros: clearly maps keys to data columns for clarity, can handle different data types in each column, and easy column customization: Specify column names and order explicitly.
Cons: more complex, requires multiple steps, code might seem excessive for basic lists, and there is a potential for duplicate keys.
Method 3: Using NumPy Arrays
Pros: efficient for large datasets, flexible data types, and can leverage NumPy operations like using array manipulation before converting to DataFrame.
Cons: most complex, not beginner-friendly, might require NumPy understanding.
Overall: The best method depends on your specific needs and data complexity.
- For quick and simple conversions, use direct conversion (pd.DataFrame()).
- For organized data with different types, leverage dictionaries.
- For large datasets or needing NumPy operations, consider using NumPy arrays.
By understanding the pros and cons of each method, you can choose the right tool for efficiently and effectively converting your Python lists to Pandas DataFrames.
Conclusion
Transforming a Python list into a Pandas DataFrame is a straightforward process that enhances your efficiency in working with data. Pandas DataFrames provide flexibility and power, making them indispensable for data scientists and software engineers. By following the steps outlined in this article and exploring additional conversion methods, you can seamlessly convert your Python lists into Pandas DataFrames and leverage their myriad benefits for enhanced data analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.