Python Classification with Lasso: How to Predict Classes
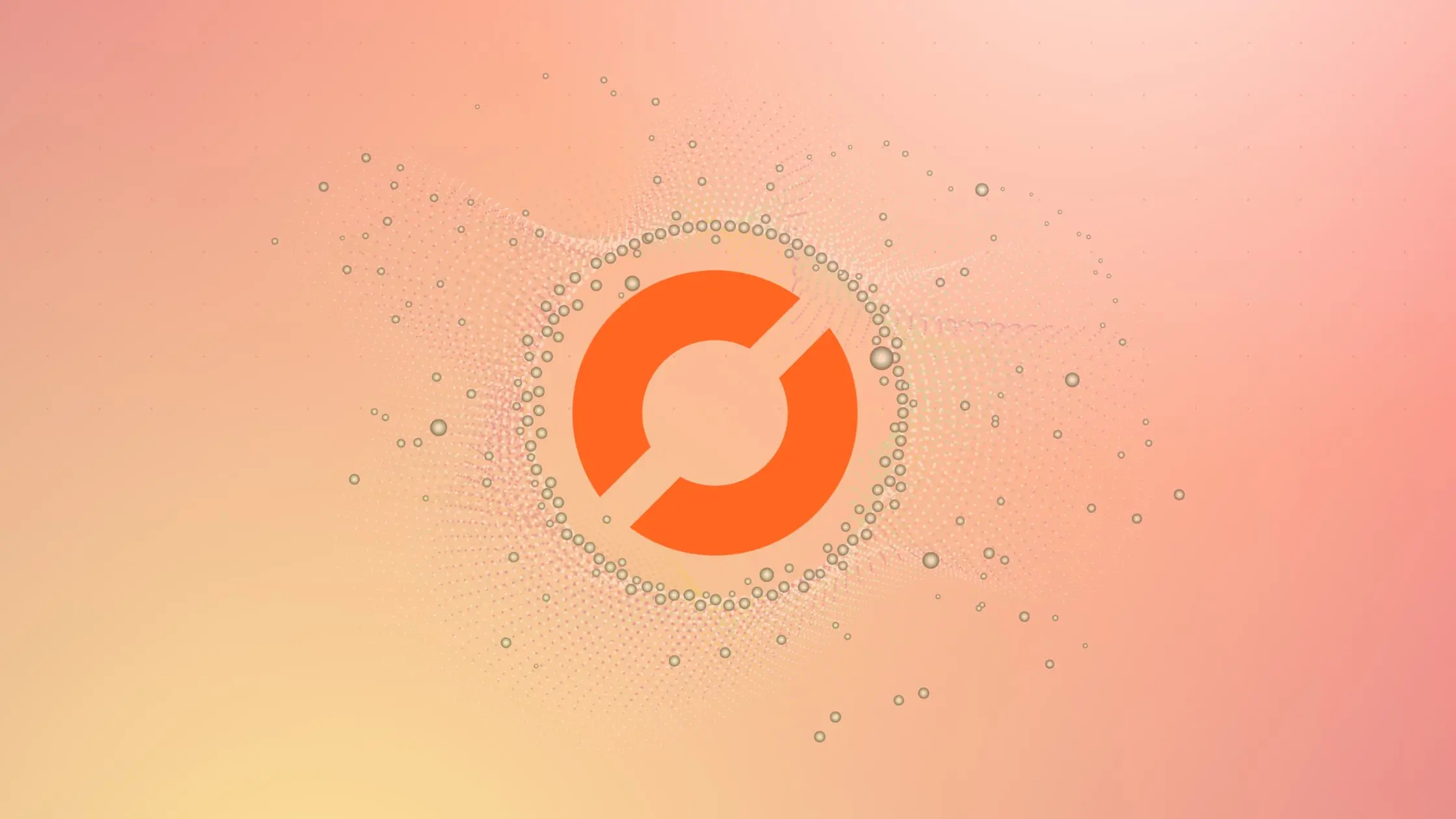
As a data scientist or software engineer, you know that classification is a fundamental task in machine learning. It involves predicting discrete labels based on input features, and it’s used in a wide range of applications, from fraud detection to image recognition. In this post, we’ll focus on the Lasso algorithm for classification in Python, and we’ll show you how to predict classes using scikit-learn.
Table of Contents
- What is Lasso?
- How to Use Lasso for Classification
- Pros and Cons of Lasso
- Tips for Using Lasso for Classification
- Conclusion
What is Lasso?
Lasso is a linear model that performs both variable selection and regularization. It’s similar to Ridge regression, which also performs regularization, but Lasso has the additional property of setting some coefficients to zero. This makes Lasso useful for feature selection, as it can identify the most important features for the task at hand.
In classification, Lasso is often used to perform binary classification, where the goal is to predict one of two possible labels. Lasso works by fitting a hyperplane that separates the data into two classes, and it uses a regularization parameter that controls the strength of the penalty for large coefficients.
How to Use Lasso for Classification
To use Lasso for classification in Python, you’ll need to install scikit-learn, a popular machine learning library. Once you have scikit-learn installed, you can create a Lasso classifier using the Lasso
class:
from sklearn.linear_model import Lasso
lasso_classifier = Lasso(alpha=0.1)
Here, we’re creating a Lasso classifier with an alpha value of 0.1. The alpha value controls the strength of the regularization penalty, and higher values of alpha will result in more coefficients being set to zero. You can experiment with different values of alpha to find the best value for your data.
Next, you’ll need to fit the Lasso classifier to your data. You can do this using the fit
method:
lasso_classifier.fit(X_train, y_train)
Here, X_train
is a matrix of input features, and y_train
is a vector of corresponding labels. The fit
method will adjust the coefficients of the Lasso model to best fit the training data.
Once you’ve fit the model, you can use it to predict labels for new data using the predict
method:
y_pred = lasso.predict(X_test)
Here, X_test
is a matrix of input features for the test data, and y_pred
is a vector of predicted labels. You can then evaluate the accuracy of your model using a metric such as accuracy or F1 score.
Complete Code:
# Import necessary libraries
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import Lasso
from sklearn.metrics import accuracy_score, confusion_matrix
# Load the Iris dataset
from sklearn.datasets import load_iris
iris = load_iris()
X, y = iris.data, iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Implement Lasso regression for classification
lasso_classifier = Lasso(alpha=0.1)
lasso_classifier.fit(X_train, y_train)
# Make predictions
y_pred = lasso_classifier.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred.round())
conf_matrix = confusion_matrix(y_test, y_pred.round())
print(f"Accuracy: {accuracy}")
print(f"Confusion Matrix:\n{conf_matrix}")
Pros and Cons of Lasso
Pros:
- Feature Selection: Lasso automatically selects relevant features by assigning zero coefficients, reducing dimensionality and improving model generalization.
- Regularization: Prevents overfitting by penalizing large coefficients, leading to a more robust model, especially in scenarios with a high feature-to-sample ratio.
- Interpretability: Sparsity induced by Lasso makes the model easier to interpret, focusing on a subset of features that significantly contribute to predictions.
Cons:
- Selection Bias: Tends to select only one correlated feature from a group, potentially introducing selection bias.
- Sensitivity to Scaling: Lasso is sensitive to input feature scales, necessitating standardization or normalization.
Tips for Using Lasso for Classification
When using Lasso for classification, there are a few tips to keep in mind:
Normalize your data: Lasso is sensitive to the scale of the input features, so it’s important to normalize your data before fitting the model. You can do this using the
StandardScaler
class in scikit-learn.Tune the alpha value: The choice of alpha can have a big impact on the performance of your model. It’s important to experiment with different values of alpha to find the best value for your data.
Use cross-validation: Cross-validation can help you avoid overfitting your model to the training data. You can use the
GridSearchCV
class in scikit-learn to perform cross-validation and tune the hyperparameters of your model.
Conclusion
Lasso is a powerful algorithm for classification in Python, and it’s particularly useful for feature selection. By setting some coefficients to zero, Lasso can identify the most important features for the task at hand. To use Lasso for classification, you’ll need to install scikit-learn and create a Lasso classifier with an appropriate value of alpha. You can then fit the model to your data and use it to predict labels for new data. Remember to normalize your data and tune the alpha value for best results, and use cross-validation to avoid overfitting your model. With these tips in mind, you’ll be well on your way to building accurate and effective classifiers with Lasso.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.