Pandas vs. Scikit-learn: One-Hot Encoding Dataframes
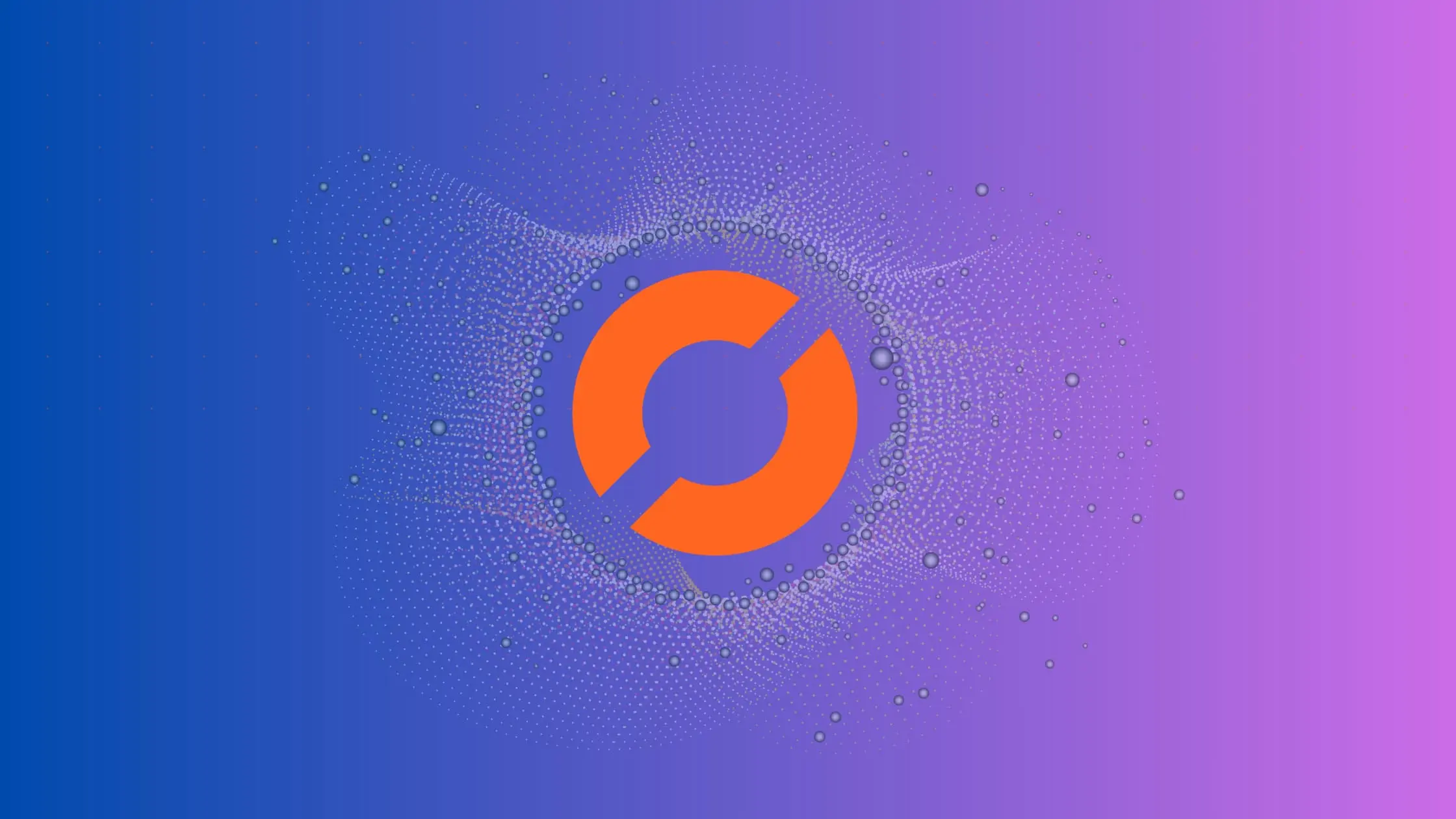
Table of Contents
- What is one-hot encoding?
- Pandas for one-hot encoding
- Scikit-learn for one-hot encoding
- When to use Pandas vs. Scikit-learn for one-hot encoding
- Common Errors and Troubleshooting
- Conclusion
As a data scientist or software engineer, you have likely encountered the need to one-hot encode categorical variables in your datasets. One-hot encoding is a common technique used in machine learning to transform categorical data into numerical data, making it easier for machine learning algorithms to understand and process the data.
When it comes to one-hot encoding dataframes in Python, two popular libraries stand out: Pandas and Scikit-learn. In this blog post, we will explore the differences between the two libraries and discuss when you should use one over the other.
What is one-hot encoding?
Before diving into the differences between Pandas and Scikit-learn, let’s first define what one-hot encoding is.
One-hot encoding is a process of converting categorical variables into a binary representation usable in machine learning algorithms. It involves creating a new column for each unique value in the categorical variable and encoding the presence of that value as a 1 in the corresponding column and 0’s in the other columns.
For example, suppose you have a dataset containing a categorical variable called "color"
with three unique values: "red"
, "blue"
, and "green"
. One-hot encoding this variable would create three new columns: "color_red"
, "color_blue"
, and "color_green"
, where each row contains a 1 in the column corresponding to the color the row represents and 0’s in the other columns.
One-hot encoding is important because many machine learning algorithms cannot process categorical data directly. By one-hot encoding the data, we can represent categorical variables in a way that is compatible with these algorithms.
Pandas for one-hot encoding
Pandas is a popular Python library for data manipulation and analysis. It provides a range of functions for cleaning, transforming, and analyzing data, including one-hot encoding.
Pandas provides the get_dummies()
function to one-hot encode categorical variables. This function takes a pandas dataframe as its input and returns a new dataframe with the one-hot encoded columns added.
Here is an example:
import pandas as pd
# create example dataframe
data = {'color': ['red', 'blue', 'green', 'red', 'blue']}
df = pd.DataFrame(data)
# one-hot encode color column
one_hot_df = pd.get_dummies(df['color'], prefix='color')
print(one_hot_df)
Output:
color_blue color_green color_red
0 0 0 1
1 1 0 0
2 0 1 0
3 0 0 1
4 1 0 0
As you can see, get_dummies()
creates a new dataframe with three columns, one for each unique value in the "color"
column.
Pandas also provides several options for customizing the one-hot encoding process, such as specifying the prefix for the new columns and handling missing values.
Scikit-learn for one-hot encoding
Scikit-learn is a popular Python library for machine learning. It provides a range of functions for modeling data, including one-hot encoding.
Scikit-learn provides the OneHotEncoder()
class to one-hot encode categorical variables. This class takes a numpy array or pandas dataframe as its input and returns a new numpy array with the one-hot encoded columns added.
Here is an example:
import pandas as pd
from sklearn.preprocessing import OneHotEncoder
# create example dataframe
data = {'color': ['red', 'blue', 'green', 'red', 'blue']}
df = pd.DataFrame(data)
# create OneHotEncoder object
encoder = OneHotEncoder()
# fit and transform color column
one_hot_array = encoder.fit_transform(df[['color']]).toarray()
# create new dataframe from numpy array
one_hot_df = pd.DataFrame(one_hot_array, columns=encoder.get_feature_names())
print(one_hot_df)
Output:
x0_blue x0_green x0_red
0 0.0 0.0 1.0
1 1.0 0.0 0.0
2 0.0 1.0 0.0
3 0.0 0.0 1.0
4 1.0 0.0 0.0
As you can see, OneHotEncoder()
creates a new numpy array with three columns, one for each unique value in the “color” column. We then create a new pandas dataframe from this numpy array.
Scikit-learn also provides several options for customizing the one-hot encoding process, such as specifying the categories to encode and handling unknown categories.
When to use Pandas vs. Scikit-learn for one-hot encoding
Before going further, let’s consider the Pros and Cons of each library.
Pandas
- Pros:
- Straightforward implementation.
- Integration with existing Pandas workflows.
- Customization options for column names.
- Cons:
- May not be memory-efficient for large datasets.
- Can lead to a large number of columns for high-cardinality categorical features.
Scikit-learn
- Pros:
- Efficient handling of large datasets.
- Integration with Scikit-learn pipelines.
- Option for handling unseen categories during encoding.
- Cons:
- Requires a two-step process (fitting and transforming).
- The output is a SciPy sparse matrix by default.
So, which library should you use for one-hot encoding dataframes in Python? The answer depends on your specific use case.
Pandas: Ideal when working primarily with pandas dataframes, offering a simple and flexible way to one-hot encode categorical variables. The resulting dataframe can be easily merged with the original dataframe.
Scikit-learn: Preferable when primarily working with scikit-learn for machine learning and needing to integrate one-hot encoding within a larger machine learning pipeline. It seamlessly integrates with other scikit-learn functions.
Common Errors and Troubleshooting
Error: Memory Exhaustion:
When using pandas, if the dataframe is too large, memory errors may occur.
pd.get_dummies(df, columns=['category'])
Solution: Use the sparse option to create sparse matrices, reducing memory usage.
pd.get_dummies(df, columns=['category'], sparse=True)
Error: Unseen Categories During Transformation:
When using Scikit-learn, if new categories appear during the transformation step, an error may occur.
encoder.transform(new_df)
Solution: Set handle_unknown=‘ignore’ during initialization to handle unseen categories gracefully.
encoder = OneHotEncoder(handle_unknown='ignore')
Conclusion
In summary, both Pandas and Scikit-learn provide powerful tools for one-hot encoding dataframes in Python. By understanding the differences between the two libraries, you can choose the one that best fits your specific use case and data processing needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.