Pandas Pivot Table List of Aggfunc A Guide
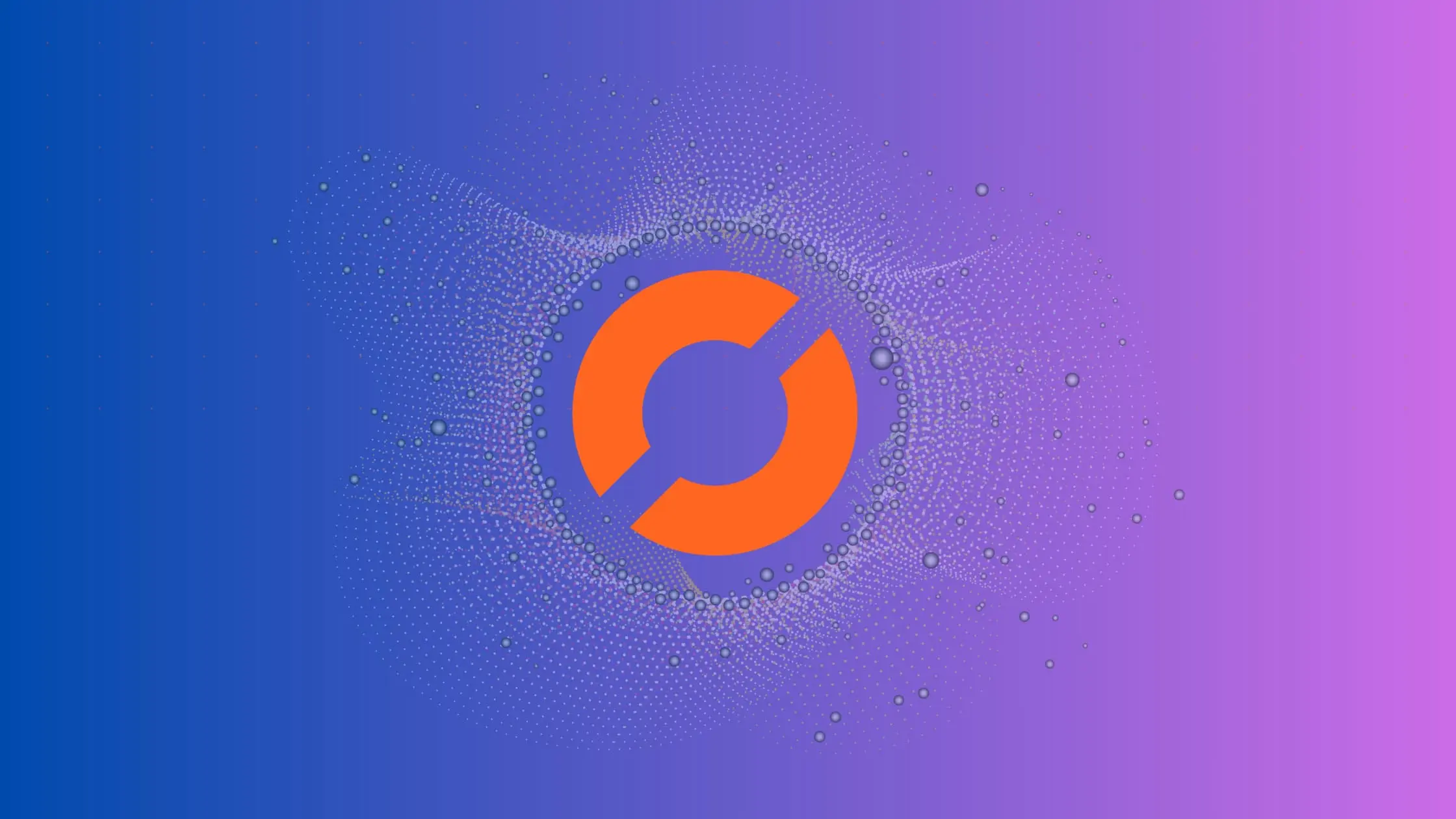
As a data scientist or software engineer, you are likely to work with large datasets that require extensive analysis and manipulation. One of the most powerful tools in your arsenal is the Pandas library, which provides a wide range of functions for data manipulation and analysis. In particular, the Pandas pivot table function is a powerful tool for summarizing and aggregating data, which can be used to quickly analyze large datasets and derive meaningful insights. In this post, we will explore the Pandas pivot table list of aggfunc, which is an important aspect of this powerful tool.
Table of Contents
- What is a Pivot Table?
- How to Create a Pivot Table in Pandas
- Understanding the List of Aggfunc
- Examples of Using Aggfunc in a Pivot Table
- Pros and Cons of Using Pandas Pivot Table with Aggfunc
- Error Handling in Pandas Pivot Table with Aggfunc
- Conclusion
What is a Pivot Table?
A pivot table is a data summarization tool that allows you to quickly extract insights from large datasets. The basic idea behind a pivot table is to take a large dataset and summarize it in a way that makes it easier to analyze. Pivot tables are particularly useful for working with datasets that have multiple dimensions, such as time, geography, or product categories. By summarizing large datasets in this way, you can quickly identify patterns and trends that might otherwise be difficult to detect.
How to Create a Pivot Table in Pandas
Creating a pivot table in Pandas is a straightforward process that can be accomplished with just a few lines of code. The basic syntax for creating a pivot table in Pandas is as follows:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'Product': ['A', 'A', 'A', 'B', 'B', 'B', 'C', 'C', 'C',],
'Region': ['North', 'South', 'North', 'North', 'South', 'South', 'North', 'South', 'South'],
'Sales': [125, 250, 170, 230, 195, 215, 150, 250, 175]
})
# Create a pivot table
pivot_table = pd.pivot_table(df, values='Sales', index='Product', columns='Region', aggfunc='sum')
In this example, we create a DataFrame with three columns: Product, Region, and Sales. We then use the pd.pivot_table
function to create a pivot table from this data. The values
parameter specifies the column to be aggregated (in this case, Sales), the index
parameter specifies the row labels (in this case, Product), and the columns
parameter specifies the column labels (in this case, Region). Finally, the aggfunc
parameter specifies the aggregation function to be used (in this case, sum
).
Understanding the List of Aggfunc
The aggfunc
parameter is one of the most important aspects of creating a pivot table in Pandas. This parameter specifies the aggregation function to be used when summarizing the data in the pivot table. There are several different aggregation functions available in Pandas, each of which can be used to summarize data in a different way. The following is a list of the most common aggregation functions used in Pandas:
sum
: Calculates the sum of the values in the specified column(s).mean
: Calculates the mean (average) of the values in the specified column(s).median
: Calculates the median (middle value) of the values in the specified column(s).min
: Returns the minimum value in the specified column(s).max
: Returns the maximum value in the specified column(s).count
: Counts the number of values in the specified column(s).std
: Calculates the standard deviation of the values in the specified column(s).var
: Calculates the variance of the values in the specified column(s).
It is worth noting that the aggfunc
parameter can also accept a custom aggregation function, which can be defined using a lambda function or a user-defined function.
Examples of Using Aggfunc in a Pivot Table
To illustrate how the aggfunc
parameter can be used in a pivot table, let’s consider a few examples. Suppose we have a DataFrame containing information about sales revenue for different products and regions:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'Product': ['A', 'A', 'A', 'B', 'B', 'B', 'C', 'C', 'C',],
'Region': ['North', 'South', 'North', 'North', 'South', 'South', 'North', 'South', 'South'],
'Sales': [125, 250, 170, 230, 195, 215, 150, 250, 175]
})
Suppose we want to create a pivot table that summarizes the total sales revenue for each product in each region. We can use the sum
aggregation function as follows:
# Create a pivot table
pivot_table = pd.pivot_table(df, values='Sales', index='Product', columns='Region', aggfunc='sum')
This will produce a pivot table that looks like this:
Region North South
Product
A 295 250
B 230 410
C 150 425
Suppose we want to create a pivot table that summarizes the average sales revenue for each product in each region. We can use the mean
aggregation function as follows:
# Create a pivot table
pivot_table = pd.pivot_table(df, values='Sales', index='Product', columns='Region', aggfunc='mean')
This will produce a pivot table that looks like this:
Region North South
Product
A 147.5 250.0
B 230.0 205.0
C 150.0 212.5
Suppose we want to create a pivot table that summarizes the number of sales for each product in each region. We can use the count
aggregation function as follows:
# Create a pivot table
pivot_table = pd.pivot_table(df, values='Sales', index='Product', columns='Region', aggfunc='count')
This will produce a pivot table that looks like this:
Region North South
Product
A 2 1
B 1 2
C 1 2
Pros and Cons of Using Pandas Pivot Table with Aggfunc:
Pross
Data Summarization: The pivot table, coupled with the aggfunc parameter, allows for efficient summarization and aggregation of large datasets. This is crucial for data scientists and software engineers who need to derive meaningful insights from extensive data.
Multi-Dimensional Analysis: Pivot tables are particularly useful when working with datasets that have multiple dimensions, such as time, geography, or product categories. They enable users to analyze data from different perspectives, making it easier to identify patterns and trends.
Flexibility in Aggregation: The list of aggregation functions provided by Pandas offers flexibility in summarizing data based on specific requirements. Users can choose from common functions like sum, mean, median, min, max, count, std, and var, or even define custom aggregation functions.
Ease of Implementation: Creating a pivot table in Pandas is a straightforward process, requiring just a few lines of code. This simplicity enhances the ease of implementation, allowing users to quickly perform complex data summarization tasks.
Cons
Potential Complexity: As datasets become more complex, the creation of pivot tables with multiple dimensions and various aggregation functions can lead to intricate code and potentially result in challenges in understanding or maintaining the code.
Memory Usage: Depending on the size of the dataset and the chosen aggregation functions, the memory usage for pivot tables can be significant. Users should be mindful of memory constraints, especially when dealing with large datasets.
Loss of Granularity: While pivot tables provide aggregated views of data, the original granularity of individual data points may be lost. This loss of granularity might be a concern if detailed insights at the individual data point level are required.
Error Handling in Pandas Pivot Table with Aggfunc:
Invalid Aggregation Function: Providing an invalid or unsupported aggregation function to the aggfunc parameter can result in an error. Users should consult the list of available aggregation functions and ensure the chosen function is appropriate for the analysis.
Mismatched Data Types: Inconsistent data types in the columns used for aggregation may lead to unexpected errors. Ensuring uniform data types or handling conversions appropriately is essential for error-free execution.
Missing Data Handling: The chosen aggregation function might be sensitive to missing or NaN values in the dataset. Users should consider addressing missing data through preprocessing or using aggregation functions that handle missing values gracefully.
Conclusion
In conclusion, the Pandas pivot table function is a powerful tool for summarizing and aggregating data, which can be used to quickly analyze large datasets and derive meaningful insights. The aggfunc
parameter is an important aspect of this tool, as it allows you to specify the aggregation function to be used when summarizing the data in the pivot table. By understanding the list of aggregation functions available in Pandas, you can create pivot tables that summarize data in a wide range of ways, giving you the flexibility to analyze your data in the most effective way possible.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.