How to Change All the Values of a Column in a Pandas DataFrame
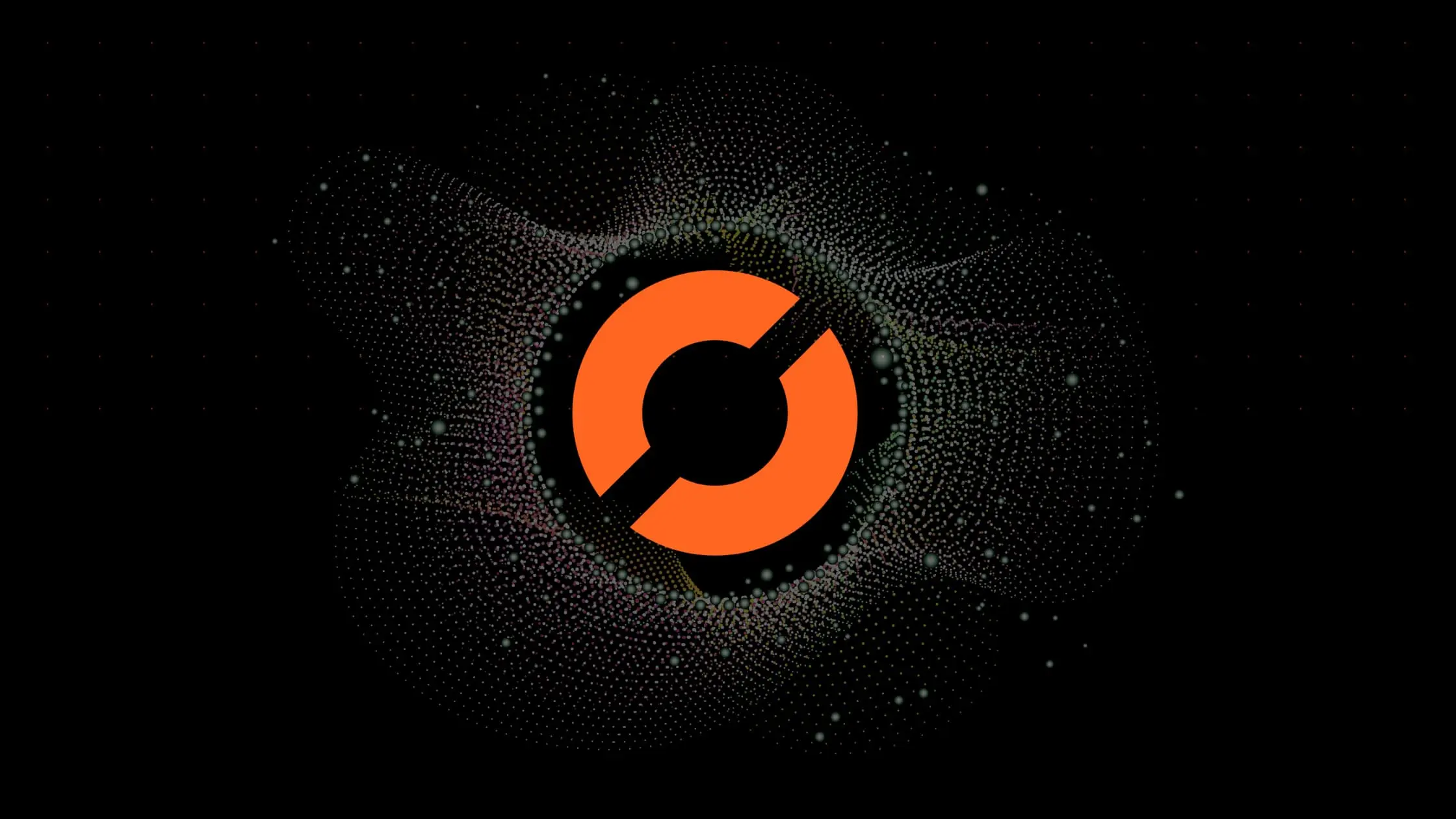
What is Pandas?
Before we dive into how to change column values, let’s briefly review what Pandas is and what it can do. Pandas is a Python library that provides data structures for efficiently storing and manipulating data. It is particularly useful for working with tabular data, such as CSV files or SQL database tables. Pandas provides a variety of functions for performing operations on data, including filtering, sorting, grouping, and aggregation.
How to Change All the Values of a Column in Pandas
Changing all the values of a column in Pandas is a straightforward process. Here are the general steps:
- Select the column you want to change.
- Use the
.apply()
method to apply a function to each value in the column. - Assign the new values back to the column.
Let’s walk through each step in more detail.
Step 1: Select the Column
To select a column in Pandas, you can use the bracket notation with the column name as the key. For example, if you have a DataFrame df
with a column named age
, you can select the column like this:
age_column = df['age']
Step 2: Apply a Function to Each Value
Once you have selected the column, you can use the .apply()
method to apply a function to each value in the column. The function should take a single argument, which will be the current value of the column. It should return the new value that you want to replace the current value with.
For example, let’s say you want to replace all the values in the age
column with their square roots. You could define a function like this:
import numpy as np
def sqrt(x):
return np.sqrt(x)
Then, you can use the .apply()
method to apply this function to each value in the age
column:
new_age_column = age_column.apply(sqrt)
This will create a new Series new_age_column
with the square root of each value in the age
column.
Step 3: Assign the New Values Back to the Column
Finally, you can assign the new values back to the original column. You can do this by using the bracket notation to select the column, and then assigning the new Series to it. For example:
df['age'] = new_age_column
This will replace the age
column in the original DataFrame df
with the new values.
Example: Changing Column Values in a DataFrame
Let’s look at a more concrete example to see how this works in practice. Suppose we have a DataFrame df
with two columns, name
and age
, like this:
import pandas as pd
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35]
})
Suppose we want to change the values in the age
column to their square roots. We can follow the three steps we outlined above:
import numpy as np
# Step 1: Select the column
age_column = df['age']
# Step 2: Apply a function to each value
def sqrt(x):
return np.sqrt(x)
new_age_column = age_column.apply(sqrt)
# Step 3: Assign the new values back to the column
df['age'] = new_age_column
After running these three steps, the df
DataFrame will have the following values:
name age
0 Alice 5.000000
1 Bob 5.477226
2 Charlie 5.916080
As you can see, the values in the age
column have been replaced with their square roots.
Conclusion
In this post, we’ve discussed how to change all the values of a column in Pandas. We’ve outlined the three general steps:
- Select the column you want to change.
- Use the
.apply()
method to apply a function to each value in the column. - Assign the new values back to the column.
We’ve also provided an example to help you understand the process. We hope this post has been helpful in your data manipulation and analysis tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.