Pandas DataFrame Concat vs Append Whats the Difference and When to Use Each
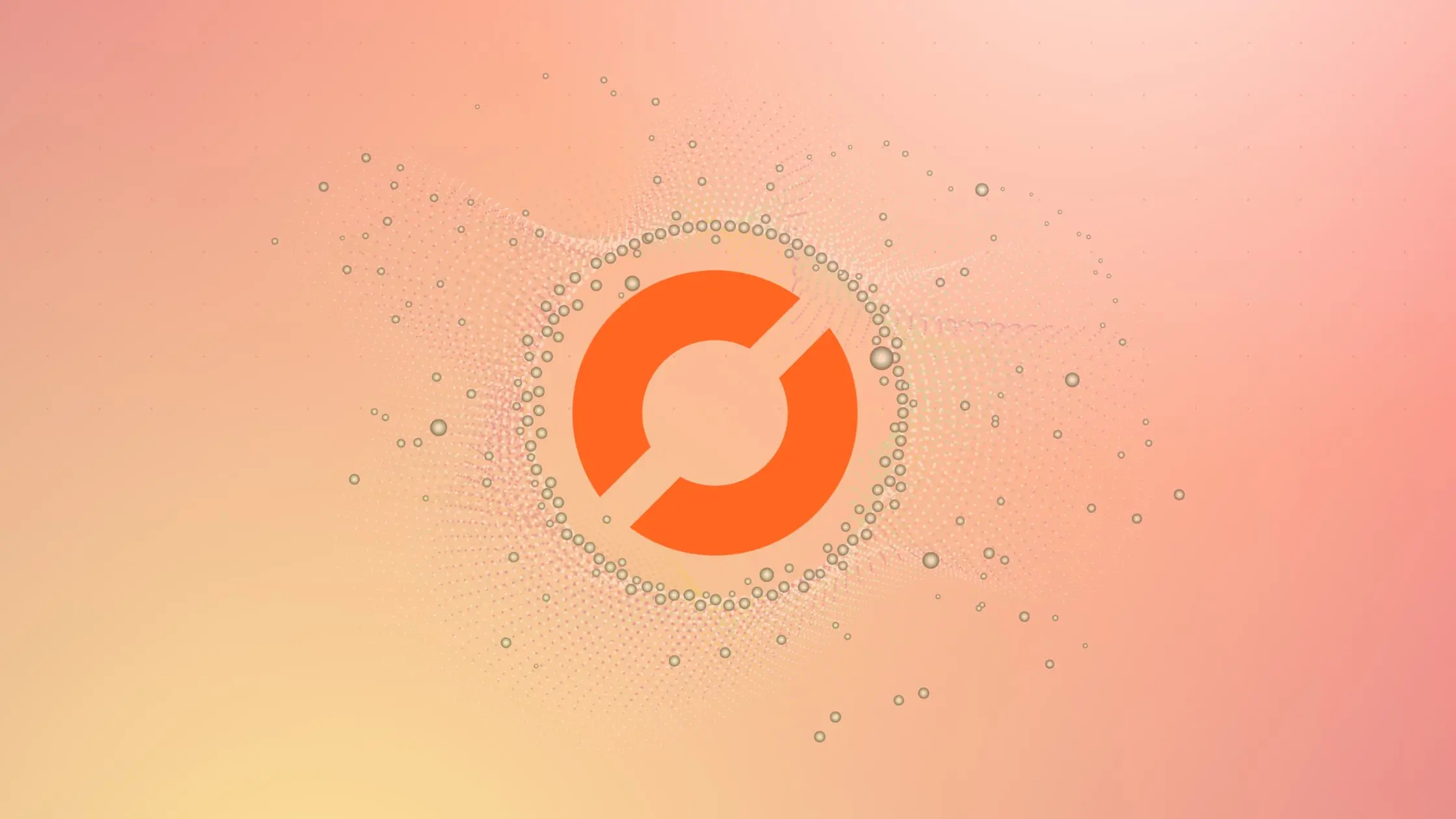
As a data scientist or software engineer, we often work with large datasets that require manipulation and analysis. Pandas is a popular library in Python that offers powerful tools for data manipulation and analysis. One of the most common operations we perform on data is merging or combining multiple data frames. In Pandas, we have two methods for combining data frames: concat
and append
. In this blog post, we will explore the differences between these two methods and when to use each.
Table of Contents
- What is Pandas?
- Concatenation
- Appending
- Differences between Concat and Append
- When to Use Concat vs Append
- Common Errors and How to Handle Them
- Conclusion
What is Pandas?
Before we dive into the differences between concat
and append
, let’s briefly review what Pandas is. Pandas is a Python library built on top of NumPy that provides fast, flexible, and expressive data structures for data manipulation and analysis. Pandas offers two main classes for storing and manipulating data: Series and DataFrame.
A Series is a one-dimensional array-like object that can hold any data type. A DataFrame is a two-dimensional table-like data structure that consists of rows and columns. It is similar to a spreadsheet or SQL table. DataFrames are the most commonly used Pandas object for data manipulation and analysis.
Concatenation
Concatenation is the process of combining two or more objects, in this case, data frames, into a single object. In Pandas, we can use the concat
method to concatenate two or more data frames. The concat
method takes a sequence of data frames and combines them along a specified axis.
import pandas as pd
# create two data frames
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [4, 5, 6], 'B': [7, 8, 9]})
# concatenate the data frames along the rows
concatenated_df = pd.concat([df1, df2])
print(concatenated_df)
Output:
A B
0 1 4
1 2 5
2 3 6
0 4 7
1 5 8
2 6 9
In the above example, we created two data frames df1
and df2
with the same columns A
and B
. We then used the concat
method to concatenate the two data frames along the rows. The resulting data frame concatenated_df
will have six rows and two columns.
Appending
Appending is a specific type of concatenation where we add one or more rows to an existing data frame. In Pandas, we can use the append
method to append one or more rows to a data frame. The append
method takes a data frame and appends it to the end of another data frame.
import pandas as pd
# create a data frame
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# create a row to append
row_to_append = pd.DataFrame({'A': [4], 'B': [7]})
# append the row to the data frame
appended_df = df1.append(row_to_append, ignore_index=True)
print(appended_df)
Output:
A B
0 1 4
1 2 5
2 3 6
0 4 7
1 5 8
2 6 9
In the above example, we created a data frame df1
with two columns A
and B
. We then created a new row to append to the data frame df1
. We used the append
method to append the new row to the end of the data frame. The resulting data frame appended_df
will have four rows and two columns.
Differences between Concat and Append
The main difference between concat
and append
is the axis along which they combine data frames. The concat
method can combine data frames along either rows or columns, while the append
method only combines data frames along rows.
Another important difference is that concat
can combine more than two data frames at once, while append
only appends one data frame to another. In addition, the concat
method allows us to specify how to handle missing data, while the append
method only appends data with no options for handling missing data.
When to Use Concat vs Append
Now that we understand the differences between concat
and append
, let’s discuss when to use each method.
We should use the concat
method when we want to combine two or more data frames along either rows or columns. The concat
method is also useful when we want to specify how to handle missing data. For example, we can use the concat
method to concatenate data frames with different columns by specifying how to handle missing data.
We should use the append
method when we want to append one or more rows to an existing data frame. The append
method is useful when we want to add new data to an existing data frame. However, if we need to append multiple data frames, we should use the concat
method instead.
Common Errors and How to Handle Them
Duplicate Index Issues
If duplicate indices are causing problems, reset them using reset_index
or set them uniquely before concatenation.
df1 = df1.reset_index(drop=True)
df2 = df2.reset_index(drop=True)
result = pd.concat([df1, df2], axis=0)
Mismatched Columns
To avoid issues with mismatched columns during concatenation, use the ignore_index
parameter or ensure columns are aligned.
result = pd.concat([df1, df2], axis=1, ignore_index=True)
Ignoring Index
If index alignment is not crucial, use the ignore_index
parameter to reset the index during concatenation.
result = pd.concat([df1, df2], ignore_index=True)
Conclusion
In summary, Pandas offers two methods for combining data frames: concat
and append
. The concat
method concatenates two or more data frames along either rows or columns, while the append
method appends one or more rows to an existing data frame. The main differences between concat
and append
are the axis along which they combine data frames and the ability to handle missing data. We should use the concat
method when we want to combine two or more data frames, while we should use the append
method when we want to append one or more rows to an existing data frame.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.