Pandas DataFrame Applying Functions to All Columns
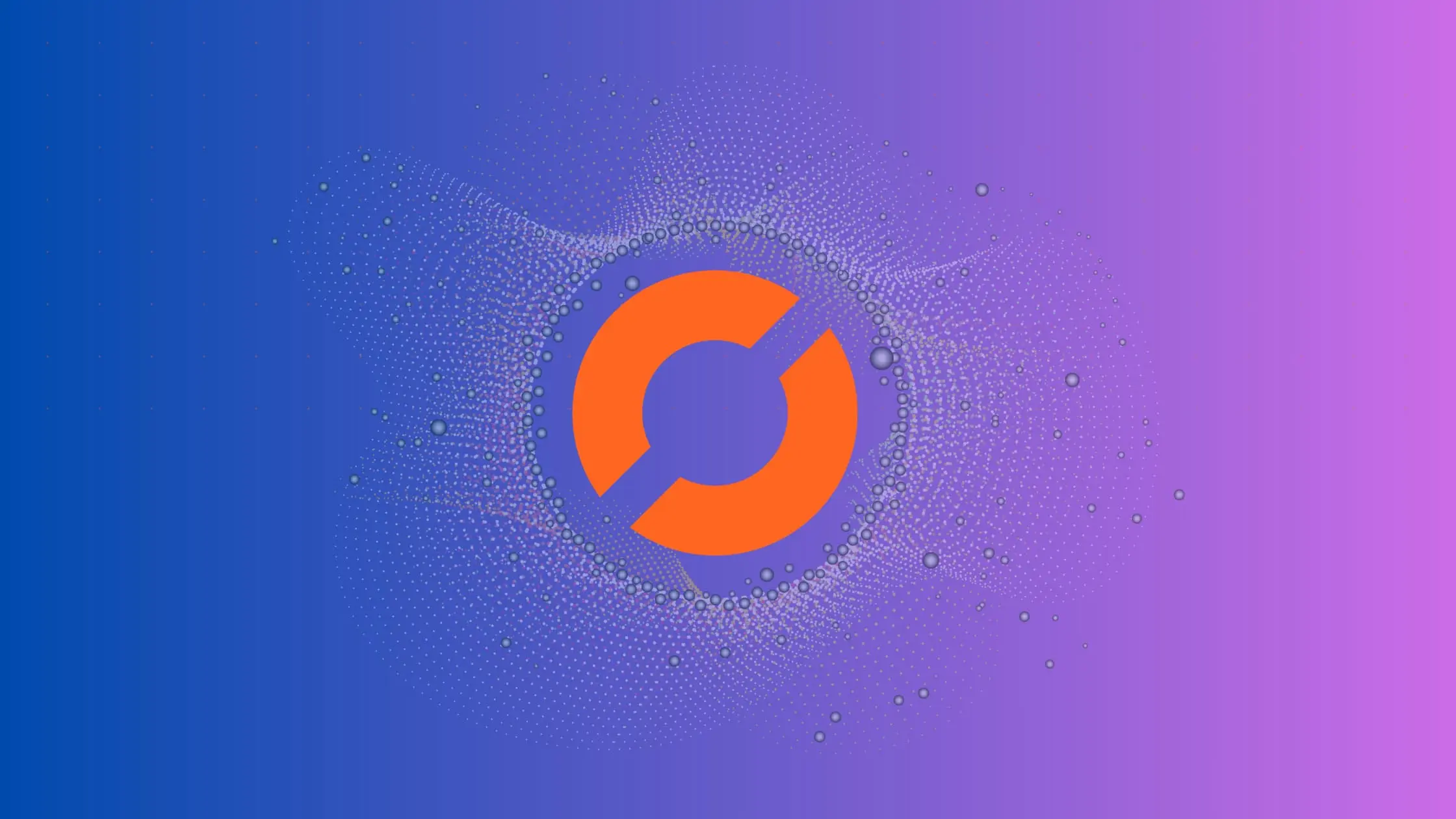
As a data scientist or software engineer working with data, you may often need to apply a function to all columns in a Pandas DataFrame. This can be a time-consuming and tedious task if you try to do it manually. Fortunately, Pandas provides a simple and efficient way to apply functions to all columns in a DataFrame using the apply()
method.
In this blog post, we will explain how to use the apply()
method to apply a function to all columns in a Pandas DataFrame. We will also discuss some common use cases for this method and provide some tips for optimizing its performance.
Table of Contents
- What is the apply() method?
- How to use the apply() method to apply a function to all columns in a DataFrame
- Common use cases for the
apply()
method - Tips for optimizing the performance of the
apply()
method - Conclusion
What is the apply() method?
The apply()
method is a powerful feature of Pandas that allows you to apply a function to each element in a DataFrame. The method takes a single argument: the function you want to apply. You can pass a Python built-in function, a lambda function, or a user-defined function to the apply()
method.
When you apply a function to a DataFrame using the apply()
method, the function is applied to each element in the DataFrame. By default, the apply()
method applies the function to each column in the DataFrame. However, you can use the axis
parameter to apply the function to each row instead.
How to use the apply() method to apply a function to all columns in a DataFrame
Let’s start by creating a simple DataFrame that we can use to demonstrate how to use the apply()
method:
import pandas as pd
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
print(df)
Output:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
This will create a DataFrame with three columns (A
, B
, and C
) and three rows. Now, let’s say we want to apply a function that adds 1 to each element in all columns. We can use the following code:
df_plus=df.apply(lambda x: x + 1)
print(df_plus)
This will apply the lambda function to each column in the DataFrame and return a new DataFrame with the updated values:
A B C
0 2 5 8
1 3 6 9
2 4 7 10
As you can see, the apply()
method has applied the lambda function to each column in the DataFrame and returned a new DataFrame with the updated values.
Common use cases for the apply()
method
The apply()
method is a versatile feature of Pandas that can be used in a wide variety of use cases. Here are some common examples of how you can use the apply()
method to work with DataFrame columns:
Applying a function to a subset of columns
Sometimes, you may want to apply a function to only a subset of columns in a DataFrame. For example, you may want to apply a function that calculates the sum of two columns, but only to a subset of columns. You can use the apply()
method with the subset
parameter to achieve this:
df_ab=df[['A', 'B']].apply(lambda x: x.sum(), axis=1)
print(df_ab)
This will apply the lambda function to only the A
and B
columns in the DataFrame and return a new Series with the sum of the values in each row:
0 5
1 7
2 9
dtype: int64
Applying a function that returns a Series
Sometimes, you may want to apply a function that returns a Series instead of a scalar value. For example, you may want to apply a function that calculates the mean and standard deviation of each column in a DataFrame. You can use the apply()
method with the result_type
parameter to achieve this:
df_series=df.apply(lambda x: pd.Series([x.mean(), x.std()]), result_type='expand')
print(df_series)
This will apply the lambda function to each column in the DataFrame and return a new DataFrame with two columns (0
and 1
) that contain the mean and standard deviation of each column:
A B C
0 2.0 5.0 8.0
1 1.0 1.0 1.0
Applying a user-defined function
Sometimes, you may want to apply a user-defined function to a DataFrame. For example, you may want to apply a function that converts all values in a column to uppercase. You can define a function that does this and then use the apply()
method to apply it to the column:
data = {
'A': ['a', 'b', 'c'],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
def convert_to_uppercase(x):
return x.upper()
df['A']=df['A'].apply(convert_to_uppercase)
print(df)
This will apply the convert_to_uppercase()
function to the A
column in the DataFrame and return a new Series with all values in the column converted to uppercase:
A B C
0 A 4 7
1 B 5 8
2 C 6 9
Tips for optimizing the performance of the apply()
method
The apply()
method can be a powerful tool for working with DataFrame columns, but it can also be slow if used incorrectly. Here are some tips for optimizing the performance of the apply()
method:
Use vectorized functions whenever possible: Vectorized functions, such as those provided by NumPy and Pandas, are much faster than scalar functions. Whenever possible, use vectorized functions instead of scalar functions to improve the performance of the
apply()
method.Avoid using the
apply()
method on large DataFrames: Theapply()
method can be slow on large DataFrames because it applies the function to each element in the DataFrame. If you need to apply a function to a large DataFrame, try to find a vectorized solution instead.Use the
axis
parameter wisely: Theapply()
method can be used to apply a function to each row in a DataFrame by setting theaxis
parameter to 1. However, applying a function to each row can be slower than applying it to each column. Use theaxis
parameter wisely to optimize the performance of theapply()
method.
Conclusion
The apply()
method is a powerful feature of Pandas that allows you to apply a function to each element in a DataFrame. By default, the apply()
method applies the function to each column in the DataFrame, but you can use the axis
parameter to apply the function to each row instead. The apply()
method can be used in a wide variety of use cases, from applying a function to a subset of columns to applying a user-defined function. By following the tips for optimizing the performance of the apply()
method, you can improve the efficiency of your data analysis workflows.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.