Pandas Convert String to Int A Guide for Data Scientists
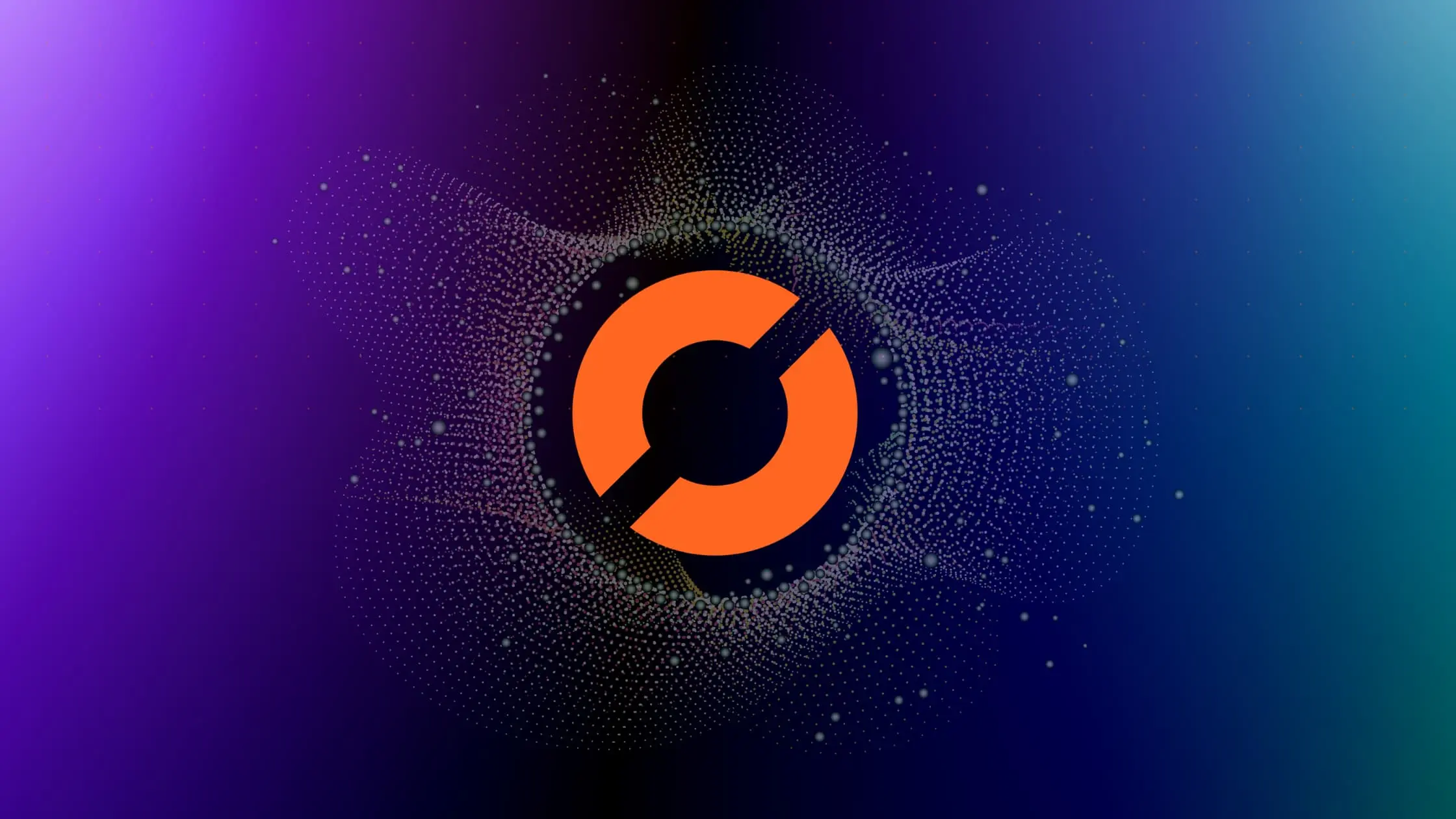
Table of Contents
- Introduction to Pandas
- Converting String to Int using Pandas
- Handling Missing Values
- Handling Non-Numeric Strings
- Conclusion
Introduction to Pandas
Pandas is a popular data manipulation library in Python. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for data cleaning, filtering, and transformation. Pandas is built on top of the NumPy library and is a key tool for data scientists and software engineers working with Python.
Converting String to Int using Pandas
To convert a string to an integer using Pandas, you can use the astype()
method. This method is available on Pandas Series and DataFrame objects and can be used to convert the data type of a column from one type to another.
Let’s start by creating a simple DataFrame that contains a column of strings representing numeric values:
import pandas as pd
df = pd.DataFrame({'numbers': ['1', '2', '3', '4', '5']})
print(df['numbers'].dtype)
Output:
Object
This DataFrame contains a single column called ‘numbers’ with five rows of strings that represent numeric values.
To convert the ‘numbers’ column to integers, we can use the astype()
method as follows:
df['numbers'] = df['numbers'].astype(int)
print(df['numbers'].dtype)
Output:
int32
This code converts the ‘numbers’ column from a string data type to an integer data type.
Handling Missing Values
If the ‘numbers’ column contains missing values, such as NaN, we can use the fillna()
method to fill these values with a default value before converting to integers.
df = pd.DataFrame({'numbers': ['1', '2', '3', '4', 'NaN']})
df['numbers'] = df['numbers'].replace('NaN', pd.NA).fillna(0).astype(int)
print(df['numbers'].dtype)
Output:
int32
In this example, we have used replace
and fillna()
to replace missing values with ‘0’ before converting to integers.
Handling Non-Numeric Strings
If the ‘numbers’ column contains non-numeric strings, such as ‘NaN’ or ‘None’, the astype()
method will raise an error. To handle this, we can use the to_numeric()
method, which can convert strings to numeric values while also handling non-numeric strings.
df = pd.DataFrame({'numbers': ['1', '2', '3', '4', 'None']})
df['numbers'] = pd.to_numeric(df['numbers'], errors='coerce')
print(df)
Output:
numbers
0 1.0
1 2.0
2 3.0
3 4.0
4 NaN
In this example, we have added a ‘None’ value to the ‘numbers’ column. When we try to convert this column to integers using astype()
, we will get a ValueError
. However, if we use to_numeric()
with the errors='coerce'
parameter, non-numeric values will be converted to NaN
values, which can be handled more easily.
Conclusion
In this article, we have explored how to convert string to int using Pandas. We have seen how to handle non-numeric strings and missing values, and we have learned how to use the astype()
and to_numeric()
methods to convert data types. By mastering these techniques, data scientists and software engineers can more effectively manipulate and analyze datasets in Python using Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.