Joining a DataFrame to Another DataFrame Using Pandas Concat
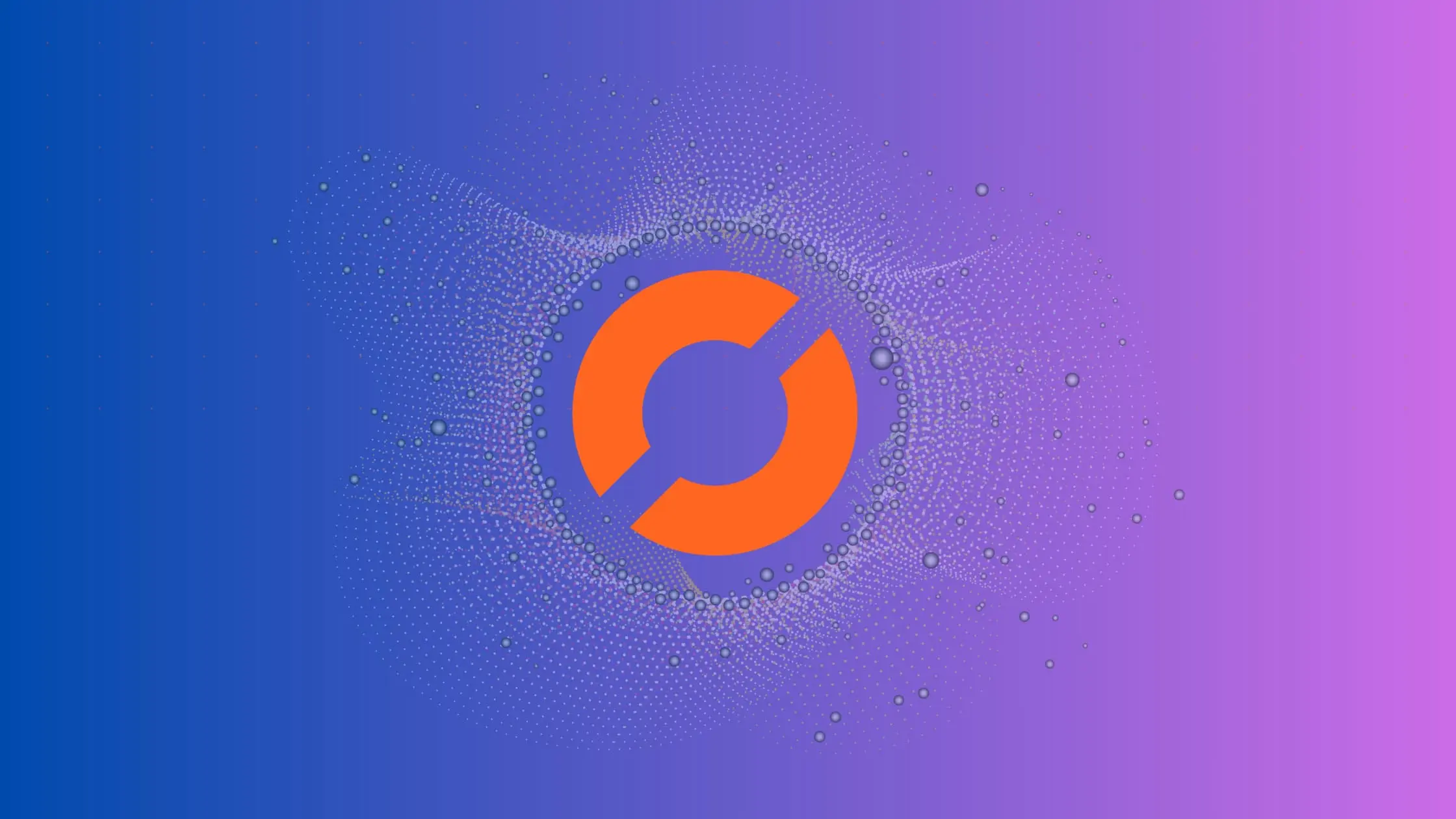
What is Pandas?
Pandas is an open-source data analysis and manipulation library for the Python programming language. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for data analysis, filtering, and visualization.
What is a DataFrame?
A DataFrame is a two-dimensional data structure in Pandas that is used for storing and manipulating tabular data. It is similar to a spreadsheet or a SQL table, where each column can have a different data type, and each row represents a unique record.
How to Join a DataFrame to Another DataFrame
To join one DataFrame to another DataFrame in Pandas, we use the concat()
function. The concat()
function takes two DataFrames as an argument and returns a new DataFrame with the joined data.
The syntax for using the concat()
function is as follows:
new_dataframe = pd.concat([dataframe1, dataframe2])
Here, dataframe1
is the original DataFrame, and dataframe2
is the DataFrame that we want to combine to dataframe1
. The concat()
function returns a new DataFrame, which we store in the variable new_dataframe
.
Let’s take a look at an example. Suppose we have two DataFrames, df1
and df2
, which contain the following data:
import pandas as pd
# create df1
df1 = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
})
# create df2
df2 = pd.DataFrame({
'Name': ['Dave', 'Eve'],
'Age': [40, 45],
'City': ['Houston', 'Miami']
})
We can use the concat()
function to combine df1
and df2
as follows:
# append df2 to df1
new_df = pd.concat([df1, df2])
print(new_df)
This will output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
0 Dave 40 Houston
1 Eve 45 Miami
As you can see, the concat()
function has combined df1
and df2
into a single DataFrame called new_df
. The index values of df2
are preserved in new_df
.
Conclusion
In this article, we have explored how to use the concat()
function in Pandas to combine two data frames into a single data frame. The concat()
function is a powerful tool for data manipulation in Pandas, and is especially useful for combining data frames with different structures or missing data. By following the steps outlined in this article, you can easily combine two data frames in Pandas and streamline your data analysis workflow.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.