Merge and Replace Elements of Two Dataframes Using PySpark
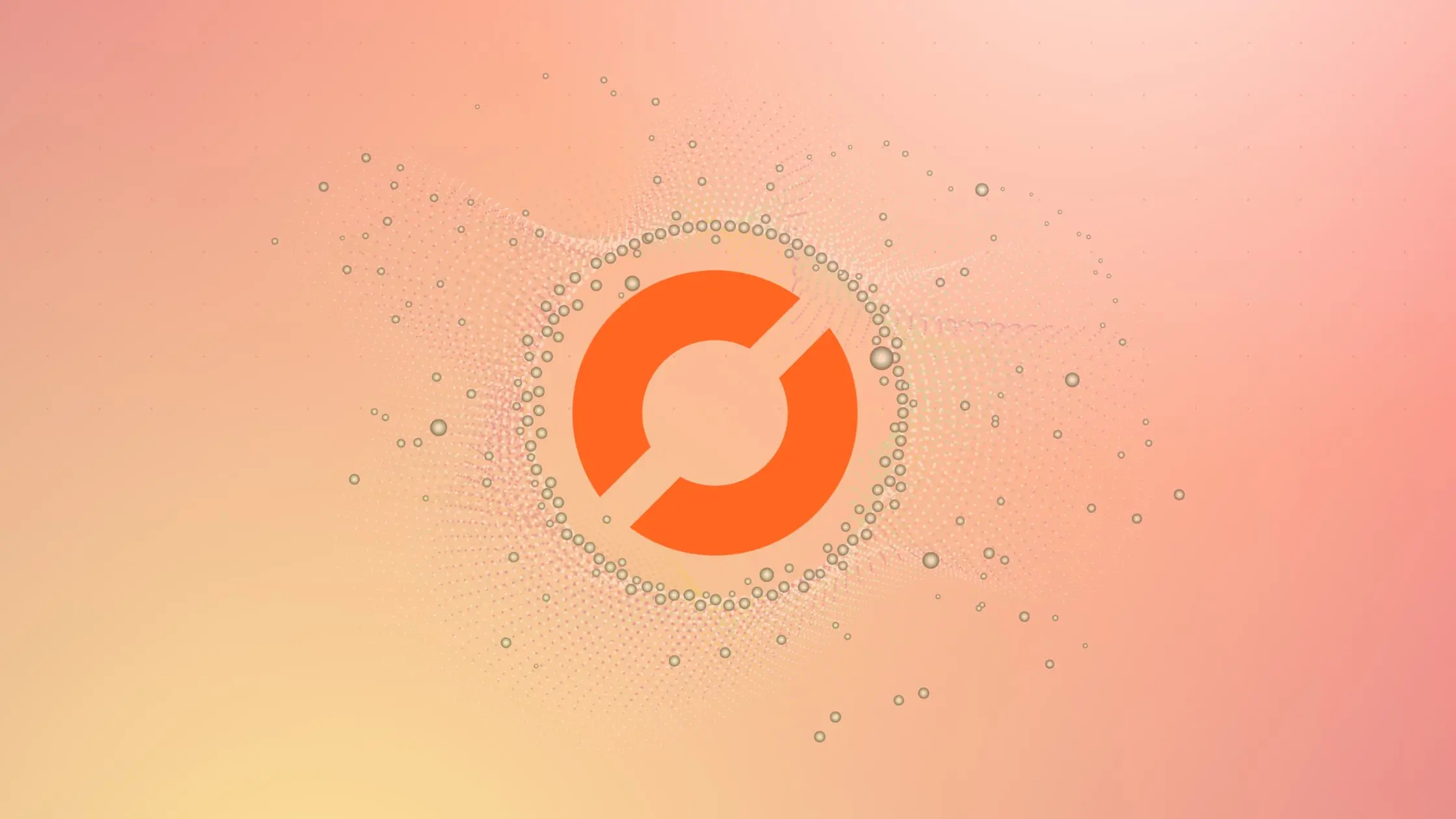
Merge and Replace Elements of Two Dataframes Using PySpark
PySpark, the Python library for Apache Spark, is a powerful tool for large-scale data processing. It’s particularly useful for data scientists who need to handle big data. In this tutorial, we’ll explore how to merge and replace elements of two dataframes using PySpark.
Setting Up Your Environment
Before we dive in, make sure you have PySpark installed. If you haven’t, you can install it using pip:
pip install pyspark
You’ll also need to have a Spark cluster running. If you don’t have one, you can set one up using the instructions in the Spark documentation.
Creating DataFrames
Let’s start by creating two simple dataframes:
from pyspark.sql import SparkSession
from pyspark.sql import Row
# create session
spark = SparkSession.builder.appName("MergeDataframes").getOrCreate()
data1 = [Row(Name='Alice', Age=25, Location='New York'),
Row(Name='Bob', Age=30, Location='Boston'),
Row(Name='Carol', Age=22, Location='Chicago'),
Row(Name='David', Age=28, Location='Los Angeles')]
data2 = [Row(Name='Emily', Age=29, Location='Houston'),
Row(Name='Frank', Age=27, Location='Miami'),
Row(Name='Alice', Age=26, Location='Seattle')]
# create dataframes uisng spark
df1 = spark.createDataFrame(data1)
df2 = spark.createDataFrame(data2)
Merging DataFrames
Merging dataframes in PySpark is done using the union()
function.
# merge 2 dataframes using union function
merged_df = df1.union(df2)
merged_df.show()
Output:
+-----+---+-----------+
| Name|Age| Location|
+-----+---+-----------+
|Alice| 25| New York|
| Bob| 30| Boston|
|Carol| 22| Chicago|
|David| 28|Los Angeles|
|Emily| 29| Houston|
|Frank| 27| Miami|
|Alice| 26| Seattle|
+-----+---+-----------+
This will create a new dataframe that includes all rows where the ‘Name’ column matches in both dataframes.
Replacing Elements
Suppose we aim to substitute the full city names with their respective abbreviations. To achieve this, we can construct a dictionary and apply it in conjunction with the replace
function to exchange the initial city names with their abbreviated forms.
# Create a dictionary containing city's names and its replacements.
diz = {"New York": "NY", "Boston": "BOS", "Chicago": "CHI", "Los Angeles": "LA", "Houston": "HOU", "Miami": "MIA"}
# replace using replace function
replace_df = final_df.na.replace(diz,1,"Location")
replace_df.show()
Output:
+-----+---+--------+
| Name|Age|Location|
+-----+---+--------+
|Alice| 25| NY|
| Bob| 30| BOS|
|Carol| 22| CHI|
|David| 28| LA|
|Emily| 29| HOU|
|Frank| 27| MIA|
+-----+---+--------+
Conclusion
Merging and replacing elements of dataframes are common operations in data processing. PySpark provides efficient and straightforward methods to perform these operations, making it a valuable tool for data scientists working with big data.
PySpark operates in a distributed system, which means it’s designed to process large datasets across multiple nodes. This makes it a powerful tool for handling big data, but it also means you need to be mindful of how you’re structuring your data and operations to get the most out of it.
In this tutorial, we’ve only scratched the surface of what you can do with PySpark. There’s a lot more to explore, including more complex operations and optimizations. So keep experimenting and learning!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.