Loading Images in Google Colab
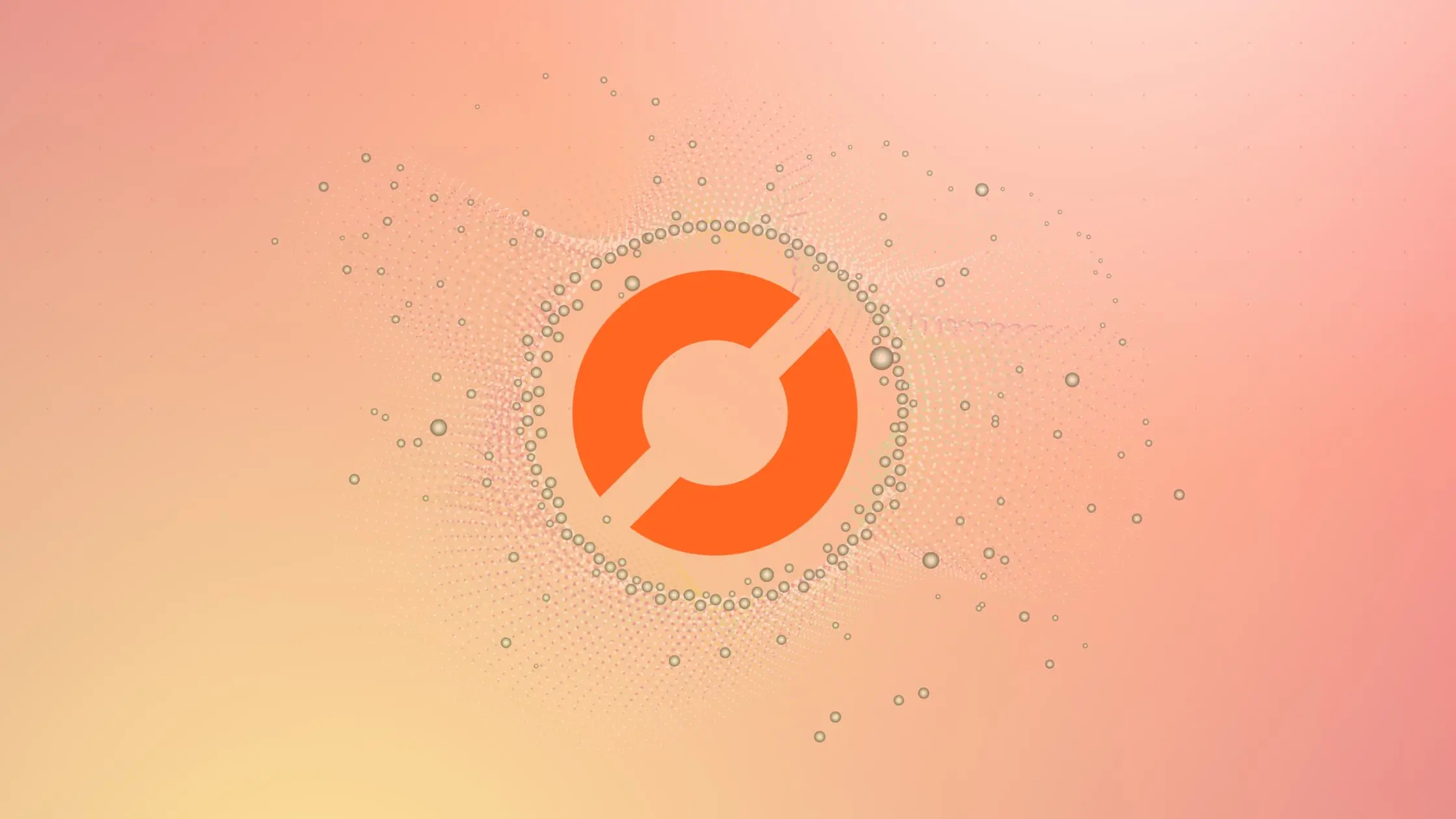
As a software engineer, you may often find yourself working with large datasets that include images. Google Colab is a powerful tool that can be used to create and run Jupyter notebooks, which can be used to analyze and manipulate these datasets. However, loading images into Colab can be a bit tricky, and if not done correctly, can lead to errors and frustration.
In this blog post, we will explore the various ways to load images in Google Colab and discuss best practices for doing so. We will cover the following topics:
- Uploading images to Google Colab
- Loading images using PIL
- Loading images using OpenCV
- Loading images using TensorFlow
Uploading Images to Google Colab
Before we can load images into Colab, we first need to upload our image files to the Colab environment. There are a few ways to do this:
- Upload from local machine: You can upload images from your local machine by clicking on the files icon in the left sidebar and then clicking on the “Upload” button. This will open a file upload dialog where you can select the images to upload.
- Upload from URL: You can also upload images from a URL by using the
wget
command in a code cell. For example, to download an image from the internet, you can use the following command:
!wget https://example.com/image.png -O /content/image.png
This will download the image and save it to the /content
directory in Colab.
Loading Images using PIL
Once we have uploaded our images to Colab, we can load them using the Python Imaging Library (PIL). PIL is a popular Python library for working with images and provides a simple and easy-to-use interface for loading, manipulating, and saving images.
To load an image using PIL, we first need to import the Image
class from the PIL
module. We can then open the image by calling the Image.open()
method and passing in the path to the image file. For example:
from PIL import Image
img = Image.open('/content/image.png')
display(img)
We can then perform various operations on the image, such as resizing, cropping, and rotating.
Loading Images using OpenCV
Another popular Python library for working with images is OpenCV. OpenCV is a powerful computer vision library that provides a wide range of image processing and analysis functions.
To load an image using OpenCV, we first need to import the cv2
module. We can then read the image by calling the cv2.imread()
function and passing in the path to the image file. For example:
import cv2
from matplotlib import pyplot as plt
img = cv2.imread('/content/image.png')
# convert BGR to RGB
img = img[:,:,::-1]
plt.imshow(img)
We can then perform various operations on the image, such as converting it to grayscale, applying filters, and detecting edges.
Loading Images using TensorFlow
If you’re working with image datasets for machine learning tasks, you may want to load your images using TensorFlow. TensorFlow is a popular machine learning library that provides a powerful set of tools for working with images and other types of data.
To load images using TensorFlow, we first need to import the tensorflow
module. We can then use the tf.keras.preprocessing.image
module to load and preprocess our images. For example:
import tensorflow as tf
from tensorflow.keras.preprocessing.image import load_img, img_to_array
img = load_img('/content/image.png')
display(im)
We can then perform various preprocessing steps on the image, such as resizing, normalization, and augmentation.
Best Practices
When loading images in Google Colab, it’s important to follow some best practices to ensure that your code runs smoothly and efficiently. Here are a few tips:
- Use relative paths: Instead of using absolute paths to your image files, use relative paths that are relative to your current working directory. This will make your code more portable and easier to maintain.
- Use the appropriate library: Depending on the task you’re working on, you may want to use different libraries for loading and processing images. For example, if you’re working with machine learning models, you may want to use TensorFlow to load and preprocess your images.
- Preprocess your images: Before using your images for analysis or modeling, it’s important to preprocess them by resizing, normalizing, and augmenting them as needed. This will ensure that your models perform well and that your analysis is accurate.
In conclusion, loading images in Google Colab can be a bit tricky, but by following best practices and using the appropriate libraries, you can load and preprocess your images with ease. Whether you’re working on computer vision projects, machine learning models, or simply analyzing image datasets, Google Colab provides a powerful and flexible environment for working with images.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.