Loading CSV Data into a NumPy Array: A Guide
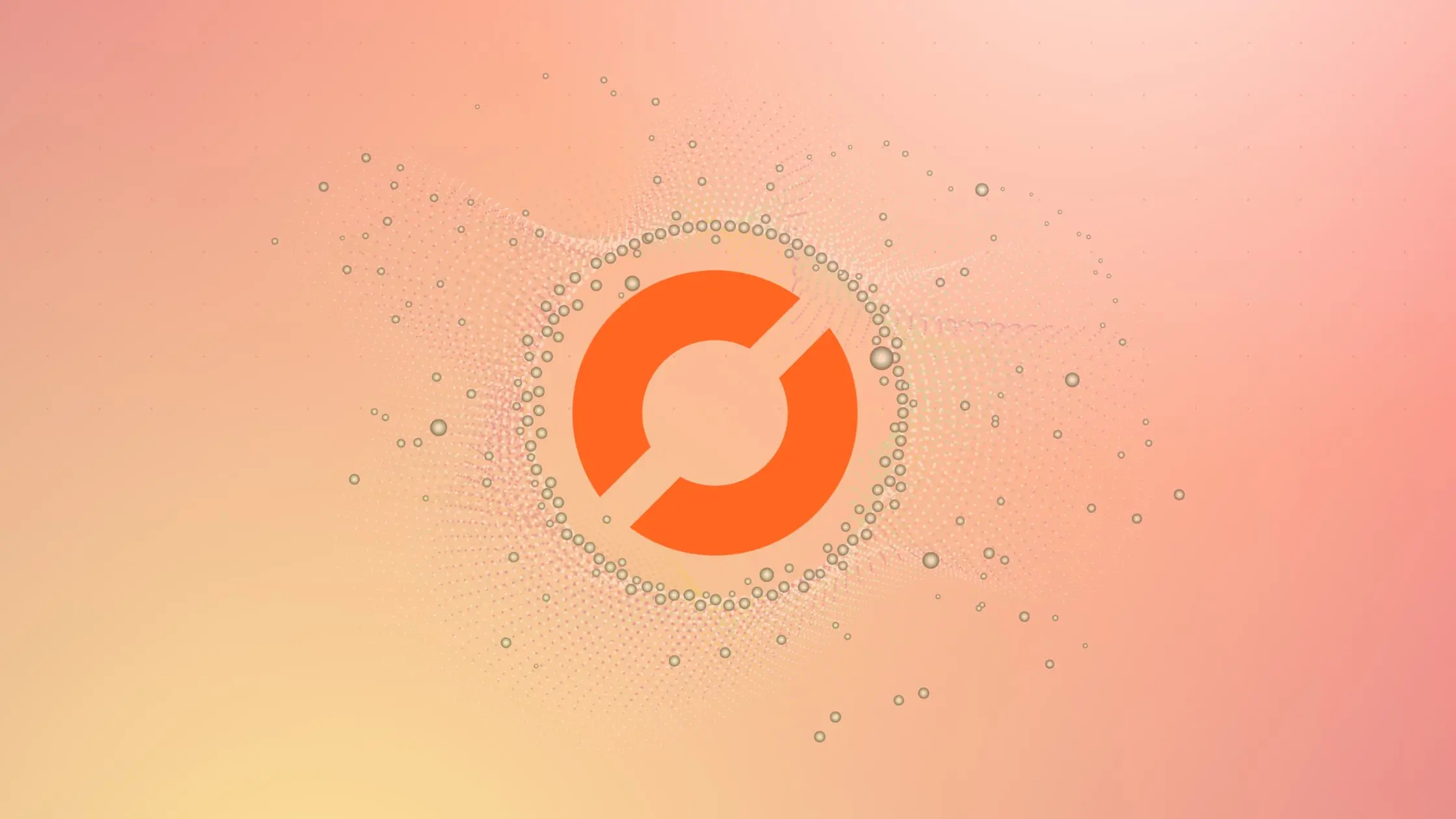
Loading CSV Data into a NumPy Array: A Guide
As data scientists, we often find ourselves dealing with large datasets stored in various formats. One of the most common formats is CSV (Comma Separated Values). In this blog post, we’ll explore how to load data from a CSV file into a NumPy array, a powerful data structure that allows for efficient computation.
Why Use NumPy?
NumPy, short for Numerical Python, is a fundamental package for scientific computing in Python. It provides a high-performance multidimensional array object, and tools for working with these arrays. NumPy arrays are more efficient than Python’s built-in list data structure, making them ideal for handling large datasets and performing mathematical operations.
Step 1: Importing the Necessary Libraries
Before we start, we need to import the necessary libraries. In this case, we’ll need both NumPy and the csv module from Python’s standard library.
import numpy as np
import csv
Step 2: Reading the CSV File
Next, we’ll read the CSV file. We’ll use the csv.reader
function, which returns a reader object that iterates over lines in the specified CSV file.
with open('data.csv', 'r') as f:
reader = csv.reader(f)
data = list(reader)
In this code snippet, 'data.csv'
is the name of our CSV file. Replace this with the path to your own CSV file.
Step 3: Converting the Data to a NumPy Array
Now that we have our data in a Python list, we can convert it to a NumPy array using the np.array
function.
data_array = np.array(data)
However, this will create an array of strings. If your CSV file contains numerical data, you’ll want to convert these strings to the appropriate numerical type. You can do this by specifying the dtype
parameter in the np.array
function.
data_array = np.array(data, dtype=float)
This will create a NumPy array of floats. If your data is integer-based, you can use dtype=int
instead.
Step 4: Manipulating the Data
With our data now in a NumPy array, we can perform a variety of operations on it. For example, we can calculate the mean of the data.
mean = np.mean(data_array)
Or, we can find the maximum value in the array.
max_value = np.max(data_array)
Conclusion
Loading CSV data into a NumPy array is a straightforward process that can be accomplished in just a few lines of code. By using NumPy, we can efficiently manipulate and analyze large datasets, making it an essential tool for any data scientist.
Remember, the key to mastering any programming task is practice. So, try loading different CSV files and performing various operations on the resulting NumPy arrays. Happy coding!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.