Enhancing Data Visualization: Moving Legends Outside the Plot with Matplotlib in Python
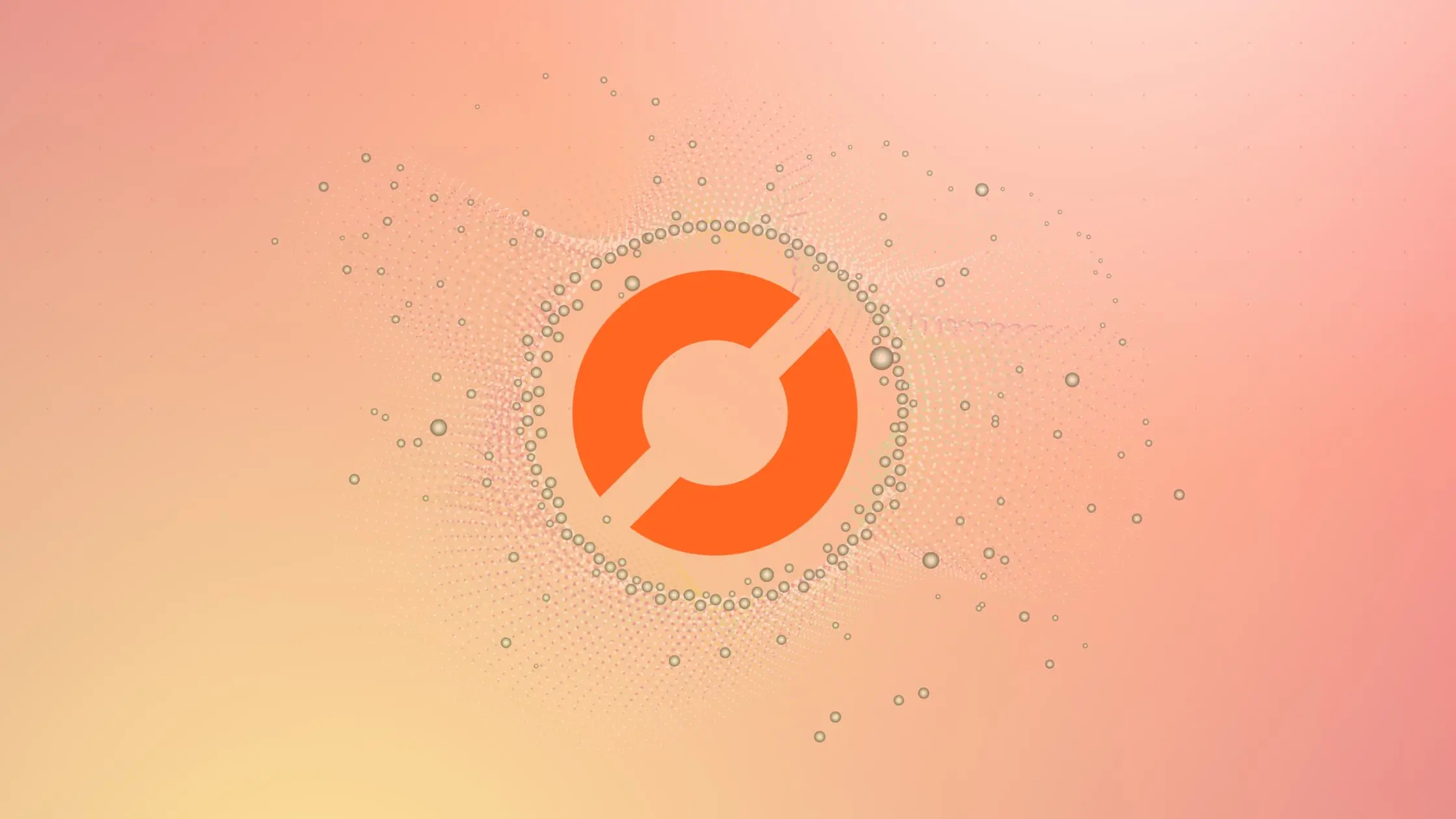
Introduction
When creating plots with numerous data series, the legend can often take up valuable space within the plot itself. This can obscure the data and make the plot difficult to interpret. By placing the legend outside the plot, we can make our visualizations clearer and more effective.
Step 1: Import Necessary Libraries
First, we need to import the necessary libraries. We’ll be using Matplotlib and NumPy for this tutorial.
import matplotlib.pyplot as plt
import numpy as np
Step 2: Create Some Data
Next, we’ll create some data to plot. For simplicity, we’ll generate three sets of random data using NumPy.
np.random.seed(0)
data1 = np.random.randn(100)
data2 = np.random.randn(100) + 1
data3 = np.random.randn(100) + 2
Step 3: Plot the Data
Now, let’s plot the data. We’ll use different colors for each data series and label them accordingly.
plt.plot(data1, color='blue', label='Data 1')
plt.plot(data2, color='red', label='Data 2')
plt.plot(data3, color='green', label='Data 3')
At this point, if we call plt.show()
, we’ll see our three data series plotted with a legend inside the plot.
Step 4: Move the Legend Outside the Plot
To move the legend outside the plot, we’ll use the bbox_to_anchor
and loc
parameters of the legend
function.
plt.legend(bbox_to_anchor=(1.05, 1), loc='upper left')
The bbox_to_anchor
parameter specifies the legend’s position. The tuple (1.05, 1)
positions the legend just outside the plot’s right edge. The loc
parameter determines where the legend’s anchor point should be. ‘upper left’ means the upper left corner of the legend will be at the position specified by bbox_to_anchor
.
Step 5: Adjust the Plot’s Size
Finally, we may need to adjust the plot’s size to ensure the legend doesn’t overlap with it. We can do this using the subplots_adjust
function.
plt.subplots_adjust(right=0.7)
The right
parameter specifies the right side of the subplot’s position in the figure. By setting it to 0.7, we leave enough space for the legend on the right.
Conclusion
And that’s it! With just a few lines of code, we’ve moved the legend outside the plot, making our data visualization clearer and easier to interpret.
Remember, effective data visualization is not just about presenting data, but presenting it in a way that is easy to understand. By mastering tools like Matplotlib, you can ensure your visualizations are as effective as possible.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.