Joining DataFrames in PySpark Without Duplicate Columns
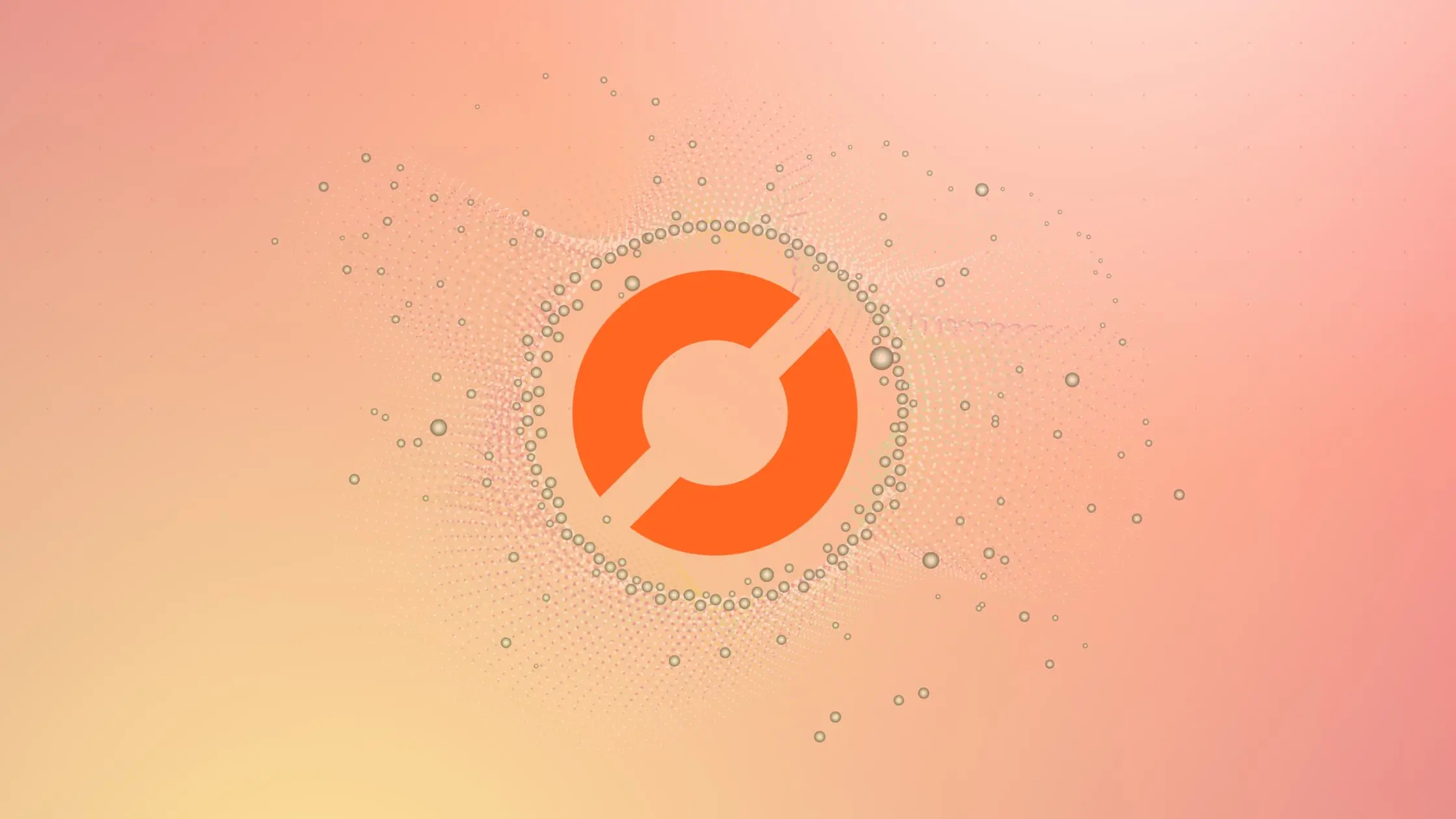
In the world of big data, PySpark has emerged as a powerful tool for processing and analyzing large datasets. One common operation in PySpark is joining two DataFrames. However, this operation can often result in duplicate columns, which can be problematic. In this blog post, we’ll explore how to perform a join in PySpark without creating duplicate columns.
Table of Contents
- What is PySpark?
- The Problem with Duplicate Columns
- The Solution: Drop Duplicate Columns After Join
- Conclusion
What is PySpark?
PySpark is the Python library for Apache Spark, an open-source, distributed computing system used for big data processing and analytics. PySpark allows data scientists to write Spark applications using Python, without the need to know Scala or Java.
The Problem with Duplicate Columns
When joining two DataFrames in PySpark, it’s common to end up with duplicate columns. This happens when the DataFrames have columns with the same name. This can be problematic because it can lead to confusion and errors in subsequent operations.
For example, consider two DataFrames, df1
and df2
, with the following data:
from pyspark.sql import SparkSession
# create a Spark Session
spark = SparkSession.builder.appName("MergeDataframes").getOrCreate()
# create dataframes
df1 = spark.createDataFrame([(1, "John"), (2, "Mike")], ["id", "name"])
df2 = spark.createDataFrame([(1, "New York"), (2, "Los Angeles")], ["id", "city"])
If we join these DataFrames on the id
column, we’ll end up with two id
columns:
joined_df = df1.join(df2, df1.id == df2.id)
joined_df.show()
The output will be:
+---+----+---+-----------+
| id|name| id| city|
+---+----+---+-----------+
| 1|John| 1| New York|
| 2|Mike| 2|Los Angeles|
+---+----+---+-----------+
As you can see, we have two id
columns, which can be confusing.
The Solution: Drop Duplicate Columns After Join
The solution to this problem is to drop the duplicate columns after the join operation. PySpark provides the drop()
function for this purpose. Here’s how you can use it:
joined_df = df1.join(df2, df1.id == df2.id).drop(df2.id)
joined_df.show()
The output will be:
+---+----+-----------+
| id|name| city|
+---+----+-----------+
| 1|John| New York|
| 2|Mike|Los Angeles|
+---+----+-----------+
As you can see, we now have only one id
column.
Conclusion
Joining DataFrames is a common operation in PySpark, but it can often result in duplicate columns. By using the drop()
function, you can easily remove these duplicates and keep your data clean and understandable. This technique is essential for data scientists working with large datasets in PySpark.
Remember, clean and well-structured data is the foundation of any successful data science project. So, always ensure your data is as clean and well-structured as possible before performing any analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.