How to Use Python and Pandas to Convert XLSX to CSV and Remove the Index Column
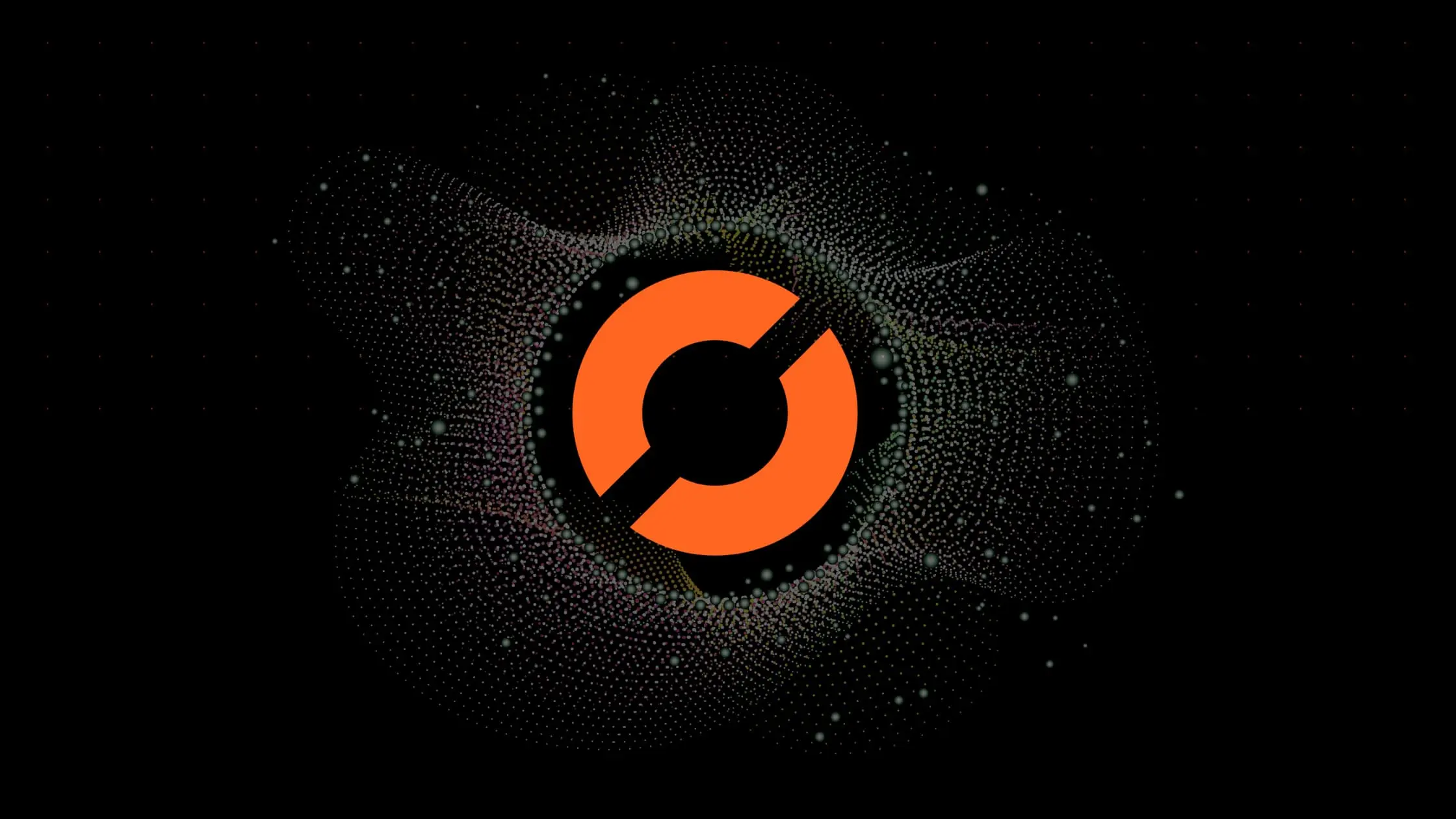
As a data scientist or software engineer, you may find yourself needing to convert an XLSX file to a CSV file for analysis or to feed into another program. Python and Pandas make this task easy and efficient. In this tutorial, we will walk through the steps to convert an XLSX file to a CSV file using Pandas and then remove the index column.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.
What is Pandas?
Pandas is a popular Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating data, and tools for reading and writing data in a variety of formats, including CSV, Excel, SQL databases, and more.
How to Convert XLSX to CSV using Pandas
To convert an XLSX file to a CSV file using Pandas, we will use the read_excel()
and to_csv()
functions.
Excel Sample Data:
First, we will import the necessary libraries:
import pandas as pd
Next, we will read in the XLSX file using the read_excel()
function. We will specify the file path, sheet name (if applicable), and any other relevant parameters. For example:
df = pd.read_excel('path/to/file.xlsx', sheet_name='Sheet1', header=0)
This will create a Pandas DataFrame object containing the data from the XLSX file.
Output:
Name Age Occupation
0 John 25 Engineer
1 Jane 30 Data Scientist
2 Bob 28 Analyst
3 Alice 22 Developer
Next, we will use the to_csv()
function to write the DataFrame to a CSV file. We will specify the file path and any other relevant parameters. For example:
df.to_csv('path/to/file.csv', index=False)
This will write the DataFrame to a CSV file without the index column.
Output:
Name,Age,Occupation
John,25,Engineer
Jane,30,Data Scientist
Bob,28,Analyst
Alice,22,Developer
How to Remove the Index Column
By default, when you write a Pandas DataFrame to a CSV file using the to_csv()
function, it includes the index column. However, you may not always want this column included in your output file. To remove the index column, you can simply set the index
parameter to False
, as shown above.
If you have already written your DataFrame to a CSV file and want to remove the index column, you can do so using the following steps:
- Read in the CSV file using Pandas and specify the index column (which should be the first column in the file) as the index:
df = pd.read_csv('path/to/file.csv', index_col=0)
- Write the DataFrame back to the CSV file without the index column:
df.to_csv('path/to/file.csv', index=False)
This will overwrite the original CSV file with a new file that does not include the index column.
Output:
Name,Age,Occupation
John,25,Engineer
Jane,30,Data Scientist
Bob,28,Analyst
Alice,22,Developer
Conclusion
Converting an XLSX file to a CSV file using Python and Pandas is a simple and efficient process. The read_excel()
and to_csv()
functions make it easy to read in and write out data in a variety of formats, and the index
parameter makes it easy to remove the index column from your output file. By following the steps outlined in this tutorial, you should be able to quickly convert XLSX files to CSV files and remove the index column as needed.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.