How to Use Pandas to Subtract DataFrames
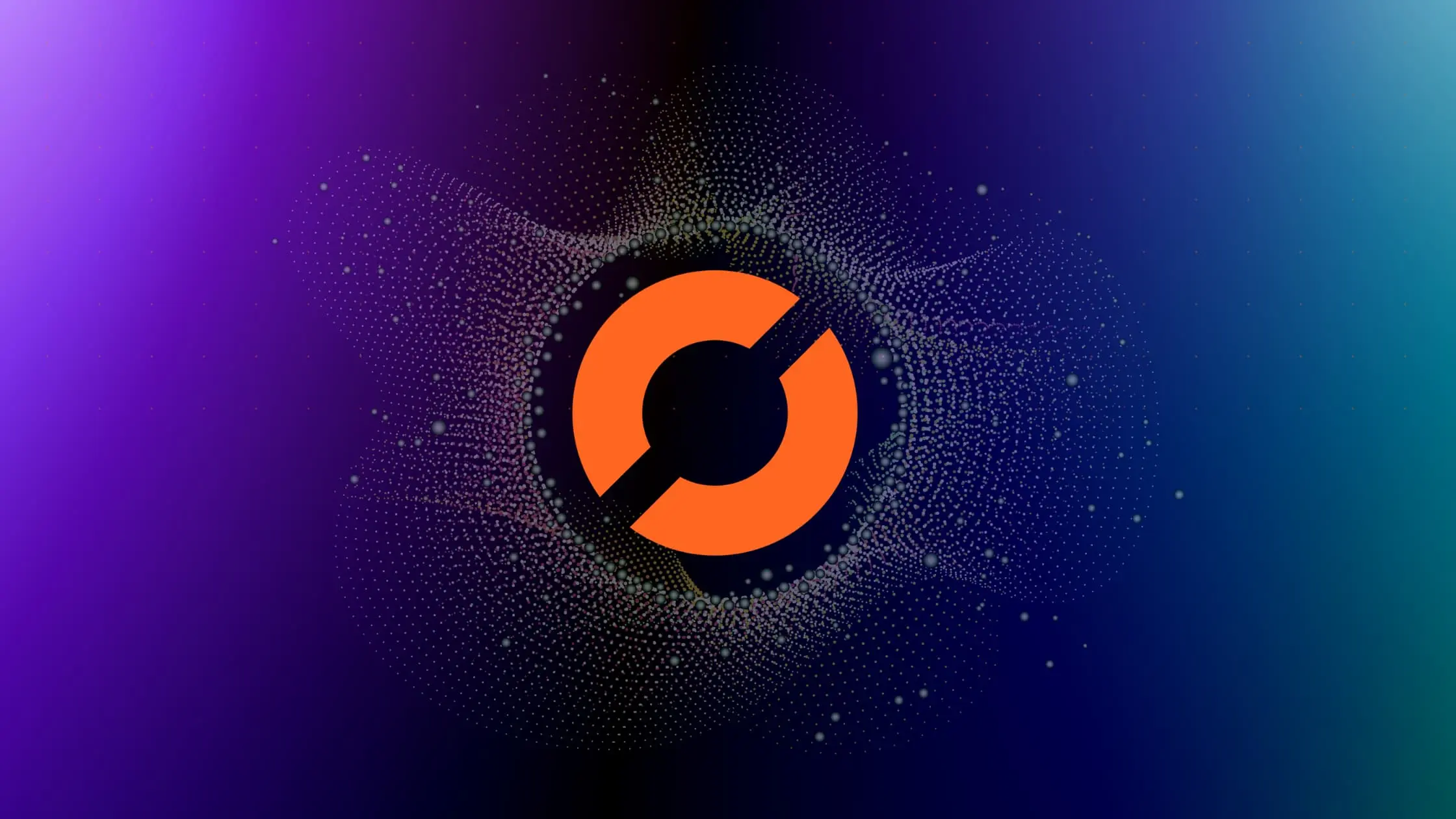
As a data scientist or software engineer, you are likely to encounter situations where you need to perform mathematical operations on data. One such operation is subtraction, and Pandas provides a robust set of tools that make it easy to subtract DataFrames.
In this tutorial, we’ll explore how to subtract DataFrames using Pandas. We’ll start with a brief overview of Pandas, then move on to the different ways you can subtract DataFrames.
Table of Contents
What Is Pandas?
Pandas is a popular data manipulation library for Python. It provides a powerful set of tools for working with structured data, including DataFrames, which are two-dimensional arrays with labeled axes (rows and columns).
Pandas provides a wide range of functions and methods for manipulating DataFrames, including mathematical operations like addition, subtraction, multiplication, and division.
How to Subtract DataFrames with Pandas
Subtracting DataFrames in Pandas is straightforward. There are several ways to do it, depending on your specific needs. Here are the most common methods:
Method 1: Using the - Operator
The simplest way to subtract two DataFrames is to use the -
operator. This operator subtracts the values in the second DataFrame from the values in the first DataFrame, element-wise.
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [1, 2, 3]})
df3 = df1 - df2
print(df3)
In this example, we create two DataFrames, df1
and df2
, with the same shape. We then subtract df2
from df1
using the -
operator and store the result in a new DataFrame, df3
.
The output of this program is:
A B
0 0 3
1 0 3
2 0 3
As you can see, the values in df2
have been subtracted from the values in df1
, element-wise.
Method 2: Using the .sub() Method
Another way to subtract two DataFrames is to use the .sub()
method. This method works similarly to the -
operator but provides more flexibility.
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [1, 2, 3]})
df3 = df1.sub(df2)
print(df3)
In this example, we create two DataFrames, df1
and df2
, with the same shape. We then subtract df2
from df1
using the .sub()
method and store the result in a new DataFrame, df3
.
The output of this program is:
A B
0 0 3
1 0 3
2 0 3
As you can see, the result is the same as in the previous example.
The .sub()
method also provides optional arguments that allow you to control how the subtraction is performed. For example, you can specify a fill value to use for missing values, or you can specify how to handle the axis labels.
Method 3: Using the .subtract() Method
Finally, you can also subtract two DataFrames using the .subtract()
method. This method is similar to the .sub()
method but provides even more flexibility.
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [1, 2, 3]})
df3 = df1.subtract(df2)
print(df3)
In this example, we create two DataFrames, df1
and df2
, with the same shape. We then subtract df2
from df1
using the .subtract()
method and store the result in a new DataFrame, df3
.
The output of this program is:
A B
0 0 3
1 0 3
2 0 3
As you can see, the result is once again the same as in the previous examples.
The .subtract()
method provides even more optional arguments than the .sub()
method, allowing you to fine-tune the subtraction process even further.
Conclusion
Subtracting DataFrames in Pandas is a simple process that can be done in several ways. The -
operator, .sub()
, and .subtract()
methods all provide different levels of flexibility and control over the subtraction process.
By mastering these methods, you can easily handle situations where you need to subtract two DataFrames in your data analysis projects. With Pandas, you have a powerful tool at your disposal that can help you perform a wide range of mathematical operations on your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.