How to Use Pandas loc with Multiple Conditions
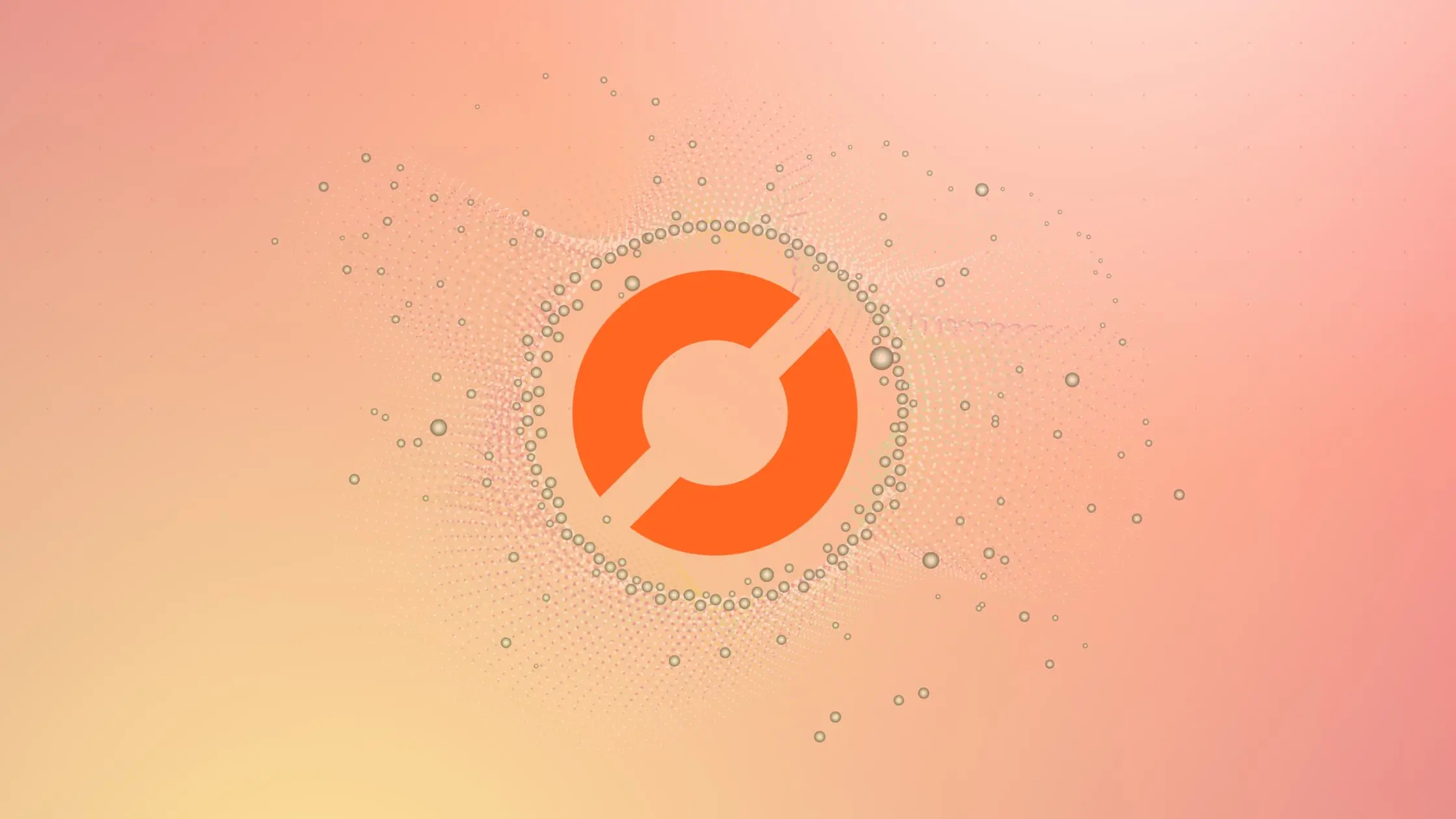
As a data scientist or software engineer, you may often need to filter and manipulate data based on multiple conditions. Pandas, a popular Python library for data analysis, offers a powerful method called .loc
that allows you to select rows and columns based on labels or boolean conditions. In this blog post, we will explore how to use Pandas loc with multiple conditions to filter and manipulate data efficiently.
Table of Contents
What is Pandas loc?
Pandas loc is a method that allows you to select rows and columns from a DataFrame based on labels or boolean conditions. Loc stands for location-based indexing, which means that you can select data based on its position within the DataFrame. The basic syntax for using loc is as follows:
df.loc[row_label, column_label]
Here, df
is the DataFrame, row_label
is the label or boolean condition for the rows, and column_label
is the label or boolean condition for the columns. You can use loc to select data based on the following types of labels or conditions:
- A single label or list of labels for rows or columns
- A boolean condition for rows or columns
- A function that returns a boolean condition for rows or columns
Using Pandas loc with Multiple Conditions
One of the most powerful features of Pandas loc is the ability to select data based on multiple conditions. This can be achieved by combining boolean conditions using logical operators such as &
(and), |
(or), and ~
(not). Let’s explore some examples of how to use Pandas loc with multiple conditions.
Example 1: Selecting Rows Based on Multiple Conditions
Suppose we have a DataFrame df
with the following data:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Age': [25, 30, 35, 40, 45],
'Gender': ['Female', 'Male', 'Male', 'Male', 'Female'],
'Salary': [50000, 60000, 70000, 80000, 90000]}
df = pd.DataFrame(data)
To select rows based on multiple conditions, we can combine boolean conditions using the &
operator. For example, to select all rows where the age is greater than 30 and the gender is male, we can do the following:
df.loc[(df['Age'] > 30) & (df['Gender'] == 'Male')]
This will give us the following output:
Name Age Gender Salary
2 Charlie 35 Male 70000
3 David 40 Male 80000
Note that we used parentheses to group the boolean conditions, which is necessary when using multiple conditions.
Example 2: Updating Values Based on Multiple Conditions
In addition to selecting rows based on multiple conditions, we can also update values in the DataFrame based on multiple conditions. For example, suppose we want to increase the salary of all female employees who are over 35 years old. We can do the following:
df.loc[(df['Age'] > 35) & (df['Gender'] == 'Female'), 'Salary'] += 10000
This will increase the salary of Emily by 10000, as she is the only female employee who meets the conditions.
Example 3: Using Functions to Define Conditions
In addition to using boolean conditions directly, we can also use functions to define conditions for Pandas loc. For example, suppose we want to select rows where the name starts with the letter ‘A’ and the salary is greater than 45000. We can define a function starts_with_a
as follows:
def starts_with_a(name):
return name.startswith('A')
Then we can use this function to define the row condition as follows:
df.loc[(df['Name'].apply(starts_with_a)) & (df['Salary'] > 45000)]
This will give us the following output:
Name Age Gender Salary
0 Alice 25 Female 50000
Pros
- Flexibility: Pandas loc provides a flexible and powerful way to filter and manipulate data based on various conditions, enabling data scientists and software engineers to perform complex data manipulations with ease.
- Readability: The syntax for using Pandas loc is intuitive, making the code readable and easy to understand. This is crucial for collaboration and maintenance of code.
- Efficiency: Pandas loc is optimized for performance, and when used correctly, it can efficiently handle large datasets, making it suitable for data analysis tasks.
- Consistency: The location-based indexing philosophy of Pandas loc is consistent with the overall design of the library, ensuring a consistent approach to data selection and manipulation.
Cons
- Memory Usage: Depending on the complexity of conditions and the size of the DataFrame, using multiple conditions with Pandas loc may lead to increased memory usage. Users should be mindful of memory constraints, especially when working with large datasets.
- Learning Curve: While the basic usage of Pandas loc is straightforward, mastering the handling of multiple conditions and logical operators may have a learning curve for beginners.
- Potential for Errors: Writing complex conditions using logical operators increases the likelihood of errors. Parentheses must be used correctly to group conditions, and logical operators need to be applied in the right order.
Error Handling
- Parentheses Misuse: Ensure that parentheses are correctly used to group boolean conditions when combining them with logical operators. Incorrect usage can lead to unexpected results or errors.
- Logical Operator Precedence: Be aware of the precedence of logical operators (
&
,|
,~
) to avoid unintended behavior. When in doubt, use parentheses to explicitly define the order of evaluation. - Data Type Mismatch: Check for data type compatibility when applying conditions. Mismatched data types may result in unexpected behavior or errors.
- Empty Results: Be prepared for the possibility of empty results if the combination of conditions does not match any rows in the DataFrame. Check for empty results and handle them appropriately in your code.
- Performance Considerations: Keep in mind the performance implications of using multiple conditions, especially with large datasets. Test the code with smaller subsets of data to ensure it performs efficiently before applying it to the entire dataset.
Conclusion
In this blog post, we have explored how to use Pandas loc with multiple conditions to filter and manipulate data efficiently. We have seen how to select rows based on multiple conditions using boolean operators, how to update values based on multiple conditions, and how to use functions to define conditions. By mastering these techniques, you can perform complex data manipulations with ease and efficiency.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.