How to Use k-fold Cross Validation with DataLoaders in PyTorch
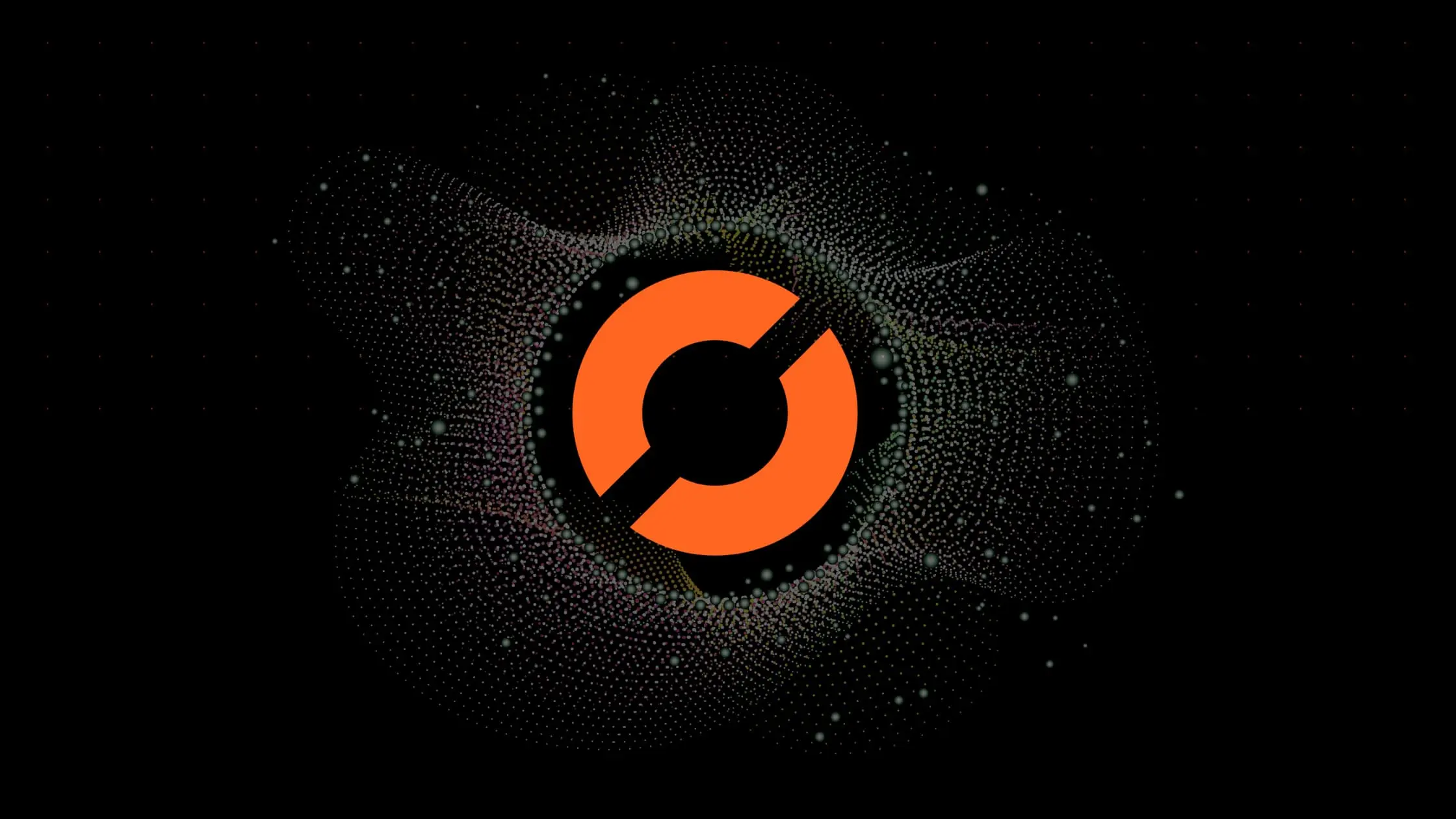
As a data scientist or software engineer working with deep learning models, it’s important to ensure that your models are performing well and are trained on high-quality data. One way to achieve this is by using k-fold cross validation, a technique that helps evaluate the performance of your model on a variety of data subsets. In this article, we’ll explain what k-fold cross validation is, how it works, and how to implement it using DataLoaders in PyTorch.
Table of Contents
- Introduction
- What is k-fold Cross Validation?
- How Does k-fold Cross Validation Work?
- Implementing k-fold Cross Validation using DataLoaders in PyTorch
- Pros and Cons of k-Fold Cross Validation in PyTorch
- Conclusion
What is k-fold Cross Validation?
K-fold cross validation is a technique used to evaluate the performance of machine learning models. It involves splitting the dataset into k equal-sized partitions or folds, where k is a positive integer. The model is then trained on k-1 folds and tested on the remaining fold, with this process repeated k times. The performance of the model is evaluated by averaging the results of these k iterations.
The advantage of using k-fold cross validation is that it allows for a more robust evaluation of the model’s performance. By training and testing the model on different subsets of the data, we can get a better idea of how the model would perform on new, unseen data. Additionally, it helps to ensure that the model is not overfitting to a particular subset of the data.
How Does k-fold Cross Validation Work?
The k-fold cross validation process can be broken down into the following steps:
- The dataset is divided into k equal-sized partitions or folds.
- For each fold i, the model is trained on the remaining k-1 folds.
- The model is tested on the fold i.
- Steps 2 and 3 are repeated for each fold i, with the performance of the model evaluated by averaging the results of these k iterations.
Implementing k-fold Cross Validation using DataLoaders in PyTorch
Now that we have a better understanding of what k-fold cross validation is and how it works, let’s take a look at how we can implement it using DataLoaders in PyTorch.
Step 1: Importing the Required Libraries
We’ll start by importing the required libraries, including PyTorch and scikit-learn.
import torch
from torch.utils.data import DataLoader
from sklearn.model_selection import KFold
Step 2: Defining the Dataset and Transformations
Next, we’ll define our dataset and any necessary transformations. For this example, we’ll use the MNIST dataset as an example.
from torchvision.datasets import MNIST
from torchvision.transforms import ToTensor
# Define the dataset and transformations
train_dataset = MNIST(root="data", train=True, download=True, transform=ToTensor())
Step 3: Defining the Model and Training Function
We’ll also need to define our model and the training function, which will be used to train the model on each fold.
import torch.nn as nn
import torch.optim as optim
# Define the model architecture
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(1, 32, kernel_size=3)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3)
self.fc1 = nn.Linear(64, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = nn.functional.relu(self.conv1(x))
x = nn.functional.relu(self.conv2(x))
# Use adaptive pooling to dynamically reshape the tensor
x = nn.functional.adaptive_avg_pool2d(x, (1, 1))
# Flatten the tensor before passing it to the fully connected layers
x = x.view(x.size(0), -1)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return nn.functional.log_softmax(x, dim=1)
# Define the training function
def train(model, device, train_loader, optimizer, epoch):
model.train()
for batch_idx, (data, target) in enumerate(train_loader):
data, target = data.to(device), target.to(device)
optimizer.zero_grad()
output = model(data)
loss = nn.functional.nll_loss(output, target)
loss.backward()
optimizer.step()
Step 4: Implementing k-fold Cross Validation
Lastly, we’ll implement k-fold cross validation using DataLoaders in PyTorch.
# Define the number of folds and batch size
k_folds = 5
batch_size = 64
# Define the device (CPU or GPU)
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# Initialize the k-fold cross validation
kf = KFold(n_splits=k_folds, shuffle=True)
# Loop through each fold
for fold, (train_idx, test_idx) in enumerate(kf.split(train_dataset)):
print(f"Fold {fold + 1}")
print("-------")
# Define the data loaders for the current fold
train_loader = DataLoader(
dataset=train_dataset,
batch_size=batch_size,
sampler=torch.utils.data.SubsetRandomSampler(train_idx),
)
test_loader = DataLoader(
dataset=train_dataset,
batch_size=batch_size,
sampler=torch.utils.data.SubsetRandomSampler(test_idx),
)
# Initialize the model and optimizer
model = Net().to(device)
optimizer = optim.SGD(model.parameters(), lr=0.01, momentum=0.5)
# Train the model on the current fold
for epoch in range(1, 11):
train(model, device, train_loader, optimizer, epoch)
# Evaluate the model on the test set
model.eval()
test_loss = 0
correct = 0
with torch.no_grad():
for data, target in test_loader:
data, target = data.to(device), target.to(device)
output = model(data)
test_loss += nn.functional.nll_loss(output, target, reduction="sum").item()
pred = output.argmax(dim=1, keepdim=True)
correct += pred.eq(target.view_as(pred)).sum().item()
test_loss /= len(test_loader.dataset)
accuracy = 100.0 * correct / len(test_loader.dataset)
# Print the results for the current fold
print(f"Test set: Average loss: {test_loss:.4f}, Accuracy: {correct}/{len(test_loader.dataset)} ({accuracy:.2f}%)\n")
Output example:
Fold 1
-------
Test set: Average loss: 0.3416, Accuracy: 4132/60000 (6.89%)
Fold 2
-------
Test set: Average loss: 0.3323, Accuracy: 4413/60000 (7.36%)
Fold 3
-------
Test set: Average loss: 0.3596, Accuracy: 3669/60000 (6.12%)
Fold 4
-------
Test set: Average loss: 0.3420, Accuracy: 3871/60000 (6.45%)
Fold 5
-------
Test set: Average loss: 0.3399, Accuracy: 4135/60000 (6.89%)
This code will perform k-fold cross validation on the MNIST dataset using DataLoaders in PyTorch. The results for each fold will be printed to the console, including the test set accuracy and loss.
Pros and Cons of k-Fold Cross Validation in PyTorch
Pros:
Comprehensive Model Evaluation: K-fold cross validation provides a comprehensive evaluation of the model by testing it on different subsets of the data. This helps in assessing how well the model generalizes to unseen data, making the evaluation more robust.
Reduces Overfitting Risk: By training and testing the model on different subsets in each iteration, the technique helps in preventing overfitting. It ensures that the model does not overly specialize to a particular subset of the data, promoting better generalization.
Improved Confidence in Model Performance: Averaging the results over multiple folds reduces the impact of random variability in a single train-test split, providing a more reliable estimate of the model’s performance.
Guidance on Model Stability: Identifying variations in performance across folds can give insights into the stability of the model. Consistent performance across folds indicates a stable and reliable model.
Applicability to Various Datasets: K-fold cross validation is versatile and can be applied to different datasets, making it a widely used technique for model evaluation.
Cons:
Computational Cost: Performing k-fold cross validation requires training and evaluating the model multiple times, increasing computational cost compared to a single train-test split.
Time-Consuming: The technique involves a repetitive process of training and testing k times, making it time-consuming, especially for large datasets and complex models.
Data Shuffling Dependency: The performance of k-fold cross validation can be sensitive to the initial shuffling of the data. If the data is not shuffled properly, it might lead to biased results.
Conclusion
In this article, we’ve explained what k-fold cross validation is and how it can be used to evaluate the performance of machine learning models. We’ve also shown how to implement k-fold cross validation using DataLoaders in PyTorch, providing a step-by-step guide to help you get started with this technique. By using k-fold cross validation, you can ensure that your models are performing well on a variety of data subsets, helping to improve the overall quality and accuracy of your models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.