How to update values in a specific row in a Python Pandas DataFrame
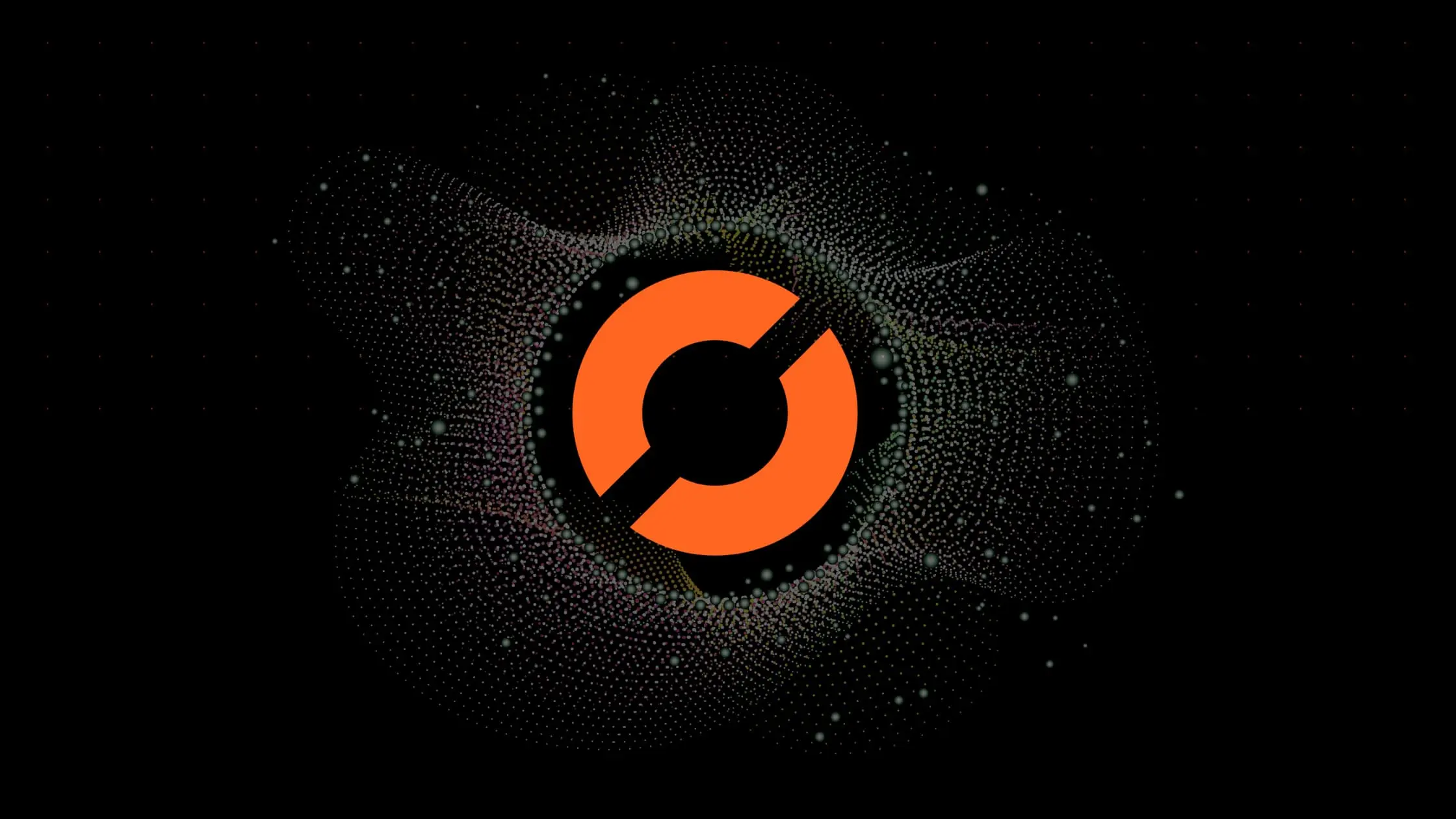
As a data scientist or software engineer, you will often find yourself working with data in Python Pandas DataFrames. These data structures are incredibly useful for manipulating and analyzing data, but sometimes you will need to update the values in a specific row. In this article, we will explore different ways to update values in a specific row in a Pandas DataFrame.
Table of Contents
- Introduction
- Updating a single value in a row
- Updating multiple values in a row
- Updating values based on a condition
- Conclusion
Updating a single value in a row
Let’s start with the simplest case: updating a single value in a specific row. To do this, we first need to select the row we want to update. We can use the .loc
method to select the row by label, or the .iloc
method to select the row by integer index. Once we have selected the row, we can update the value in the desired column using the =
operator.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# update the value in the second row, second column
df.loc[1, 'B'] = 10
print(df)
This will output:
A B
0 1 4
1 2 10
2 3 6
Updating multiple values in a row
If we need to update multiple values in the same row, we can use the .loc
or .iloc
methods to select the row as before, but this time we will pass a dictionary of column names and values to the .loc
or .iloc
methods. The keys of the dictionary should correspond to the column names, and the values should be the new values we want to set.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# update the values in the second row
df.loc[1] = {'A': 10, 'B': 20}
print(df)
This will output:
A B
0 1 4
1 10 20
2 3 6
Note that if we use .loc
to select the row, we need to pass a dictionary to update all the columns in the row. If we only want to update some of the columns, we can use .iloc
to select the row and pass a list of integer indices corresponding to the columns we want to update.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# update the values in the second row, columns A and C
df.iloc[1, [0, 2]] = [10, 30]
print(df)
This will output:
A B C
0 1 4 7
1 10 5 30
2 3 6 9
Updating values based on a condition
Sometimes we need to update values in a DataFrame based on a condition. For example, we may want to update all the values in a column that are greater than a certain threshold. We can use boolean indexing to select the rows that meet the condition, and then update the values in the desired column.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# update all the values in column B that are greater than 4
df.loc[df['B'] > 4, 'B'] = 0
print(df)
This will output:
A B
0 1 4
1 2 0
2 3 0
Conclusion
In this article, we have explored different ways to update values in a specific row in a Python Pandas DataFrame. We have seen how to update a single value, multiple values, and values based on a condition. By mastering these techniques, you will be able to manipulate and analyze data with more precision and efficiency.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.