How to Update a Pandas DataFrame Row with New Values
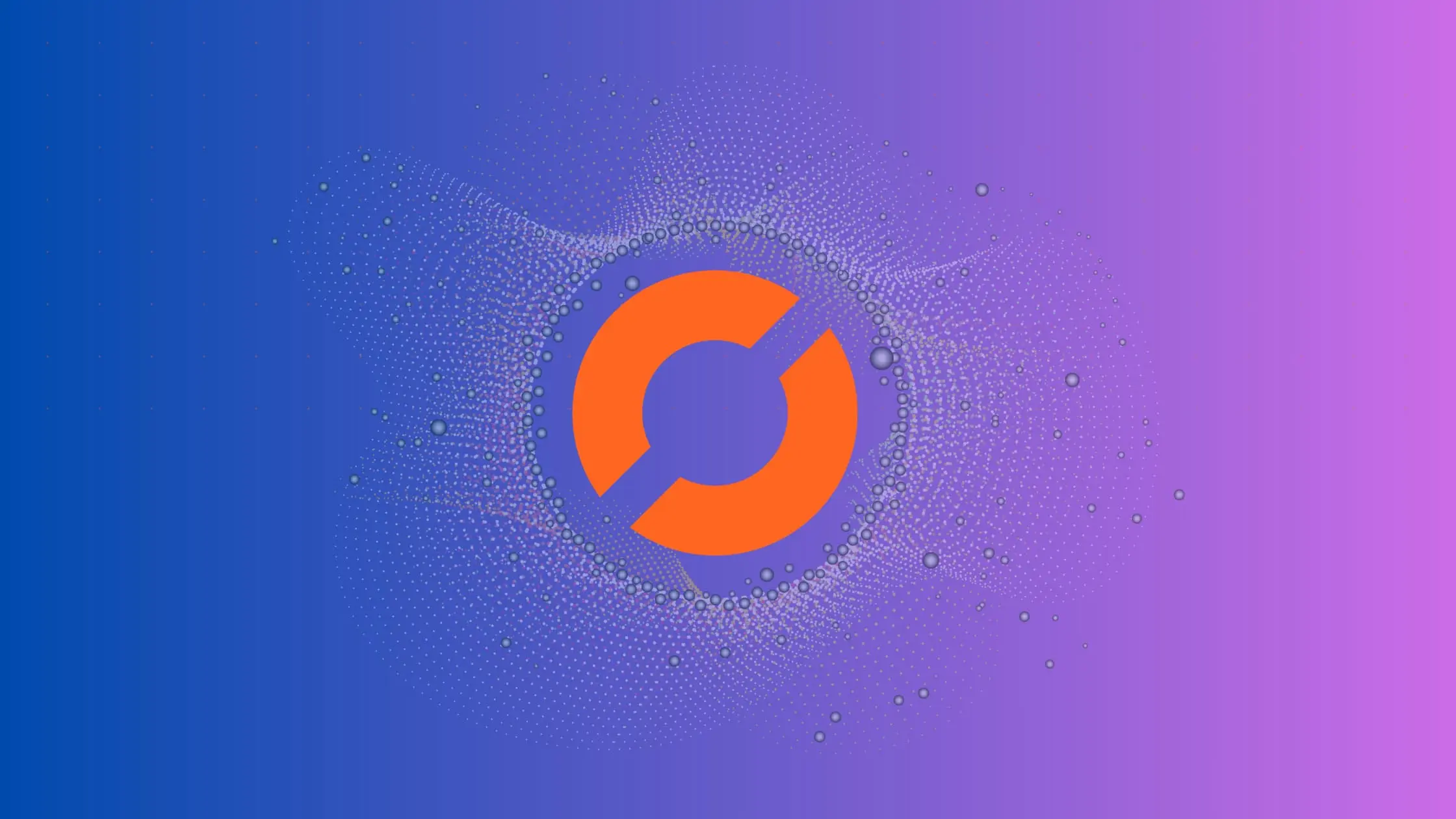
Table of Contents
Introduction to Pandas DataFrame
Before we dive into the process of updating a Pandas DataFrame row, let’s first understand what a Pandas DataFrame is and how it works.
A Pandas DataFrame is a two-dimensional size-mutable, tabular data structure with rows and columns, similar to a spreadsheet or SQL table. It is built on top of the NumPy library and provides powerful data manipulation capabilities, including slicing, indexing, filtering, merging, and grouping.
Updating a Pandas DataFrame Row
There are several ways to update a row in a Pandas DataFrame. Here are some common methods:
Using .loc[] or .iloc[]
To update a Pandas DataFrame row with new values, we first need to locate the row we want to update. This can be done using the loc
or iloc
methods, depending on whether we want to locate the row by label or integer index.
Once we have located the row, we can update the values of the row using the assignment operator =
. We simply need to assign the new values to the row using the column names as keys.
Here’s an example. Let’s say we have a Pandas DataFrame with three columns: Name
, Age
, and Gender
. We want to update the row with Name
= “John” and set the Age
to 35 and Gender
to “Male”. We can do this as follows:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({
'Name': ['John', 'Mary', 'Peter'],
'Age': [30, 25, 35],
'Gender': ['Male', 'Female', 'Male']
})
# locate the row to update
row_index = df.loc[df['Name'] == 'John'].index[0]
# update the row with new values
df.loc[row_index, 'Age'] = 35
df.loc[row_index, 'Gender'] = 'Male'
# print the updated dataframe
print(df)
Output:
Name Age Gender
0 John 35 Male
1 Mary 25 Female
2 Peter 35 Male
In this example, we first create a sample Pandas DataFrame with three columns: Name
, Age
, and Gender
. We then locate the row with Name
= “John” using the loc
method and assign its index to the row_index
variable.
Next, we update the values of the row using the assignment operator =
. We assign the new value 35 to the Age
column and the new value “Male” to the Gender
column using the column names as keys.
Finally, we print the updated dataframe, which now has the row with Name
= “John” updated with the new values.
Using .at[] with Individual Values
The .at[] accessor allows you to access and modify a single value at a time.
df.at[0, 'Age'] = '35'
This will change the Age
value at index 1
to 35
Using Boolean Indexing
Identify the row you want to update using boolean indexing.
df.loc[df['Name'] == 'John', 'Age'] = 35
This updates the Age
value for the row where Name is ‘John’
Common Errors
Indexing errors: Ensure your index selection method (loc, iloc, boolean) targets the correct row. Miscounting indices or using incorrect labels can lead to updating the wrong row.
Missing key errors: When using assignment with column names, ensure the columns you’re referencing actually exist in the DataFrame. Trying to update non-existent columns will raise errors.
Type mismatch: Make sure the new values you’re assigning are compatible with the data type of the column you’re updating. Trying to assign strings to numerical columns or vice versa might raise errors.
Circular dependencies: When using .loc or .iloc with assignment, avoid relying on the updated values within the same operation. This can lead to unexpected results or infinite loops.
Pros and Cons
.loc[] and .iloc[]:
- Pros: Precise control over row selection by label or index, easy to understand and implement.
- Cons: Can be verbose for updating multiple columns, requires additional steps for locating rows if not already known.
.at[]:
- Pros: Efficient for modifying single values, concise syntax.
- Cons: Less intuitive for updating multiple values or entire rows, limited functionality compared to .loc and .iloc.
Boolean Indexing:
- Pros: Flexible for updating subsets of rows based on conditions, efficient for large DataFrames.
- Cons: More complex syntax than direct indexing, might be less clear for beginners.
Additional Tips:
- When unsure about the index, use df.index to list all available indices.
- Use .copy() to create a copy of the DataFrame before updating, allowing for safe experimentation and avoiding accidental modifications to the original data.
Conclusion
Updating a Pandas DataFrame row with new values is a simple and straightforward process that can be done using various methods in the Pandas library in Python. Choose the method that best fits your specific use case and preferences. Note that some methods may be more efficient than others depending on the size of your DataFrame and the nature of your updates.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.