How to Subset a Pandas DataFrame with a Value in a List Using Python
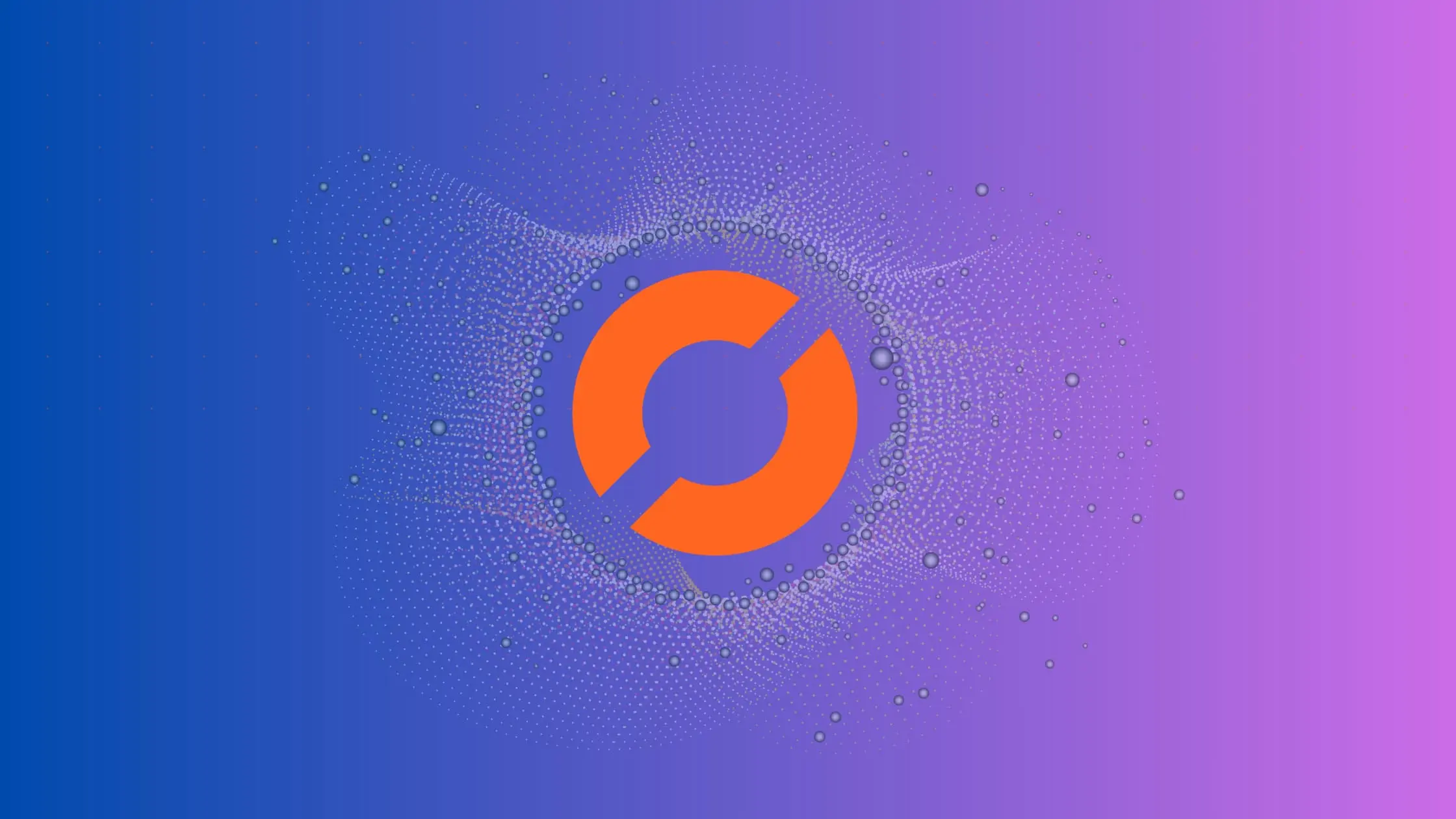
As a data scientist or software engineer, working with large datasets is a common task. Often, we need to filter or subset the data to work on a specific subset of interest. In this blog post, we will explore how to subset a pandas DataFrame with a value in a list using Python.
Table of Contents
- What is Pandas?
- How to Subset a Pandas DataFrame with a Value in a List Using Python
- Using the
query()
Method - Error Handling
- Conclusion
What is Pandas?
Pandas is a popular open-source data manipulation library for Python. It provides data structures and functions to manipulate and analyze the data in tabular form. Pandas is built on top of NumPy and offers fast and efficient data processing capabilities.
How to Subset a Pandas DataFrame with a Value in a List Using Python
Suppose we have a dataset with multiple columns and we want to extract rows with a specific value in a particular column. We can achieve this by using the pandas isin()
method.
The isin()
method is used to filter data based on a list of values. It returns a boolean series that can be used to filter the DataFrame. To extract the subset of data with the desired value, we can pass the boolean series as an indexing argument to the DataFrame.
Here is an example of how to extract data from a DataFrame using the isin()
method:
import pandas as pd
# create a sample DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Age': [25, 30, 35, 40, 45],
'Gender': ['F', 'M', 'M', 'M', 'F']}
df = pd.DataFrame(data)
# subset the DataFrame with a specific value in a column
subset = df[df['Name'].isin(['Bob', 'David'])]
print(subset)
The output of the above code will be:
Name Age Gender
1 Bob 30 M
3 David 40 M
In the above example, we create a sample DataFrame with three columns: Name
, Age
, and Gender
. We then use the isin()
method to extract the rows where the Name
column contains either 'Bob'
or 'David'
. The resulting subset DataFrame contains only the rows where the condition is true.
Pros
- Readability and Simplicity: The
isin()
method provides a clear and straightforward syntax, making it easy to read and understand. - Versatility: It is versatile and can be applied to filter based on multiple values in a single column.
- Widely Supported:
isin()
is a commonly used and well-documented method in Pandas, making it easy for others to understand and maintain your code.
Cons
- Limited to Equality Checks: The
isin()
method is primarily designed for equality checks, and it might not be as suitable for more complex conditions. - Requires Creating a List: It requires creating a list of values, which could be cumbersome if dealing with a large number of values.
Using the query() Method
Another effective method for subsetting a Pandas DataFrame with a value in a list is by utilizing the query()
method. The query()
method allows you to filter the DataFrame based on a specified condition.
Here’s an example of how to use the query()
method for subsetting:
import pandas as pd
# create a sample DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Age': [25, 30, 35, 40, 45],
'Gender': ['F', 'M', 'M', 'M', 'F']}
df = pd.DataFrame(data)
# subset the DataFrame with a specific value in a column using query()
subset = df.query('Name in ["Bob", "David"]')
print(subset)
The output of the above code will be the same as the previous example:
Name Age Gender
1 Bob 30 M
3 David 40 M
In this example, the query()
method is employed to filter the DataFrame based on the condition that the ‘Name’ column should be in the list [‘Bob’, ‘David’]. The resulting subset DataFrame contains only the rows where this condition is met.
Using the query()
method provides an alternative and concise way to perform DataFrame subsetting, particularly when dealing with complex conditions or expressions. It adds flexibility to your data manipulation toolkit in addition to the isin()
method.
Pros
- Conciseness: The
query()
method allows for concise and expressive filtering conditions, especially for more complex queries. - Dynamic Expression: It allows the use of dynamic expressions, making it suitable for scenarios where the filtering conditions are not known in advance.
- Readability for Complex Conditions: It can be more readable when dealing with complex conditions involving multiple columns and logical operators.
Cons:
- Learning Curve: Some users may find the syntax and usage of the
query()
method less intuitive, leading to a steeper learning curve. - Limited to String Expressions: The filtering condition needs to be specified as a string expression, which may be less familiar for users accustomed to using Python expressions directly.
Error Handling
- Column Existence: If the specified column for filtering does not exist in the DataFrame, a
ValueError
may occur. Use a try and except block to catch aValueError
and handle it appropriately. - Values Not Found: If any of the values in the list are not present in the specified column, the
isin()
method will still work, but it may not yield the expected results. - Query String Syntax: If there are issues with the query string, such as incorrect syntax, a
pd.errors.QuerySyntaxError
may occur. Specifically handlepd.errors.QuerySyntaxError
to address potential syntax errors in the query string.
Conclusion
In conclusion, subsetting a pandas DataFrame with a value in a list is a fundamental task in data analysis, crucial for isolating specific data points of interest. The isin()
method, demonstrated earlier, provides a straightforward and efficient means of achieving this, allowing for the extraction of relevant information based on predefined conditions.
Additionally, an alternative method, the query()
method, was introduced. This method offers a concise and expressive way to filter a DataFrame based on more complex conditions. By embracing the flexibility provided by both isin()
and query()
, data scientists and software engineers can tailor their approaches to the intricacies of the data they are working with.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.