How to Split a Date Column into Separate Day Month Year Columns in Pandas
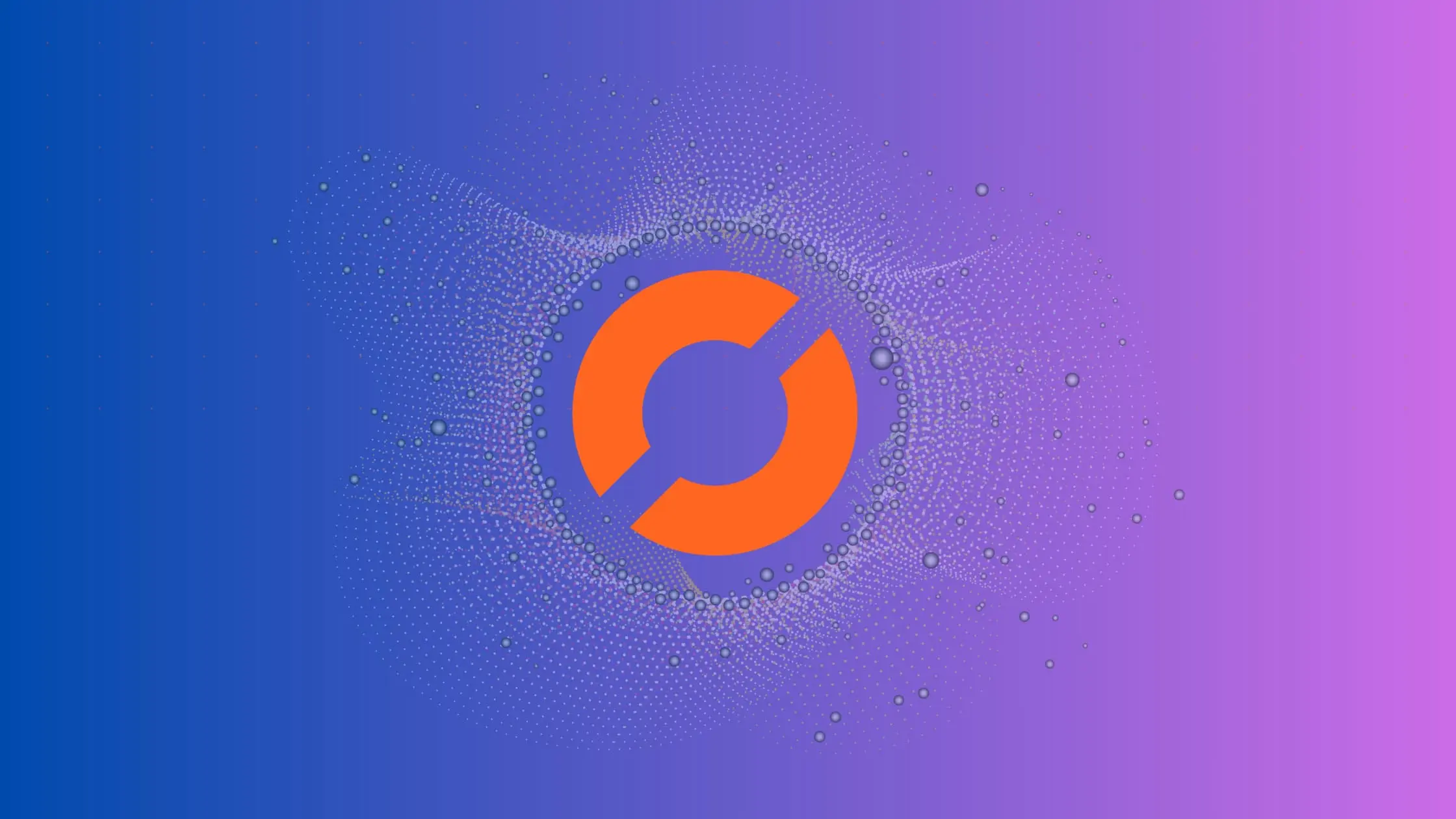
As a data scientist or software engineer, you may come across a scenario where you need to split a date column into separate day, month, and year columns. This can be a common requirement in data analysis and machine learning projects, especially when dealing with time series data.
In this article, we will explore how to split a date column into separate day, month, and year columns in Pandas, a popular data manipulation library in Python. We will provide step-by-step instructions and code examples to demonstrate how to achieve this task.
What is Pandas?
Pandas is an open-source data manipulation library in Python that provides high-performance, easy-to-use data structures and data analysis tools. It is built on top of NumPy, another popular library for scientific computing in Python.
Pandas provides a wide range of data manipulation functions, including filtering, grouping, pivoting, merging, and reshaping data. It also supports handling missing data, time series data, and data with different types and formats.
How to Split a Date Column into Separate Day, Month, Year Columns in Pandas
Let’s assume that we have a data frame with a date column in the format "YYYY-MM-DD"
, and we want to split this column into separate day, month, and year columns.
import pandas as pd
# create a sample data frame
data = {'date': ['2022-01-01', '2022-02-01', '2022-03-01']}
df = pd.DataFrame(data)
# show the original data frame
print(df)
date
0 2022-01-01
1 2022-02-01
2 2022-03-01
To split the date column into separate day, month, and year columns, we can use the pd.to_datetime()
function to convert the date column into a Pandas datetime object. Then, we can use the dt
accessor to extract the day, month, and year components from the datetime object.
# convert the date column into a datetime object
df['date'] = pd.to_datetime(df['date'])
# extract the day, month, and year components
df['day'] = df['date'].dt.day
df['month'] = df['date'].dt.month
df['year'] = df['date'].dt.year
# show the modified data frame
print(df)
date day month year
0 2022-01-01 1 1 2022
1 2022-02-01 1 2 2022
2 2022-03-01 1 3 2022
As you can see, the date column has been successfully split into separate day, month, and year columns.
Conclusion
In this article, we have demonstrated how to split a date column into separate day, month, and year columns in Pandas. We used the pd.to_datetime()
function to convert the date column into a Pandas datetime object, and then used the dt
accessor to extract the day, month, and year components from the datetime object.
This technique can be useful in data analysis and machine learning projects, especially when dealing with time series data. We hope that this article has been helpful in demonstrating how to achieve this task in Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.