How to Specify Column Names while Reading an Excel File using Pandas
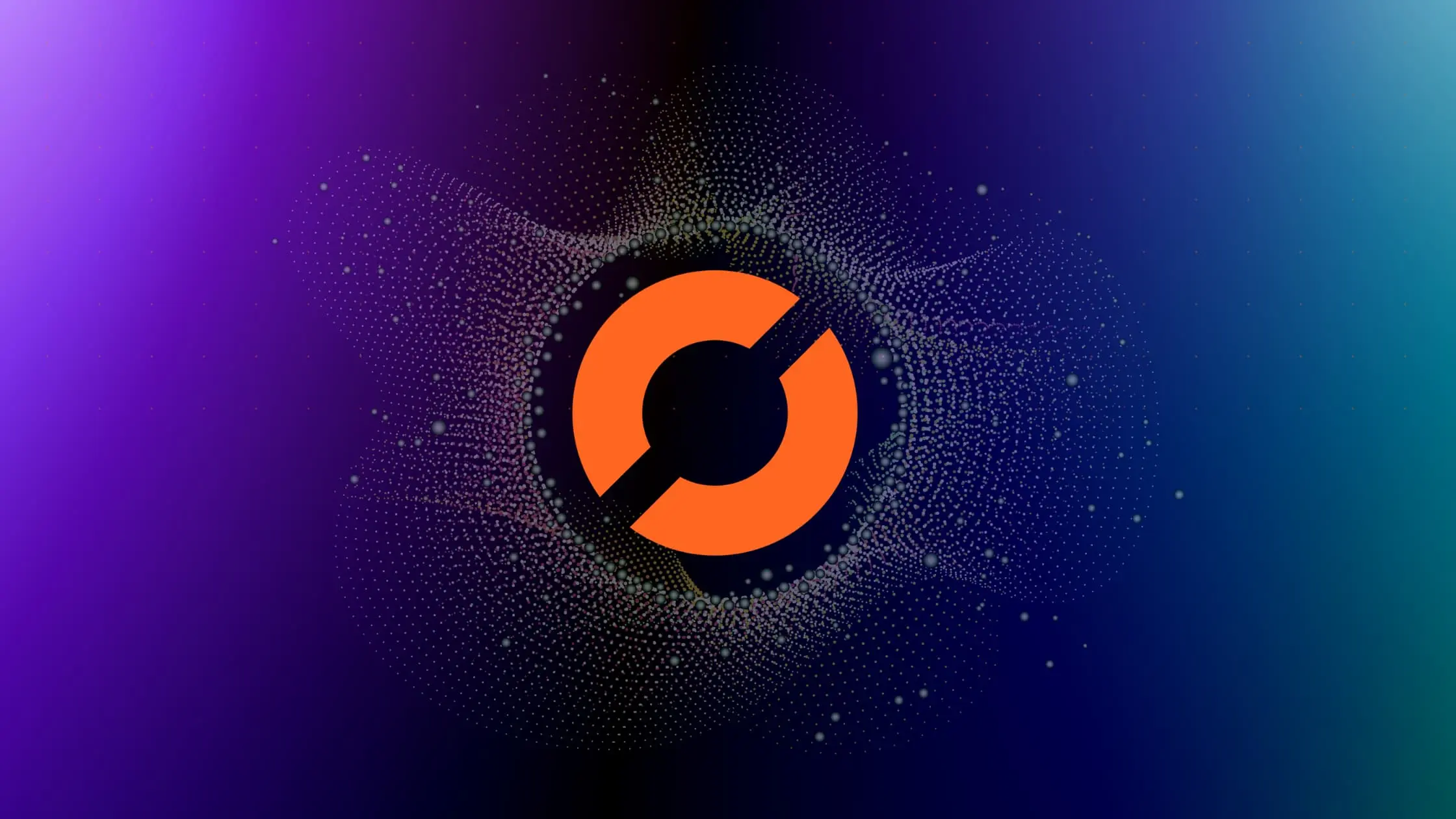
As a data scientist or software engineer, you may often need to work with Excel files that contain large amounts of data. While these files are easy to use, they can be a bit tricky when it comes to reading them into Pandas to perform data analysis. One of the common issues that you may encounter while reading Excel files is that the column names may not be located in the first row, which can cause problems when you try to analyze the data.
In this blog post, we will explore how to specify column names while reading an Excel file using Pandas. We will begin by discussing the importance of column names and how they can impact your data analysis. After that, we will dive into the specifics of reading Excel files into Pandas and how to handle the issue of non-standard column names.
Table of Contents
The Importance of Column Names
Column names are an essential part of any data analysis process. They provide context to the data and help you understand what each column represents. Without proper column names, it can be challenging to interpret the data and draw meaningful insights from it.
Moreover, incorrect or inconsistent column names can cause errors in your analysis. For instance, if you have a column named “Age” in one file and “age” in another, Pandas would treat them as two separate columns, which can lead to incorrect results.
Reading Excel Files into Pandas
Pandas provides a couple of methods to read Excel files, including read_excel()
and ExcelFile()
. The read_excel()
function is a convenient way to load Excel files into a Pandas DataFrame. You can use this function to read an entire sheet or a specific range of cells from the sheet.
Here is an example of how to use read_excel()
to load an Excel file into a Pandas DataFrame:
Excel example:
import pandas as pd
df = pd.read_excel('my_file.xlsx')
Output:
First Name Last Name Phone Number Email Address
0 John Doe 123-456-7890 john@example.com
1 Jane Smith 987-654-3210 jane@example.com
2 Sam Johnson 555-123-4567 sam@example.com
3 Emily Williams 777-888-9999 emily@example.com
By default, read_excel()
uses the first row of the Excel file as the column names. However, if your Excel file has a header row that is not located in the first row, you can specify the header row index using the header
parameter.
For instance, if your header row is located in the second row of your Excel file, you can use the following code to read the file into a Pandas DataFrame:
import pandas as pd
df = pd.read_excel('my_file.xlsx', header=1)
Output:
John Doe 123-456-7890 john@example.com
0 Jane Smith 987-654-3210 jane@example.com
1 Sam Johnson 555-123-4567 sam@example.com
2 Emily Williams 777-888-9999 emily@example.com
This will read the Excel file and use the second row as the column names.
Handling Non-Standard Column Names
If your Excel file has non-standard column names, such as spaces or special characters, Pandas may not be able to read them properly. In such cases, you can use the names
parameter to specify the column names explicitly.
Here is an example of how to use the names
parameter to specify column names while reading an Excel file:
import pandas as pd
cols = ['First Name', 'Last Name', 'Phone Number', 'Email Address']
df = pd.read_excel('my_file.xlsx', header=1, names=cols)
Output:
First Name Last Name Phone Number Email Address
0 Jane Smith 987-654-3210 jane@example.com
1 Sam Johnson 555-123-4567 sam@example.com
2 Emily Williams 777-888-9999 emily@example.com
This will read the Excel file and use the cols
list as the column names.
Another issue that you may encounter while reading Excel files is that they may contain multiple header rows. In such cases, you can use the skiprows
parameter to skip the header rows and read the data starting from a specific row.
Here is an example of how to use the skiprows
parameter to skip header rows:
import pandas as pd
df = pd.read_excel('my_file.xlsx', skiprows=[0, 1, 2], header=None)
Output:
0 1 2 3
0 Sam Johnson 555-123-4567 sam@example.com
1 Emily Williams 777-888-9999 emily@example.com
This will skip the first three rows of the Excel file and read the data starting from the fourth row. The header=None
parameter tells Pandas that the file does not contain a header row, and it should create default column names.
Conclusion
In this blog post, we have discussed how to specify column names while reading an Excel file using Pandas. We started by highlighting the importance of column names in data analysis and how they can impact your results. Then, we explored how to read Excel files into Pandas and how to handle the issue of non-standard column names.
By following the tips and tricks outlined in this post, you can ensure that your data analysis process is error-free and produces accurate results. So the next time you need to read an Excel file into Pandas, you know exactly what to do!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.