How to Solve 'CUDA out of memory' in PyTorch
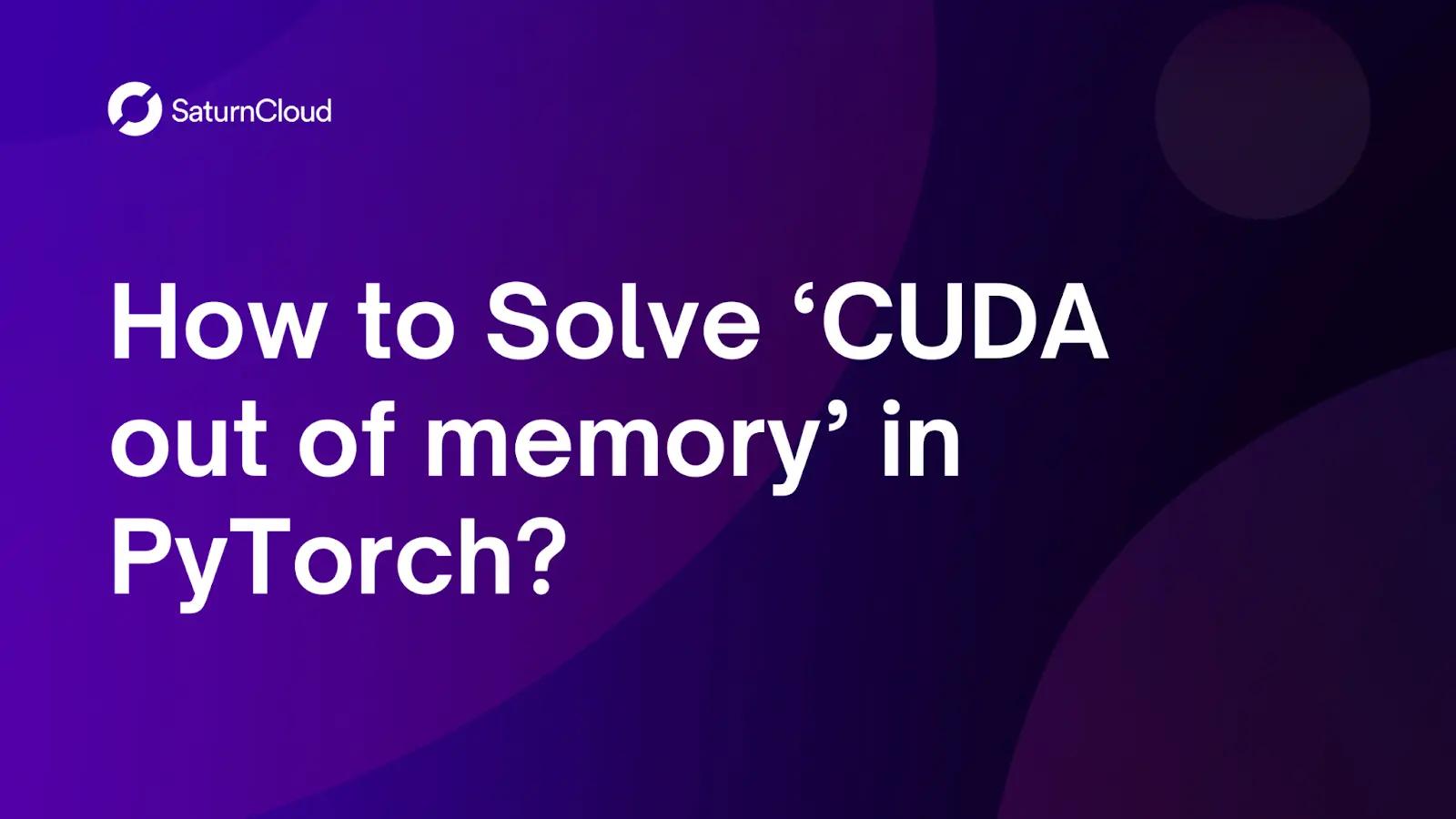
If you’ve ever worked with large datasets in PyTorch, chances are you’ve encountered the dreaded ‘CUDA out of memory’ error. This error message occurs when your GPU runs out of memory while trying to allocate space for tensors in your PyTorch model. Out-of-memory errors can be frustrating, especially when you’ve spent much time fine-tuning your model and optimizing your code.
🚀 Streamline your model training with Saturn Cloud from monitoring memory to leveraging multiple GPUs. Scale efficiently and dive into large-scale model training without the frustration. Start for free here.
In this blog post, we’ll explore some common causes of this error and provide solutions to help you solve it.
What Causes ‘CUDA out of memory’ in PyTorch?
You might encounter the ‘CUDA out of memory’ error in PyTorch for several reasons. Some of the most common causes include:
Large batch sizes: One of the most common causes of this error is trying to train your model with a batch size that’s too large. When you increase the batch size, you ask your GPU to process more data simultaneously, requiring more memory. If your GPU doesn’t have enough memory to store the entire batch, you’ll see the ‘CUDA out of memory’ error.
Large model architecture: Another reason why you might see this error is if you’re using a large model architecture. Larger models require more memory to store their parameters and process data, which can quickly consume your GPU’s memory.
Not freeing up memory: If you’re not adequately freeing up memory after each iteration of your model, you can quickly run out of memory. To keep system memory management under control, use PyTorch’s built-in memory management functions and proactively release variables that are no longer needed.
Accumulating intermediate gradients: By default, PyTorch will keep track of all variables requiring gradients. This logging can quickly consume all the available GPU memory, especially if you are training a large model with a large batch size.
GPU memory leaks: In some cases, PyTorch programs can leak GPU memory, meaning the program allocates GPU memory but does not release it when it is no longer needed. Eventually, your GPU will run out of memory, resulting in the ‘CUDA out of memory’ error.
Now that we know what causes the ‘CUDA out of memory’ error, let’s explore some solutions to help you solve it.
How to Solve ‘CUDA out of memory’ in PyTorch
Solution #1: Reduce Batch Size or Use Gradient Accumulation
As we mentioned earlier, one of the most common causes of the ‘CUDA out of memory’ error is using a batch size that’s too large. If you’re encountering this error, try reducing your batch size and see if that helps. You can also try using gradient accumulation, which allows you to effectively use a larger batch size without running out of memory.
Gradient accumulation is a powerful technique that allows training of large batch sizes, even when the available GPU memory cannot process them all simultaneously. Instead of updating model parameters after each mini-batch, the gradients across successive mini-batches are aggregated over a specified number of iterations before executing a single weight update.
To implement gradient accumulation in PyTorch, modify your training loop to not call optimizer.step()
and optimizer.zero_grad()
after every backward pass. Instead, these functions are called once every ‘n’ backward passes (where ‘n’ is the number of steps you want to accumulate gradients over):
optimizer.zero_grad() # Explicitly zero the gradient buffers
for i in range(num_mini_batches):
inputs, labels = next(training_data)
inputs, labels = inputs.to(device), labels.to(device)
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward() # Backward pass to calculate the gradient
if (i+1) % accumulation_steps == 0: # Wait for several backward passes
optimizer.step() # Now we can do an optimizer step
optimizer.zero_grad() # Reset gradients to zero
Solution #2: Use a Smaller Model Architecture
The choice of model architecture has a significant impact on your memory footprint. It’s common for newer or deeper models with many layers or complex structures to consume more memory to store model parameters during the forward/backward passes. If you find yourself frequently running into ‘CUDA out of memory’ errors, one option is opting for a smaller model architecture.
Going for a smaller or simpler model doesn’t necessarily mean a degraded performance. Many models are available online that are memory-efficient while maintaining competitive performance. For instance, MobileNet and EfficientNet provide a good trade-off between computational resources and model accuracy. Such architectures utilize depthwise separable convolutions to reduce the number of trainable parameters without sacrificing too much accuracy.
Another approach is to apply model pruning techniques. Pruning is a process where a proportion of a network’s nodes are removed, leaving a smaller, leaner network that uses less memory. Methods include unstructured pruning, where individual neuron connections are pruned based on specific criteria, and structured pruning, which removes entire channels or nodes from the network, perhaps based on their relevance scores. You can view examples of utilizing PyTorch to prune from their documentation here.
Lastly, consider leveraging knowledge distillation techniques where the acquired knowledge from a larger pre-trained model (teacher model) is transferred to a smaller model (student model). While implementing this might require additional steps, these distilled models can often reach a performance very close to the original larger models but with significantly fewer parameters.
Solution #3: Use Mixed Precision Training
PyTorch supports mixed precision training, which can help reduce memory usage by using lower-precision data types for certain parts of your model. Mixed precision training is a method that capitalizes on the performance capability of modern GPUs without significantly affecting model accuracy. It combines the use of 32-bit and 16-bit floating point types to maximize speed and efficiency, while reducing memory usage and maintaining the neural network performance.
Many parts of deep learning models, like activation functions, are less sensitive to precision. Therefore, carrying out these computations in half-precision (float16) can lead to a reduced memory footprint and faster execution time without compromising model quality.
Implementing mixed precision training in PyTorch is relatively straightforward, thanks to the torch.cuda.amp
package. This package provides a Python context manager, amp.autocast
, to perform operations with a chosen precision.
model = ...
optimizer = ...
scaler = torch.cuda.amp.GradScaler()
for inputs, labels in data:
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
# Runs the forward pass with autocasting
with torch.cuda.amp.autocast():
outputs = model(inputs)
loss = loss_fn(outputs, labels)
# Scales loss and performs backward pass using automatic mixed precision
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
You can also use the cuDNN backend for 16-bit training and inference for CUDA-enabled GPUs.
torch.backends.cudnn.benchmark = True
torch.backends.cudnn.enabled = True
By using half-precision (FP16) instead of single-precision (FP32), you can reduce your model’s memory usage by up to 50%. However, PyTorch batch-norm layers might have convergence issues. To remediate this, simply ensure your batch norm layers are float32 and remember to cast between float32 and float16 when necessary between the inputs and outputs of each layer.
To learn more about integrating mixed precision training, refer to PyTorch’s Automatic Mixed Precision (AMP) documentation here. Nvidia has also released tools to streamline mixed precision and distributed training in Pytorch called Apex.
Solution #4: Use PyTorch’s Memory Management Functions
PyTorch provides several built-in memory management functions to help you manage your GPU’s memory more efficiently. Some of these functions include:
torch.cuda.empty_cache()
- Releases all the unused cached memory currently held by the CUDA driver, which other processes can reuse.
torch.cuda.memory_allocated()
- Returns the current GPU memory managed by the PyTorch allocator in bytes
In-Place Operations: PyTorch allows for in-place operations that change the input tensors' values without creating a new tensor. These operations are followed by an underscore like
add_()
,relu_()
, and can save a lot of memory by cutting down on intermediate tensors. However, while this is memory-efficient, in-place operations might disrupt the computation graph and complicate gradient calculations, so they are generally not recommended. They’re most beneficial during model inference since backpropagation and associated gradients aren’t required. Another suitable situation is applying these operations on non-leaf variables or tensors disassociated from the graph where they don’t interfere with gradients. Despite these use cases, limit in-place operations to necessary instances for the sake of code readability and safety.Gradient Checkpointing: PyTorch provides gradient checkpointing, a technique that trades compute for memory. It allows you to run models that typically wouldn’t fit in memory at the cost of some speed since some stages are recomputed. That can be done using
torch.utils.checkpoint.checkpoint()
.
import torch
from torch.utils.checkpoint import checkpoint
# Assume `model` is an instance of a large model you want to checkpoint.
model = SomeLargeModel()
# Let's assume `input_data` is input to your model.
input_data = torch.randn(size=(1, 256, 256), requires_grad=True)
# Forward pass with checkpointing
output = checkpoint(model, input_data)
# Compute loss
loss = loss_fn(output, target)
# Backward pass
loss.backward()
- Parameter Swapping to/from CPU during Training: If some parameters are used infrequently, it might make sense to put them on CPU memory during training and move them to the GPU when needed.
Solution #5: Release Unused Variables
Managing variables properly is crucial in PyTorch to prevent memory issues. You can rapidly exhaust your memory if variables aren’t released when they’re no longer in use. To avert this, ensure you remove memory usage of tensors and other variables using the del command or set them to None
.
The memory management mechanism in Python slightly differs from other languages like C/C++, mainly due to its garbage collection system. In Python, a variable isn’t freed when it goes out of scope but rather when no more references to the variable exist. This characteristic could lead to unexpected memory retention, particularly when handling tensors in PyTorch.
Consider the following Python snippet:
i = None
for x in range(10):
if x % 2 == 0: # Only assign 'i' for even numbers
i = x
print(i) # 8 is printed
Even outside the loop’s scope, the output prints 8
, indicating that i
continues to exist, holding onto its memory allocation. This fact implicitly extends to PyTorch tensors, where memory occupied by tensors containing inputs and outputs may not be garbage collected when it’s no longer needed.
Hence, an excellent practice to adhere to is to delete tensors using del
, or set them to None
once they have served their purpose. Deleting or setting variables to None
ensures timely memory freeing as the garbage collector will then be able to deallocate these variables during its next iteration, preventing ‘CUDA out of memory’ errors.
Solution #6: Avoid accumulating intermediate gradients
During the training of neural networks, gradients play a crucial role. These gradients, which are intermediate derivatives computed during backpropagation, are essential for updating the model parameters. However, storing these intermediate gradients for every layer can consume substantial GPU memory. Releasing these gradients when they are no longer necessary is a way to curb this.
PyTorch provides a context manager function, torch.no_grad()
, which enables you to perform operations without tracking gradients or building the computational graph. This approach can significantly reduce memory usage.
with torch.no_grad():
prediction = model(input_data)
Note that torch.no_grad() should be used primarily in the context of model evaluation or inference — when the model is being used to make predictions on validation or test data, and the model’s parameters are not being updated. In this scenario, not computing gradients saves both memory and computation time.
Alternatively, the .detach()
method can be used on a tensor to remove it from the computation graph. The result is a tensor that doesn’t require gradients, eliminating the need for PyTorch to reserve memory for them.
output = model(input_data).detach()
However, it’s crucial to understand that while these techniques can help manage memory usage, they should be applied judiciously. Gradient computation is vital for updating your neural network model parameters during training. So, turning gradient computation off during training can hinder the model’s ability to learn effectively. Use these utilities wisely where gradients are not required to avoid compromising the model’s training cycle.
Adding More Memory with Another GPU
When you’re training larger models or dealing with extensive datasets, enlarging your computational resources becomes a necessity. Employing additional GPUs can be one way to address this. If your system or cluster houses more than one GPU, you can utilize their extra memory and processing power in the following ways:
Data Parallelism
If you want to increase your data bandwidth, using PyTorch’s nn.DataParallel
module can parallelize your model to run across several GPUs. The model gets replicated on each GPU, each working on a share of the input data. The outcomes from each are then compiled into a response. Not only does this method allow handling larger batch sizes, but it also accelerates computational speed.
model = Model()
if torch.cuda.device_count() > 1:
model = nn.DataParallel(model)
model = model.to(device)
However, this comes at the cost of higher communication overhead during gradient summing in the backward pass, and its efficiency drops as the number of GPUs increases. To learn more about data parallelism, click here.
Model Parallelism
When dealing with models too hefty to be accommodated in a single GPU’s memory, model parallelism comes into play. This method enables the distribution of network layers across different GPUs, allowing for the training of larger models. However, this might be slower due to inter-GPU communication bottlenecks.
model = Model()
model.layer1 = model.layer1.to('cuda:0')
model.layer2 = model.layer2.to('cuda:1')
While this method enables handling bigger models, inter-GPU communication may impact computational speed. To learn more about model parallelism, click here.
Distributed Training
Another effective way to increase your total resource pool is through distributed training. Distributed data-parallel (DDP) training proves helpful when dealing with numerous GPUs. PyTorch offers torch.nn.parallel.DistributedDataParallel
, a module that helps allocate input across specified devices by batch-dimension chunking. Herein, model parameters are replicated on each device, each of which manages a part of the input.
DDP is more efficient with a higher number of GPUs compared to data parallelism and requires less memory. Still, its setup can be more complex, especially in multi-node, multi-GPU scenarios. To learn more about DDP, click here.
Conclusion
Dealing with the ‘CUDA out of memory’ error in PyTorch is not uncommon when handling large datasets or complex models. While it can be a hindrance, understanding its core causes paves the way for efficient solutions. Adjusting the batch size, choosing smaller or more efficient model architectures, leveraging mixed precision training, effectively using PyTorch’s built-in memory management capabilities, and adequately releasing unused variables are critically important strategies to manage and optimize GPU memory usage.
Furthermore, memory requires proper monitoring and adjustment of code based on the consumption pattern, ensuring a smooth and efficient running of the machine learning model. Employing more GPUs can also come to the rescue to handle more substantial computational loads and data sizes. Techniques like data parallelism, model parallelism, and distributed data-parallel training can be efficiently utilized to scale up the model training across multiple GPUs.
Ultimately, resolving ‘CUDA out of memory’ errors might seem challenging, but with the proper techniques and appropriate practices, it is bound to get easier and make large-scale model training more efficient.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.