How to Skip Rows During CSV Import in Pandas
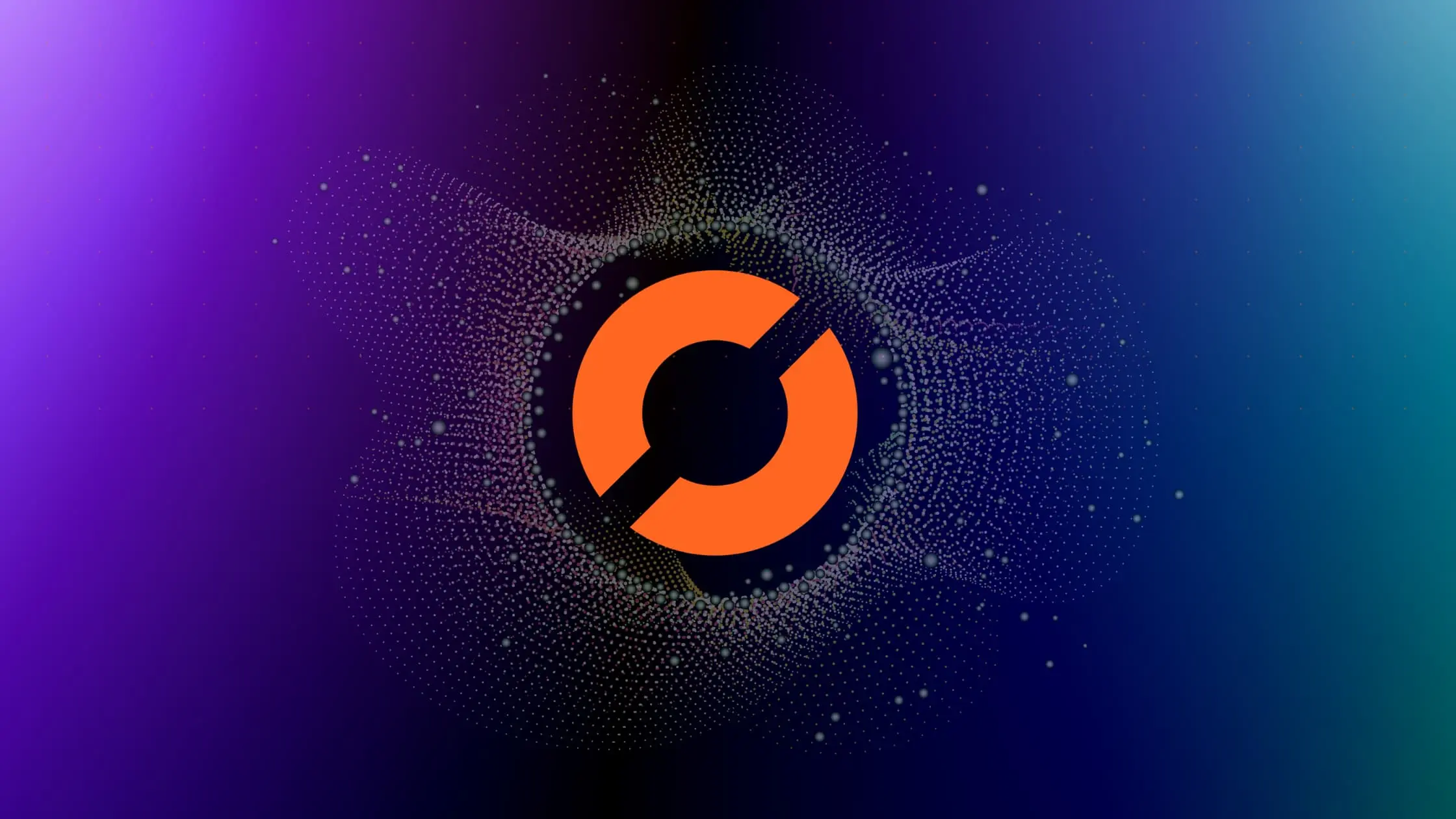
When working with large datasets in Python, importing data from CSV files is a common task. Pandas is a popular library for data manipulation and analysis that provides a simple and powerful way to read CSV files into a pandas DataFrame. However, sometimes you may need to skip rows during the CSV import process. In this article, we will show you how to do this using Pandas.
Table of Contents
- The Problem: Why Skip Rows During CSV Import?
- The Solution: How to Skip Rows During CSV Import
- Common Errors and How to Handle Them
- Conclusion
The Problem: Why Skip Rows During CSV Import?
Sometimes, CSV files may contain rows that are not relevant to the analysis or contain information that is not useful. These rows could be header rows, summary rows, or simply rows that are not structured the same way as the rest of the data.
Skipping these rows during CSV import can help improve the efficiency of your analysis by reducing the size of the DataFrame and eliminating irrelevant data. Additionally, it can prevent errors that may arise from trying to analyze data that is not structured correctly.
The Solution: How to Skip Rows During CSV Import
Pandas provides several parameters that allow you to skip rows during CSV import. These parameters are:
skiprows
: This parameter allows you to specify the number of rows to skip from the top of the CSV file.header
: This parameter allows you to specify the row number(s) to use as the column names. If you set this parameter to an integer, Pandas will skip all rows up to and including the specified row. If you set it to a list of integers, Pandas will use those rows as the column names and skip all other rows up to the row specified byskiprows
.skipfooter
: This parameter allows you to specify the number of rows to skip from the bottom of the CSV file. Let’s consider the following csv file
| Name | Age | Salary |
|----------|-----|--------|
| John | 25 | 50000 |
| Alice | 30 | 60000 |
| Bob | 28 | 55000 |
| Eva | 35 | 70000 |
| Charlie | 22 | 48000 |
| David | 32 | 62000 |
| Sophia | 29 | 58000 |
| Frank | 40 | 75000 |
| Grace | 26 | 52000 |
| Oliver | 33 | 64000 |
Example 1: Skip Rows at the Top of the CSV File
Suppose you have a CSV file that contains a header row and several summary rows at the top of the file that you want to skip during import. Here’s how you can do it using the skiprows
parameter:
import pandas as pd
# Import CSV file and skip the first 3 rows
df = pd.read_csv('data.csv', skiprows=3)
print(df)
In this example, we imported the CSV file named data.csv
and skipped the first 3 rows using the skiprows
parameter. Pandas will read the CSV file starting from the fourth row and create a DataFrame with the remaining rows.
Output:
Bob 28 55000
0 Eva 35 70000
1 Charlie 22 48000
2 David 32 62000
3 Sophia 29 58000
4 Frank 40 75000
5 Grace 26 52000
6 Oliver 33 64000
Example 2: Skip Rows at the Bottom of the CSV File
Suppose you have a CSV file that contains a summary row at the bottom of the file that you want to skip during import. Here’s how you can do it using the skipfooter
parameter:
import pandas as pd
# Import CSV file and skip the last row
df = pd.read_csv('data.csv', skipfooter=1)
In this example, we imported the CSV file named data.csv
and skipped the last row using the skipfooter
parameter. Pandas will read the CSV file up to the second-to-last row and create a DataFrame with the remaining rows.
Output:
Name Age Salary
0 John 25 50000
1 Alice 30 60000
2 Bob 28 55000
3 Eva 35 70000
4 Charlie 22 48000
5 David 32 62000
6 Sophia 29 58000
7 Frank 40 75000
8 Grace 26 52000
Example 3: header Parameter and Custom Skip
By adjusting the header parameter, you can skip rows dynamically. If your header is on, say, the third row, set header=2.
import pandas as pd
df = pd.read_csv('data.csv', header=2)
print(df)
Output:
Alice 30 60000
0 Bob 28 55000
1 Eva 35 70000
2 Charlie 22 48000
3 David 32 62000
4 Sophia 29 58000
5 Frank 40 75000
6 Grace 26 52000
7 Oliver 33 64000
Common Errors and How to Handle Them
Error 1: Inconsistent Data Types
When your CSV file contains columns with inconsistent data types, Pandas may raise a TypeError
.
Solution:
Specify the dtype
parameter to enforce data types during import.
Error 2: Blank or Missing Values
Blank or missing values can cause unexpected behavior during import, leading to ParserError
.
Solution:
Use the na_values
parameter to handle missing values.
Error 3: Incorrect Header Detection
If the header is not correctly detected, column alignment errors may occur.
Solution:
Manually specify the header using the header
parameter.
df = pd.read_csv('example.csv', dtype={'Age': int}, na_values=['N/A'], header=0)
print(df)
Conclusion
Skipping rows during CSV import in Pandas is a simple and powerful way to reduce the size of your DataFrame and eliminate irrelevant data. By using the skiprows
, header
, and skipfooter
parameters, you can skip rows at the top or bottom of the CSV file and set the column names for the DataFrame. By following the examples in this article, you should now be able to skip rows during CSV import in Pandas and improve the efficiency and accuracy of your data analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.