How to Select Rows from a DataFrame Based on List Values in a Column in Pandas
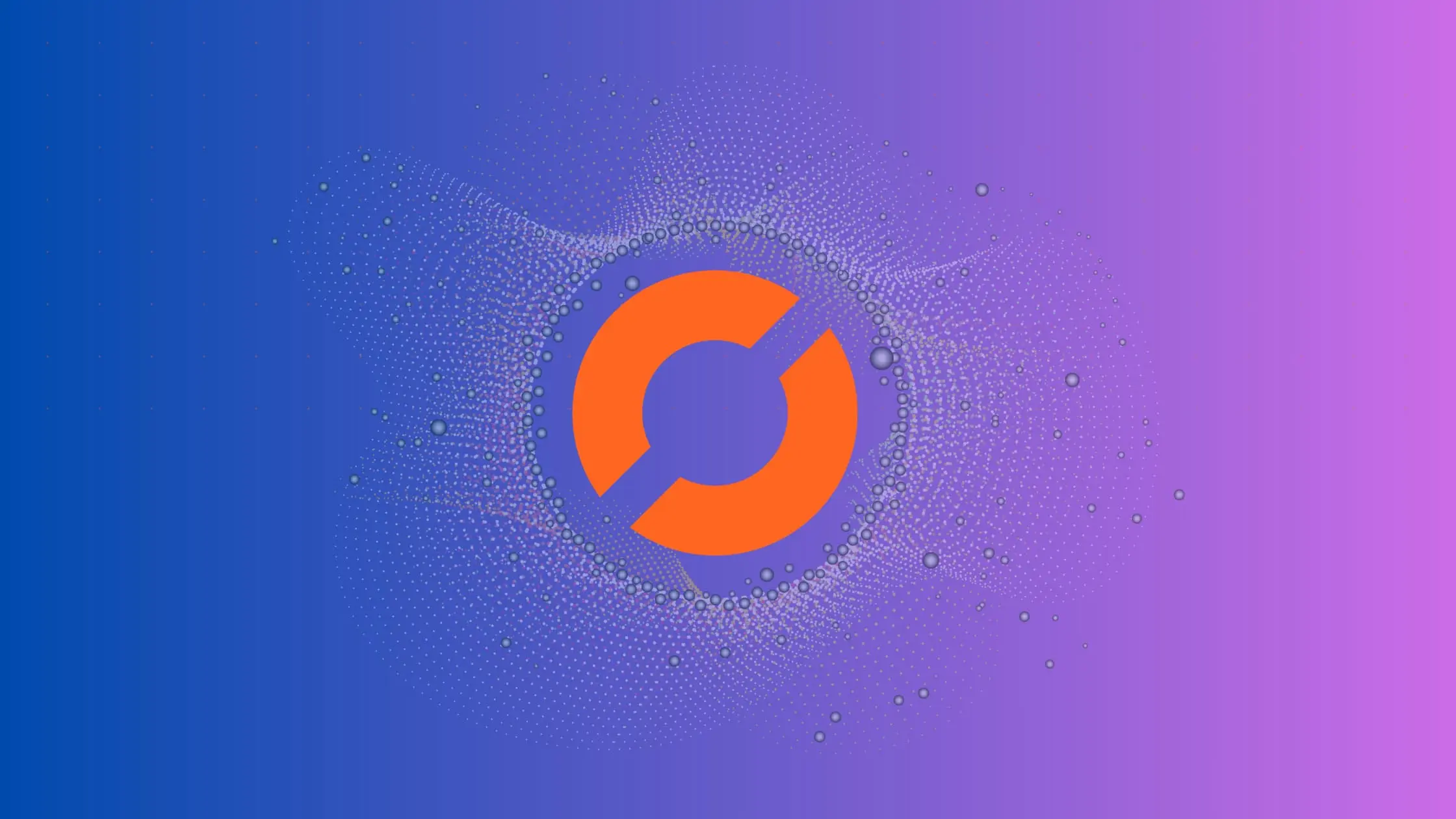
As a data scientist or software engineer, you may encounter tasks that involve filtering rows from a pandas DataFrame based on specific values in a column. One common scenario is when you have a list of values that you want to use as a filter criterion. In this article, we will discuss how to select rows from a DataFrame based on list values in a column using pandas.
Introduction to Pandas
Pandas is a popular open-source data manipulation library for Python. It provides a powerful data structure called a DataFrame, which is essentially a two-dimensional table with labeled rows and columns. The DataFrame is the primary data structure used in pandas for data analysis and manipulation.
The Problem
Suppose you have a DataFrame that contains customer data, including their names, email addresses, and subscription status. You want to select only the rows where the subscription status is “active” or “trial”, which are the values in a list called active_statuses
.
import pandas as pd
# create sample data
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Email': ['alice@example.com', 'bob@example.com', 'charlie@example.com', 'david@example.com', 'emily@example.com'],
'Subscription': ['trial', 'inactive', 'active', 'trial', 'inactive']}
df = pd.DataFrame(data)
# list of active subscription statuses
active_statuses = ['active', 'trial']
Selecting Rows Based on List Values
To select rows from a DataFrame based on a list of values in a column, we can use the isin()
method of pandas. This method returns a Boolean mask that indicates whether each element of a DataFrame column is contained in a list of values. We can then apply this mask to the DataFrame to select the desired rows.
# filter rows based on list values
mask = df['Subscription'].isin(active_statuses)
active_customers = df[mask]
print(active_customers)
The output of the above code will be:
Name Email Subscription
0 Alice alice@example.com trial
2 Charlie charlie@example.com active
3 David david@example.com trial
As you can see, the resulting DataFrame contains only the rows where the subscription status is active
or trial
.
Conclusion
In this article, we discussed how to select rows from a DataFrame based on list values in a column using pandas. We used the isin()
method to create a Boolean mask that indicates whether each element of a DataFrame column is contained in a list of values, and then applied this mask to the DataFrame to select the desired rows.
Pandas provides many other useful methods for data manipulation and analysis, making it a powerful tool for data scientists and software engineers. We hope that this article was helpful in solving your problem and that you learned something new about pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.