How to Select Row with Max Value in Column from Pandas groupby Groups
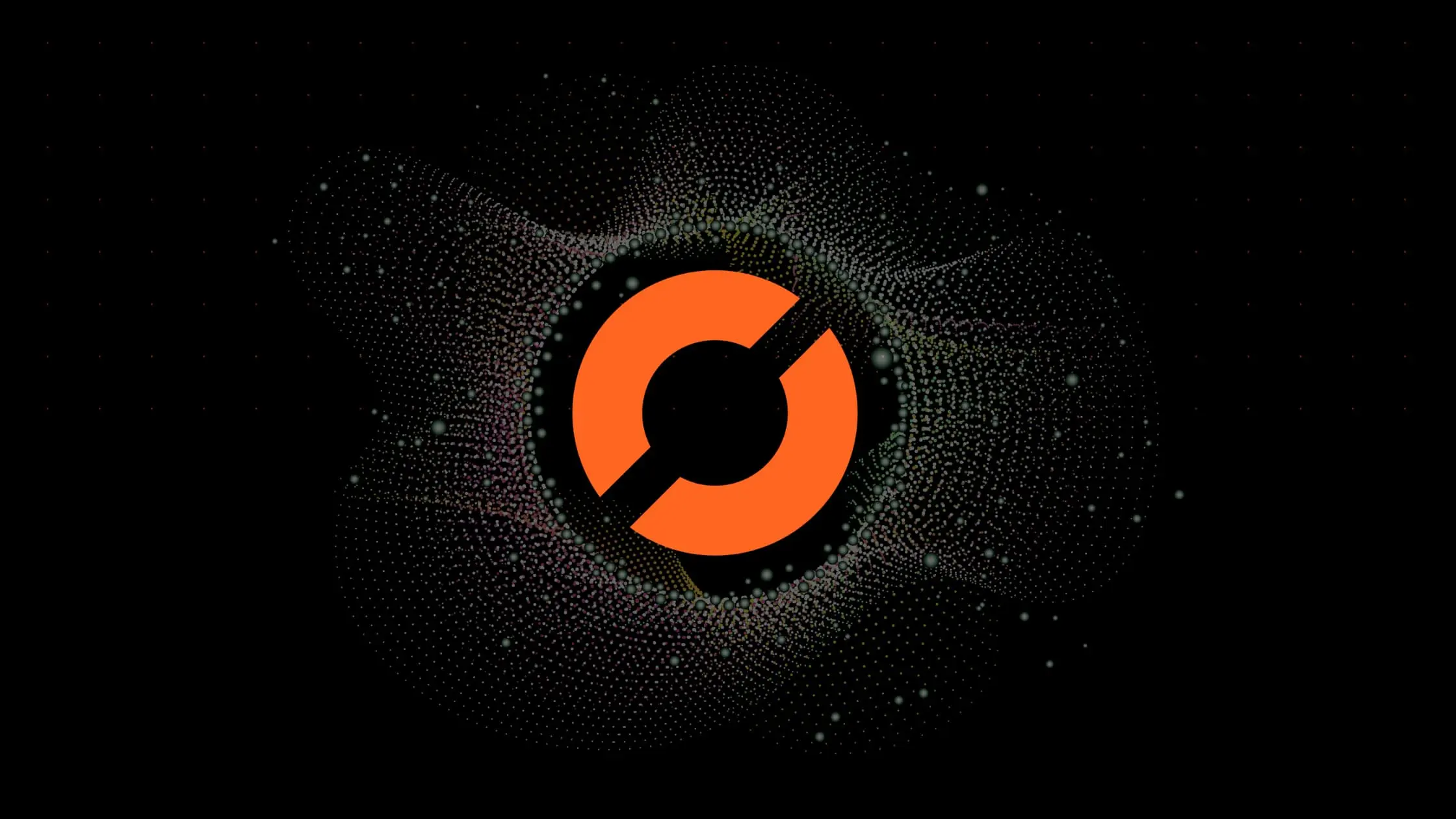
The Dataset
To demonstrate the various methods, let’s start by creating a sample dataset. We will create a Pandas DataFrame with three columns - ‘Name’, ‘Subject’, and ‘Score’. Each row represents a student’s score in a particular subject.
import pandas as pd
import numpy as np
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eva', 'Frank', 'Grace', 'Harry', 'Isabel', 'Jack'],
'Subject': ['Maths', 'Science', 'Maths', 'Science', 'Maths', 'Science', 'Maths', 'Science', 'Maths', 'Science'],
'Score': np.random.randint(60, 100, size=10)}
df = pd.DataFrame(data)
Our DataFrame looks like this:
Name Subject Score
0 Alice Maths 77
1 Bob Science 71
2 Charlie Maths 89
3 David Science 83
4 Eva Maths 89
5 Frank Science 94
6 Grace Maths 91
7 Harry Science 83
8 Isabel Maths 88
9 Jack Science 65
Using groupby() and idxmax()
The first method we will explore is using groupby() and idxmax(). groupby() groups the data by the ‘Subject’ column, and idxmax() returns the index of the row with the maximum value in the ‘Score’ column for each group.
idx = df.groupby('Subject')['Score'].idxmax()
max_scores = df.loc[idx]
Here, idx
is a Pandas Series that contains the row index of the maximum value for each group. We then use loc[] to select the rows with these indices to get the maximum scores for each group.
The resulting DataFrame looks like this:
Name Subject Score
6 Grace Maths 91
5 Frank Science 94
Using groupby() and apply()
Another method to find the row with the maximum value in a column from Pandas groupby() groups is using groupby() and apply(). We can define a function that returns the row with the maximum value in a column and apply it to each group.
def get_max_score(group):
return group.loc[group['Score'].idxmax()]
max_scores = df.groupby('Subject').apply(get_max_score)
Here, we define a function get_max_score()
that takes a group as input, finds the row with the maximum value in the ‘Score’ column using idxmax(), and returns the row. We then apply this function to each group using groupby() to get the maximum scores for each group.
The resulting DataFrame looks like this:
Name Subject Score
6 Grace Maths 91
5 Frank Science 94
Using groupby() and transform()
The final method we will explore is using groupby() and transform(). transform() applies a function to each group and returns a DataFrame with the same shape as the original DataFrame.
max_scores = df.loc[df.groupby('Subject')['Score'].transform(max) == df['Score']]
Here, we use groupby() to group the data by the ‘Subject’ column and then use transform() to find the maximum value of the ‘Score’ column for each group. We then compare this value with the ‘Score’ column of the original DataFrame to get the maximum scores for each group.
The resulting DataFrame looks like this:
Name Subject Score
6 Grace Maths 91
5 Frank Science 94
Conclusion
In this blog post, we have explored different methods to select the row with the maximum value in a column from Pandas groupby() groups. We have used groupby() and idxmax(), groupby() and apply(), and groupby() and transform() to achieve this task. Each of these methods has its advantages and disadvantages, and the choice of method depends on the specific use case.
Pandas is a powerful library that provides a wide range of functions to manipulate and analyze data. As a data scientist or software engineer, it is essential to have a good understanding of Pandas' capabilities and use them effectively to extract insights from data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.