How to Select Columns and Rows in Pandas Without Column or Row Names
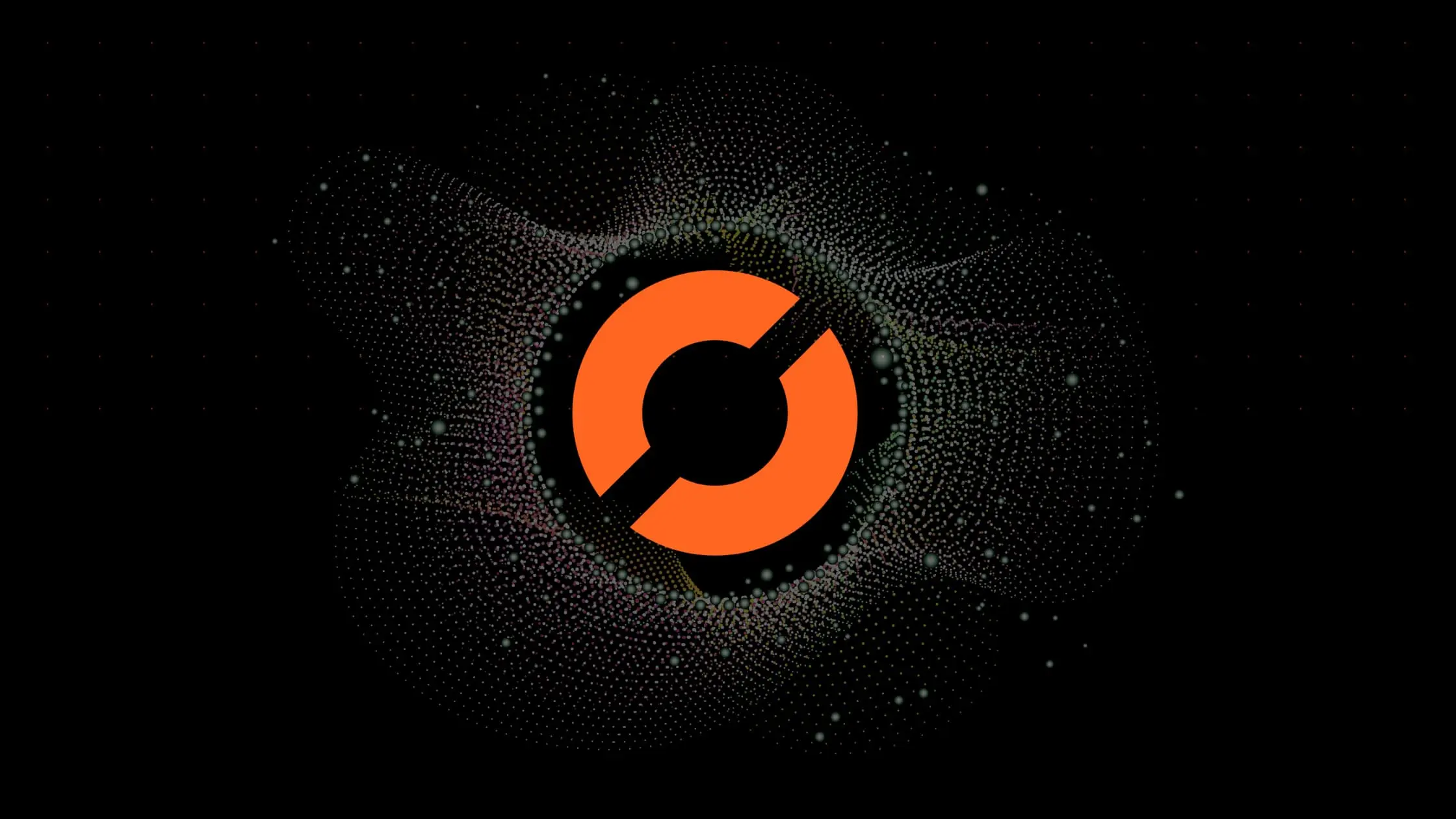
As a data scientist or software engineer, you are likely familiar with Pandas, the popular Python library for data manipulation and analysis. One of the most common tasks when working with Pandas is selecting specific columns and rows from a DataFrame. While this is straightforward when you know the names of the columns and rows, what if you don’t have access to this information? In this article, we’ll explore how to select columns and rows in Pandas without column or row names.
Table of Contents
- Introduction
- The Problem
- Selecting Columns
- Selecting Rows
- Pros and Cons of Positional Selection in Pandas
- Error Handling
- Conclusion
The Problem
Let’s set up a hypothetical scenario to explain the problem. Imagine you are working with a dataset where the column and row names are not available. All you have is the raw data in a Pandas DataFrame. You need to extract specific columns and rows based on their position in the DataFrame, but you don’t know their names.
Selecting Columns
To select columns without column names, you can use the iloc
method in Pandas. iloc
stands for “integer location” and allows you to select rows and columns by their position in the DataFrame, rather than by their labels.
To select a single column, you can pass the column’s index position to the iloc
method. For example, to select the first column in a DataFrame, you can use the following code:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# select the first column
first_column = df.iloc[:, 0]
Output:
0 1
1 2
2 3
Name: A, dtype: int64
In this example, df.iloc[:, 0]
selects all rows (:
) and the first column (0
) of the DataFrame. The resulting object is a Pandas Series containing only the values from the first column.
To select multiple columns, you can pass a list of column index positions to the iloc
method. For example, to select the first and third columns in a DataFrame, you can use the following code:
# select the first and third columns
first_and_third_columns = df.iloc[:, [0, 2]]
Output:
A C
0 1 7
1 2 8
2 3 9
In this example, df.iloc[:, [0, 2]]
selects all rows (:
) and the first and third columns ([0, 2]
) of the DataFrame. The resulting object is a Pandas DataFrame containing only the values from the first and third columns.
Selecting Rows
To select rows without row names, you can also use the iloc
method in Pandas. To select a single row, you can pass the row’s index position to the iloc
method. For example, to select the second row in a DataFrame, you can use the following code:
# select the second row
second_row = df.iloc[1, :]
Output:
A 2
B 5
C 8
Name: 1, dtype: int64
In this example, df.iloc[1, :]
selects the second row (1
) and all columns (:
) of the DataFrame. The resulting object is a Pandas Series containing only the values from the second row.
To select multiple rows, you can pass a slice of row index positions to the iloc
method. For example, to select the second and third rows in a DataFrame, you can use the following code:
# select the second and third rows
second_and_third_rows = df.iloc[1:3, :]
Output:
A B C
1 2 5 8
2 3 6 9
In this example, df.iloc[1:3, :]
selects the second and third rows (1:3
) and all columns (:
) of the DataFrame. The resulting object is a Pandas DataFrame containing only the values from the second and third rows.
Pros and Cons of Positional Selection in Pandas
Pros
Positional Flexibility: Using
iloc
allows for flexibility in selecting columns and rows based on their positions rather than relying on specific labels. This is beneficial when dealing with datasets where column and row names are unknown or not accessible.Ease of Use: The
iloc
method provides a concise and intuitive syntax for selecting columns and rows, making it easy for both beginners and experienced users to work with Pandas DataFrames.Efficiency: Selecting columns and rows by position using
iloc
can be more efficient than using label-based selection methods, especially when dealing with large datasets. It avoids the overhead associated with matching and searching for labels.Consistency: The use of
iloc
ensures a consistent approach to column and row selection across different scenarios, promoting code uniformity and ease of maintenance.
Cons
Index Dependency: The positional selection using
iloc
is index-dependent. If the DataFrame has a custom index, users need to be aware of the index positions, which might not align with the default integer index.Limited Intuitiveness: While
iloc
is powerful, it might be less intuitive for users who are accustomed to working with column and row names. The code might be less readable for someone unfamiliar with the specific positional indices.Potential for Ambiguity: In situations where the DataFrame structure is not well-documented, relying solely on positional indices might lead to ambiguity or errors if the structure changes.
Error Handling
Index Out of Range: When using
iloc
, it’s crucial to handle cases where the specified index positions are out of the valid range. This can be achieved by checking the length of the DataFrame and ensuring that the provided indices are within bounds.Non-Numeric Indices: If the DataFrame has non-numeric indices, users should handle potential errors resulting from trying to use integer-based indexing. It’s essential to validate the index types before applying
iloc
to avoid unexpected behavior.Empty DataFrame: Users should consider scenarios where the DataFrame might be empty or contain insufficient data. Proper checks should be implemented to handle such cases and prevent errors during column and row selection.
Documentation and Communication: In the absence of column and row names, thorough documentation becomes crucial. Provide clear instructions on the expected input format, index positions, and any potential pitfalls to help users understand and use the code correctly.
Conclusion
In this article, we have explored how to select columns and rows in Pandas without column or row names. To select columns, we used the iloc
method in Pandas and passed the index positions of the desired columns. To select rows, we also used the iloc
method and passed the index positions of the desired rows.
While working with data where column and row names are not available can be challenging, Pandas provides powerful tools for selecting specific columns and rows based on their position in the DataFrame. By using the iloc
method, you can extract the data you need and continue your analysis with confidence.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.