How to Search Pandas Data Frame by Index Value and Value in Any Column
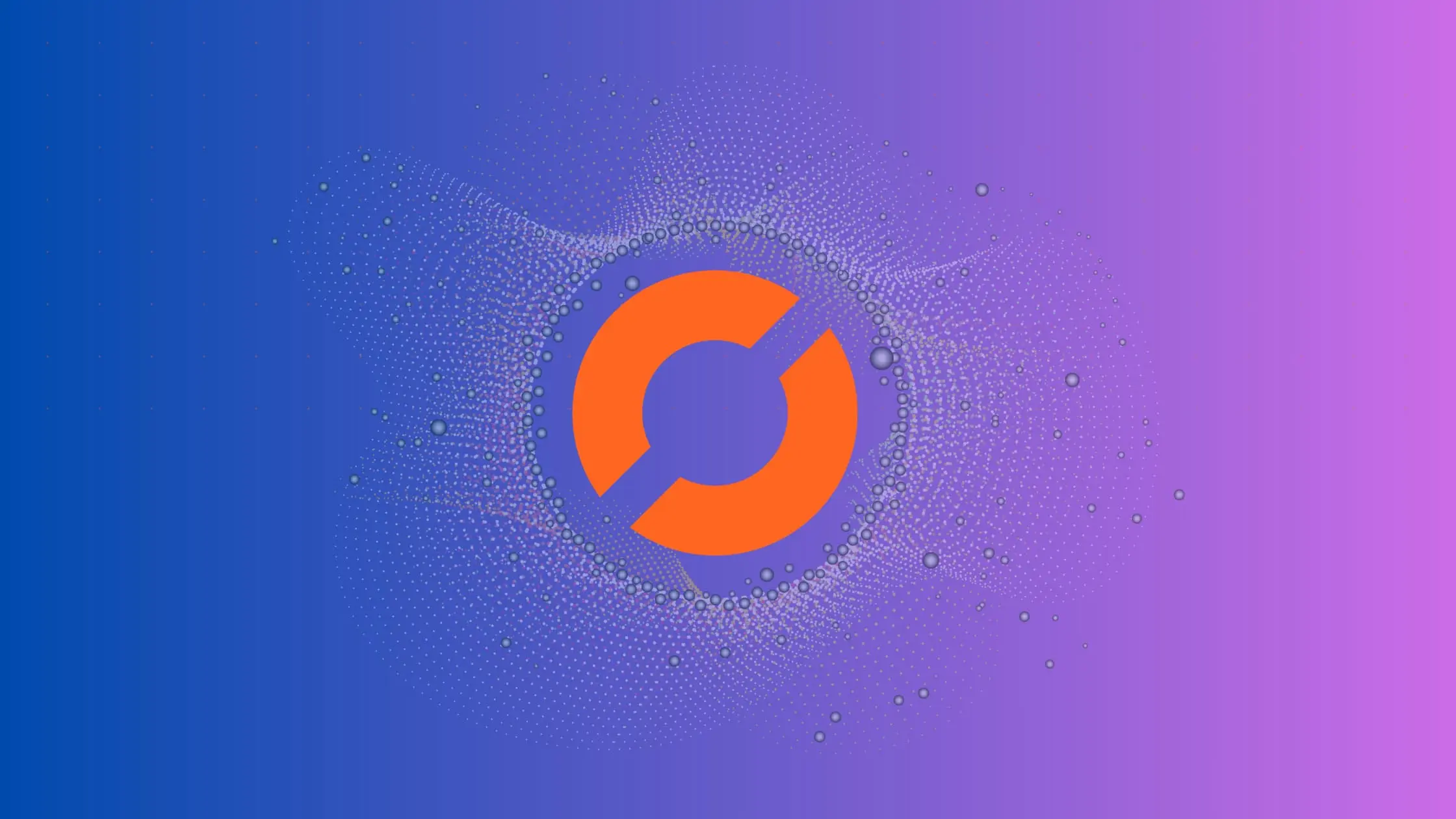
As a data scientist or software engineer, one of the most common tasks you will encounter is searching a pandas data frame for specific values. While pandas provides a variety of powerful methods for querying data frames, it can be challenging to search for data based on multiple criteria. In this article, we will explore how to search a pandas data frame by index value and value in any column.
Table of Contents
- Introduction
- Searching by Index Value
- Searching by Column Value
- Searching by Index Value and Column Value
- Pros and Cons
- Error Handling
- Conclusion
Background
Pandas is a popular data analysis library for Python that provides powerful data structures for working with tabular data. The primary data structure in pandas is the data frame, which is essentially a two-dimensional table with rows and columns.
When working with data frames, it is often necessary to find specific rows that meet certain criteria. For example, you might need to find all rows where a certain column has a particular value, or where multiple columns meet specific conditions. In pandas, you can use boolean indexing to filter data frames based on these types of criteria.
Searching by Index Value
The index of a pandas data frame is the row labels that provide a unique identifier for each row in the data frame. By default, the index values are integers that start at 0 and increase by 1 for each row. However, you can also set the index to be a column of the data frame or a combination of columns.
To search a pandas data frame by index value, you can use the .loc[]
method. The .loc[]
method allows you to select rows and columns by label, and it can accept a variety of input formats.
Here is an example that demonstrates how to search a pandas data frame by index value:
import pandas as pd
# create a sample data frame
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'salary': [50000, 60000, 70000, 80000]
})
# set the index to be the names column
df.set_index('name', inplace=True)
# search for rows with index value 'Bob'
result = df.loc['Bob']
print(result)
Output:
age 30
salary 60000
Name: Bob, dtype: int64
In this example, we first create a sample data frame with three columns: name, age, and salary. We then set the index of the data frame to be the names column using the .set_index()
method. Finally, we use the .loc[]
method to search for rows with index value ‘Bob’.
Searching by Column Value
To search a pandas data frame by column value, you can also use boolean indexing. Boolean indexing allows you to filter rows based on a boolean condition. The condition is specified as a sequence of True/False values that correspond to each row in the data frame.
Here is an example that demonstrates how to search a pandas data frame by column value:
import pandas as pd
# create a sample data frame
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'salary': [50000, 60000, 70000, 80000]
})
# search for rows where age is greater than 30
result = df[df['age'] > 30]
print(result)
Output:
name age salary
2 Charlie 35 70000
3 David 40 80000
In this example, we create a sample data frame with three columns: name, age, and salary. We then use boolean indexing to search for rows where the age column is greater than 30.
Searching by Index Value and Column Value
To search a pandas data frame by both index value and column value, you can combine the .loc[]
method and boolean indexing. The .loc[]
method allows you to select rows and columns by label, while boolean indexing allows you to filter rows based on a boolean condition.
Here is an example that demonstrates how to search a pandas data frame by index value and column value:
import pandas as pd
# create a sample data frame
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'salary': [50000, 60000, 70000, 80000]
})
# set the index to be the names column
df.set_index('name', inplace=True)
# search for rows with index value 'Bob' and salary greater than 50000
result = df.loc[(df.index == 'Bob') & (df['salary'] > 50000)]
print(result)
Output:
age salary
name
Bob 30 60000
In this example, we first create a sample data frame with three columns: name, age, and salary. We then set the index of the data frame to be the names column using the .set_index()
method. Finally, we use the .loc[]
method to search for rows with index value ‘Bob’ and boolean indexing to filter rows where the salary column is greater than 50000.
Cons
- Complexity with Multiple Conditions:
- Issue: When searching for data based on multiple criteria, the combination of boolean indexing and the .loc[] method can lead to complex and verbose code.
- Impact: This complexity may make the code harder to understand and maintain, especially as the number of conditions increases.
- Performance Concerns:
- Issue: Depending on the size of the data frame, searching using boolean indexing and .loc[] may have performance implications.
- Impact: For large data sets, the execution time of these operations could be considerable. Optimizing performance may require additional techniques or libraries.
- Potential for Ambiguity:
- Issue: When combining multiple conditions, there’s a potential for creating ambiguous queries that may not yield the expected results.
- Impact: Ambiguity can lead to errors in data retrieval, potentially impacting the accuracy of analysis or downstream processes.
Pros
- Flexibility in Criteria:
- Benefit: The combination of boolean indexing and .loc[] provides flexibility in specifying complex search criteria, allowing for precise data retrieval.
- Impact: This flexibility is valuable when dealing with diverse datasets that require nuanced queries.
- Readability for Single Criteria:
- Benefit: For simple queries involving a single criterion, the code is concise and readable.
- Impact: This readability enhances the ease of understanding for straightforward use cases.
- Integration with Pandas Ecosystem:
- Benefit: The methods discussed are native to pandas, ensuring seamless integration with other pandas functionalities.
- Impact: This integration facilitates a consistent and familiar workflow for users already accustomed to pandas.
Error Handling:
- Handling Missing Index or Column Values:
- Strategy: Before performing searches, ensure that the specified index or column values exist in the data frame.
- Example: Add checks using the .index or .columns attributes to verify the existence of specified labels.
- Dealing with Nonexistent Criteria:
- Strategy: Implement checks to handle scenarios where the specified criteria do not match any rows.
- Example: Use conditional statements to print a message or take alternative actions when no matching rows are found.
- Avoiding Data Type Mismatches:
- Strategy: Ensure that the data types of the values being compared match, especially when using boolean indexing with numerical or categorical criteria.
- Example: Use functions like .astype() to explicitly convert data types when needed.
- Error Logging for Large Datasets:
- Strategy: For large datasets, implement error logging to capture any unexpected issues during the search process.
- Example: Utilize Python’s logging module to record errors and warnings.
Conclusion
Searching a pandas data frame by index value and column value is an essential task for any data scientist or software engineer working with tabular data. In this article, we explored how to search a pandas data frame by index value and value in any column using boolean indexing and the .loc[]
method. By combining these techniques, you can quickly and efficiently find specific rows that meet the criteria you are looking for.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.