How to Round Numbers with Pandas
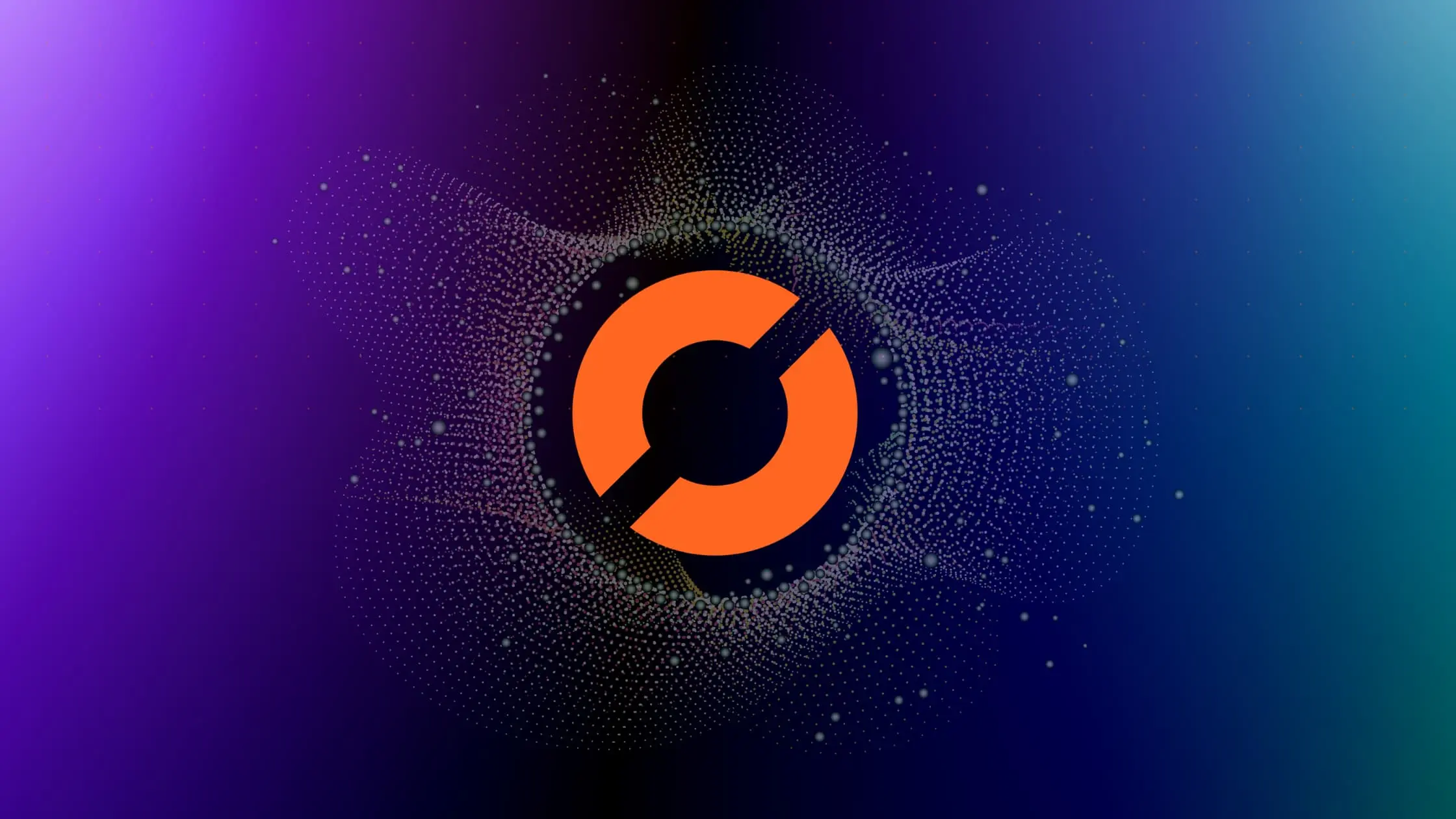
Table of Contents
- 2.1 Round to a Specific Number of Decimal Places
- 2.2 Round to the Nearest Integer
- 2.3 Round Up or Down
Introduction to Pandas
Pandas is an open-source data manipulation library for Python. It is widely used in data science for tasks such as data cleaning, data analysis, and data visualization. Pandas provides data structures for efficiently storing and manipulating large datasets, as well as tools for working with missing data, time series, and other common data types.
Rounding Numbers with Pandas
Pandas provides several functions for rounding numbers, depending on your specific needs. Let’s take a look at some of the most commonly used functions.
Round to a Specific Number of Decimal Places
To round a number to a specific number of decimal places, you can use the round()
function. The round()
function takes two arguments: the number you want to round, and the number of decimal places you want to round to. Here’s an example:
import pandas as pd
# create a pandas DataFrame with some numerical data
df = pd.DataFrame({'a': [1.23456789, 2.34567890, 3.45678901]})
# round the numbers to 2 decimal places
df['a'] = df['a'].round(2)
# print the DataFrame
print(df)
Output:
a
0 1.23
1 2.35
2 3.46
In this example, we create a pandas DataFrame with some numerical data in a column called a
. We then use the round()
function to round the numbers in that column to 2 decimal places.
Round to the Nearest Integer
To round a number to the nearest integer, you can use the astype()
function with an argument of int
. This will change the datatype of the values into integers truncating the decimal part, effectively converting the values to integers Here’s an example:
import pandas as pd
# create a pandas DataFrame with some numerical data
df = pd.DataFrame({'a': [1.4, 2.6, 3.5]})
# round the numbers to the nearest integer
df['a'] = df['a'].astype(int)
# print the DataFrame
print(df)
Output:
a
0 1
1 3
2 4
In this example, we create a pandas DataFrame with some numerical data in a column called a
. We then use the astype()
function to convert and round the numbers in that column to the nearest integer.
Round Up or Down
In some cases, you may want to round a number up or down to the nearest integer or a specific decimal place. Pandas and math provides several functions for this:
ceil()
: rounds up to the nearest integer or decimal placefloor()
: rounds down to the nearest integer or decimal place
Here’s an example of using ceil()
to round up to the nearest integer:
import pandas as pd
# create a pandas DataFrame with some numerical data
df = pd.DataFrame({'a': [1.4, 2.6, 3.5]})
# round the numbers up to the nearest integer
df['a'] = df['a'].apply(lambda x: math.ceil(x))
# print the DataFrame
print(df)
Output:
a
0 2
1 3
2 4
In this example, we create a pandas DataFrame with some numerical data in a column called a
. We then use the apply()
function with a lambda function to apply the ceil()
function to each number in that column, rounding up to the nearest integer.
Similarly, you can use floor()
to round down to the nearest integer or decimal place.
Pros and Cons
Method | Pros | Cons |
---|---|---|
round() | 1- Easy to use. | 1- Can be cumbersome for adjustments. |
2- Control over decimal places. | 2- Might lose precision for large values | |
3- Efficient for small to medium datasets | ||
astype(int) | 1- Quick and efficient | 1- Discards decimal information |
2- Simple for whole numbers rounding | 2- Not suitable for rounding to set decimal places | |
ceil() & floor() | 1- Control over rounding direction | 1- Less commonly used than round() |
2- Useful for certain operations like (min, max) | 2- Requires more methods and lambda exp |
Common Errors
Incorrect usage of arguments: Double-check the number of arguments for each function and their data types.
Unexpected results: Pay attention to data types and potential loss of precision, especially when using astype(int).
Misunderstanding rounding direction: Ensure you’re using the correct function (ceil or floor) for your desired rounding behavior.
Applying rounding to non-numeric data: Make sure you’re only applying rounding functions to columns containing numerical values.
Conclusion
In this article, we explored how to round numbers with pandas. We learned about the round()
function for rounding to a specific number of decimal places, astype()
to convert values to the nearest integer, as well as the ceil()
and floor()
functions for rounding up or down to the nearest integer or decimal place. By using these functions, you can easily manipulate numerical data in pandas to meet your specific needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.