How to Reset Index in a Pandas Dataframe
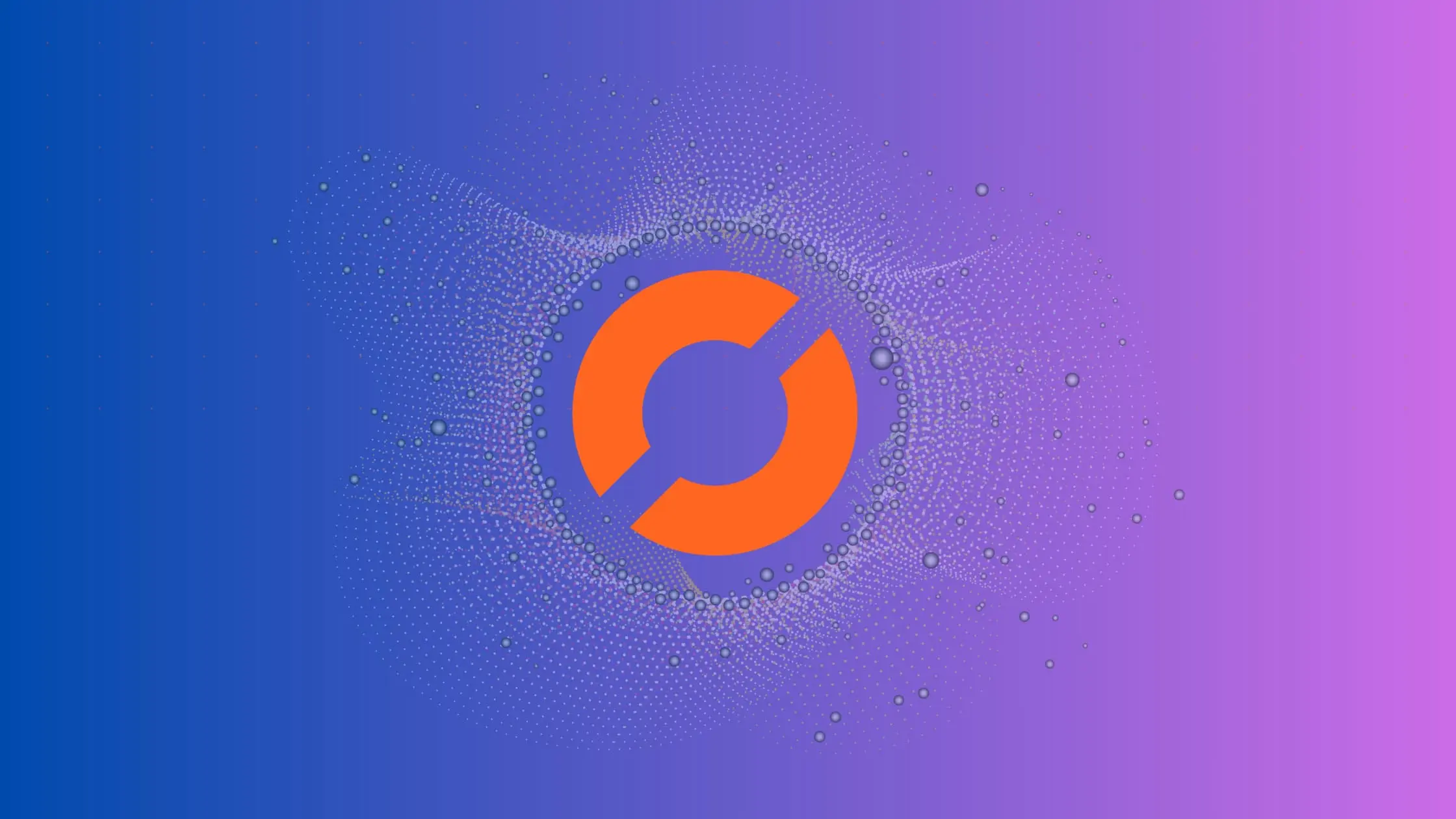
As a data scientist or software engineer, you are likely to work with large datasets, and pandas is one of the most popular libraries for data manipulation in Python. Pandas provides a lot of functionalities to manipulate data, and one of these functionalities is resetting the index of a dataframe. In this article, we will discuss what is index in a pandas dataframe, why we need to reset the index, and how to reset the index in a pandas dataframe.
What is Index in a Pandas Dataframe?
In pandas, index is a way to label rows and columns of a dataframe. The index can be thought of as an address that identifies a specific row or column in a dataframe. By default, pandas assigns a numeric index to a dataframe, starting from 0. However, we can also assign custom labels as an index, such as dates, names or any other relevant information.
For example, let’s create a simple pandas dataframe:
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'salary': [50000, 60000, 70000, 80000]}
df = pd.DataFrame(data)
print(df)
Output:
name age salary
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
3 David 40 80000
In this dataframe, the rows are indexed from 0 to 3, and the columns are labeled with the column names ‘name’, ‘age’, and ‘salary’.
Why We Need to Reset the Index?
There are a few reasons why we might want to reset the index of a pandas dataframe:
Missing or duplicate index values: Sometimes, the index values might be missing or duplicated. In such cases, resetting the index can help to reassign new index values to the dataframe.
Change the order of rows: By default, the rows in a dataframe are ordered by their index values. If we want to change the order of rows based on some other criteria, resetting the index can help to sort the rows based on a different column.
Merge or join dataframes: When we merge or join two or more dataframes, we might end up with duplicate index values. Resetting the index can help to avoid such conflicts.
How to Reset the Index in a Pandas Dataframe?
To reset the index of a pandas dataframe, we can use the reset_index()
method.
df.reset_index()
This method returns a new dataframe with a new numeric index, starting from 0. The original index becomes a new column in the dataframe, labeled with the name ‘index’.
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'salary': [50000, 60000, 70000, 80000]}
df = pd.DataFrame(data)
print(df)
df_reset = df.reset_index()
print(df_reset)
Output:
name age salary
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
3 David 40 80000
index name age salary
0 0 Alice 25 50000
1 1 Bob 30 60000
2 2 Charlie 35 70000
3 3 David 40 80000
As we can see, the original index values have been reset, and a new column ‘index’ has been added to the dataframe.
We can also use the drop
parameter in reset_index()
to drop the original index column:
df_reset = df.reset_index(drop=True)
print(df_reset)
Output:
name age salary
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
3 David 40 80000
In this case, the original index values have been reset, and the column ‘index’ has been dropped from the dataframe.
We can also reset the index based on a specific column in the dataframe. For example, if we want to reset the index based on the ‘salary’ column, we can use the following code:
df_reset = df.set_index('salary').reset_index()
print(df_reset)
Output:
salary name age
0 50000 Alice 25
1 60000 Bob 30
2 70000 Charlie 35
3 80000 David 40
In this case, the ‘salary’ column has become the new index, and the original index values have been reset.
Conclusion
In this article, we discussed what is index in a pandas dataframe, why we need to reset the index, and how to reset the index in a pandas dataframe. By resetting the index, we can avoid conflicts when merging or joining dataframes, change the order of rows, and reassign new index values to the dataframe. Pandas provides a simple and straightforward method to reset the index, which can be customized based on specific requirements. As a data scientist or software engineer, understanding how to reset the index in a pandas dataframe is an essential skill that can help to manipulate data effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.