How to Replace Values on Specific Columns in Pandas
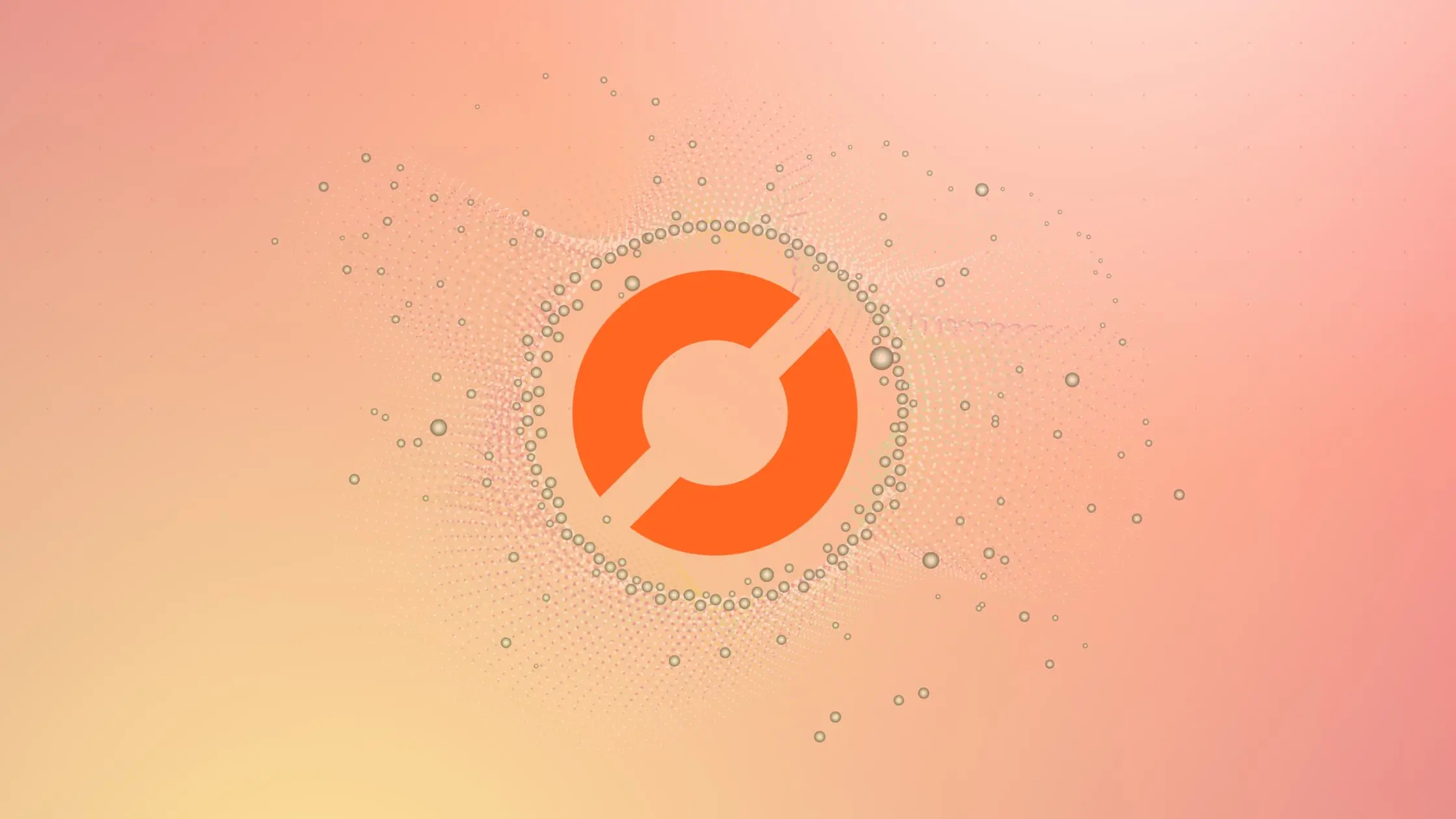
As a data scientist or software engineer, you know that working with data is not always straightforward. Often, you need to clean and preprocess the data before you can start analyzing it. One common task in data preprocessing is replacing values on specific columns. In this article, we will show you how to do this using Pandas, a popular data manipulation library in Python.
What is Pandas?
Pandas is an open-source library for data manipulation and analysis in Python. It provides data structures for efficiently storing and manipulating large datasets and tools for data cleaning, transformation, and analysis. Pandas is built on top of NumPy, another popular numerical computing library in Python.
Pandas provides two main data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, while a DataFrame is a two-dimensional table-like structure that can hold multiple Series with different data types. In this article, we will focus on the DataFrame data structure.
Why Replace Values in Specific Columns?
Replacing values in specific columns is crucial in data preprocessing and data cleaning tasks. There are several reasons you might need to replace values:
Data Correction: Correcting errors, inconsistencies, or inaccuracies in your dataset. For example, fixing typos, removing outliers, or converting units of measurement.
Data Standardization: Bringing data into a consistent format. For instance, converting all date formats to a uniform style or normalizing text data to lowercase.
Data Transformation: Converting data types to better suit your analysis. This could involve changing strings to numerical values or replacing missing values with appropriate substitutes.
Data Masking: Replacing sensitive information with placeholders or pseudonyms to protect privacy.
The Pandas DataFrame
Before diving into replacing values, it’s essential to understand the Pandas DataFrame, which is the primary data structure used in Pandas. A DataFrame is a 2-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). In simpler terms, it’s like a spreadsheet where each column can have a different data type.
To work with Pandas, you first need to import it and load your data into a DataFrame. Here’s how you can do that:
import pandas as pd
# Load data into a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'City': ['New York', 'Los Angeles', 'San Francisco', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
Output:
name age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 San Francisco
3 David 40 Chicago
Now, let’s explore various methods for replacing values in specific columns.
Using the .replace()
Method
The .replace()
method is a versatile way to replace values in a DataFrame. You can specify the column, the value to replace, and the replacement value. Here’s an example:
# Replace 'New York' with 'NY' in the 'City' column
df_copy = df.copy()
df_copy['City'].replace('New York', 'NY', inplace=True)
print(df_copy)
Output:
name age City
0 Alice 25 NY
1 Bob 30 Los Angeles
2 Charlie 35 San Francisco
3 David 40 Chicago
This code snippet will replace all occurrences of New York
in the City
column with NY
. Setting inplace=True
modifies the original DataFrame; otherwise, it returns a new DataFrame with the replacements.
Using .loc[]
for Conditional Replacement
You can use .loc[]
to replace values based on a condition. For instance, let’s say you want to replace ages greater than 30 with a default value of 30:
# Replace ages greater than 30 with 30
df_copy = df.copy()
df_copy.loc[df['Age'] > 30, 'Age'] = 30
print(df_copy)
Output:
name age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 30 San Francisco
3 David 30 Chicago
Using apply()
with a Custom Function
You can also use the apply()
function with a custom function for more complex replacements. Here’s an example where we replace city names with their respective state names:
state_mapping = {
'New York': 'NY',
'Los Angeles': 'CA',
'San Francisco': 'CA',
'Chicago': 'IL'
}
# Define a custom function
def replace_city_with_state(city):
return state_mapping.get(city, city)
# Apply the custom function to the 'City' column
df_copy = df.copy()
df_copy['City'] = df_copy['City'].apply(replace_city_with_state)
print(df_copy)
This method is especially useful when you need to perform replacements that are not straightforward to express with a single value-to-value mapping.
Output:
name age City
0 Alice 25 NY
1 Bob 30 CA
2 Charlie 30 CA
3 David 30 IL
Using str.replace()
for String Columns
If you want to replace values within string columns, you can use the .str.replace()
method. For instance, let’s replace Alice
with Alicia
in the Name
column:
df_copy = df.copy()
df_copy['Name'] = df['Name'].str.replace('Alice', 'Alicia')
print(df_copy)
Output:
name age City
0 Alicia 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 San Francisco
3 David 40 Chicago
Handling Missing Values
Replacing missing or NaN
values in a specific column is a common data preprocessing task. You can use the .fillna()
method to replace NaN
values with a specific value:
import numpy as np
import pandas as pd
# Load data into a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Marie'],
'Age': [25, 30, 35, 40, np.nan],
'City': ['New York', 'Los Angeles', 'San Francisco', 'Chicago', 'Washington']
}
df = pd.DataFrame(data)
print(df)
Output:
name age City
0 Alicia 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 San Francisco
3 David 40 Chicago
4 Marie NaN Washington
mean_age = df['Age'].mean()
df['Age'].fillna(mean_age, inplace=True)
print(df)
Output:
name age City
0 Alicia 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 San Francisco
3 David 40 Chicago
4 Marie 32.5 Washington
Most of the time, df.replace()
will get the job done, yet, there are situations where seemingly straightforward operations can lead to unexpected results where assignment to a DataFrame using the df.replace
method fails to replace values. Let’s assume we have a DataFrame with a column of numbers:
import pandas as pd
data = {'Column1': [1, 2, 3],
'Column2': ['A', 'B', 'C']}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
# Attempt to replace a value, but it fails
df.replace(2, 6)
print("\nDataFrame after attempted replacement:")
print(df)
In this example, we have a DataFrame with values in column A
. We attempt to replace the value 2
with 6
using df.replace(2, 6)
. However, if you print the DataFrame after the replacement, you’ll notice that the replacement hasn’t occurred:
Original DataFrame:
Column1 Column2
0 1 A
1 2 B
2 3 C
DataFrame after attempted replacement:
Column1 Column2
0 1 A
1 2 B
2 3 C
The reason for this failure is that df.replace returns a new DataFrame with the replacement values, but it doesn’t modify the original DataFrame in place. To achieve the desired replacement, you should either assign the result back to the original DataFrame or use the inplace=True
parameter. Here’s how you can do it:
# Assign the result back to the original DataFrame
df = df.replace(2, 6)
# Or, use the inplace parameter
df.replace(2, 6, inplace=True)
print(df)
Either way, it will return an output like this:
Column1 Column2
0 1 A
1 6 B
2 3 C
Conclusion
Replacing values in specific columns is an essential data preprocessing step when working with datasets in Pandas. Whether you need to correct errors, standardize data, or transform it for analysis, Pandas offers a range of methods to achieve these tasks. By mastering these techniques, you can ensure your data is clean, consistent, and ready for further analysis, ultimately helping you derive meaningful insights and make informed decisions.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.