How to Rename MultiIndex Columns in Pandas A Guide for Data Scientists
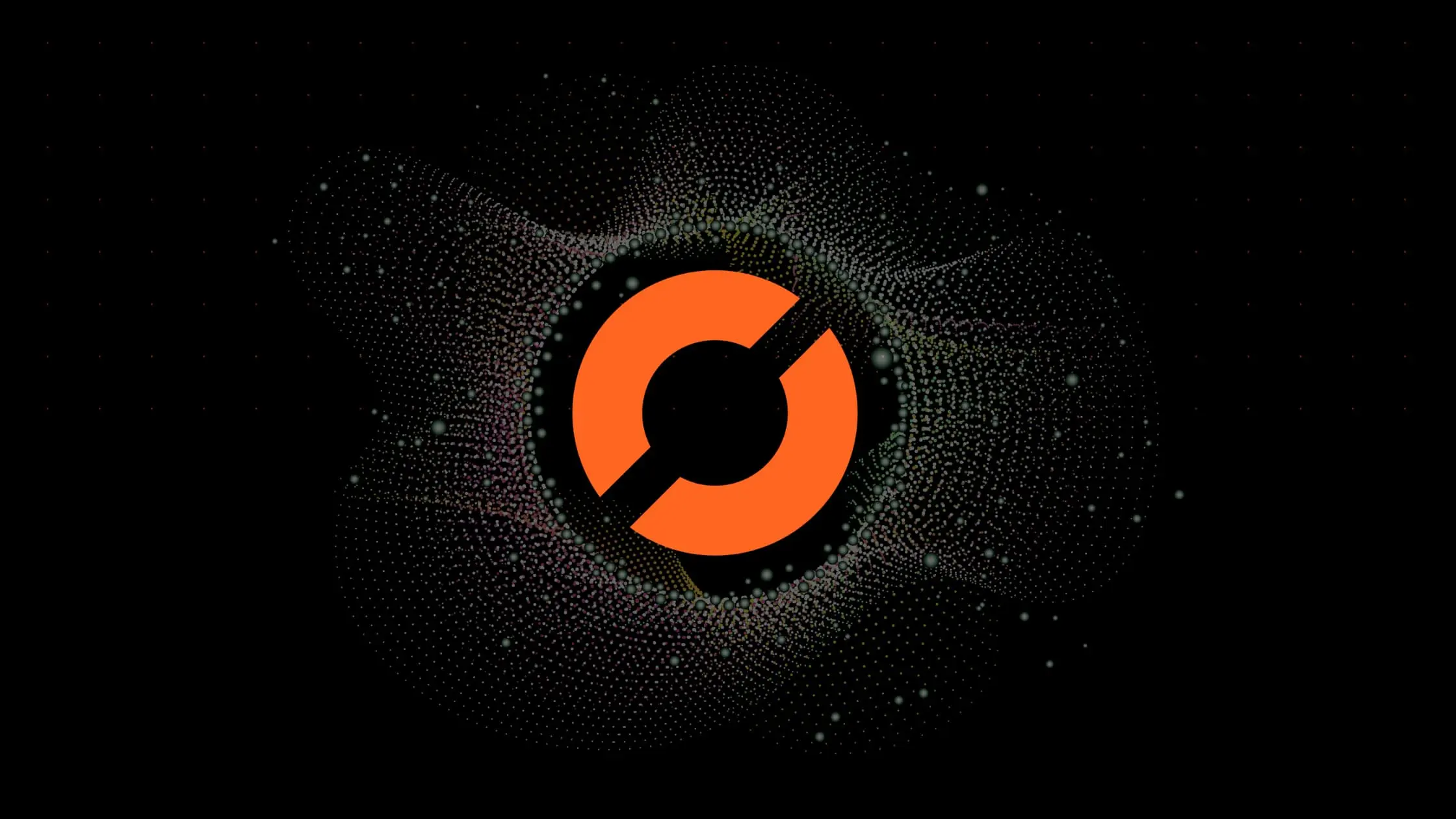
As a data scientist, you know that working with large datasets can be challenging. One of the common scenarios in data analysis is when you have multiple levels of indexing in your DataFrame. In such cases, you may need to rename MultiIndex columns in Pandas to make your data more readable and easier to work with.
In this blog post, we will explain how to rename MultiIndex columns in Pandas. We will start with a brief introduction to MultiIndex and its importance in data analysis. Then we will move on to the main topic of this blog post, which is how to rename MultiIndex columns in Pandas.
Table of Contents
- Introduction
- What is MultiIndex in Pandas?
- Why Rename MultiIndex Columns in Pandas?
- How to Rename MultiIndex Columns in Pandas
- Pros and Cons of Renaming MultiIndex Columns in Pandas
- Error Handling
- Conclusion
What is MultiIndex in Pandas?
In Pandas, MultiIndex is a way to represent hierarchical indexing for DataFrame and Series objects. It allows you to index and group data using multiple levels of labels. For example, you can use MultiIndex to represent a dataset that contains data for multiple years, months, and days. In this case, the first level of the MultiIndex represents the year, the second level represents the month, and the third level represents the day.
MultiIndex is a powerful tool that enables you to perform complex data analysis tasks such as grouping, filtering, and aggregating data. However, it can be challenging to work with MultiIndex dataframes if you don’t know how to navigate and manipulate them properly.
Why Rename MultiIndex Columns in Pandas?
When you have MultiIndex columns in your DataFrame, the column names can be complex and difficult to read. Renaming MultiIndex columns in Pandas can make your data more readable and easier to work with. It can also help you to standardize column names across different datasets.
Moreover, when you are exporting your data to other formats such as Excel or CSV, having readable column names can make it easier for others to understand and use your data.
How to Rename MultiIndex Columns in Pandas
Renaming MultiIndex columns in Pandas is straightforward. You can use the rename()
method to rename columns in your DataFrame. The rename()
method takes a dictionary that maps the old column names to new column names.
Here’s an example:
import pandas as pd
# Create a MultiIndex DataFrame
data = {'A': [1, 2, 3, 4], 'B': [5, 6, 7, 8], 'C': [9, 10, 11, 12], 'D': [13, 14, 15, 16]}
index = pd.MultiIndex.from_tuples([('foo', 'one'), ('foo', 'two'), ('bar', 'one'), ('bar', 'two')], names=['first', 'second'])
df = pd.DataFrame(data, index=index)
# Rename MultiIndex columns
df = df.rename(columns={'A': 'new_A', 'B': 'new_B', 'C': 'new_C', 'D': 'new_D'})
print(df)
Output:
new_A new_B new_C new_D
first second
foo one 1 5 9 13
two 2 6 10 14
bar one 3 7 11 15
two 4 8 12 16
In this example, we create a MultiIndex DataFrame and then use the rename()
method to rename the columns. The columns
parameter of the rename()
method takes a dictionary that maps the old column names to new column names. The resulting DataFrame will have the new column names.
Pros and Cons of Renaming MultiIndex Columns in Pandas
Pros
Clarity and Readability: Renaming MultiIndex columns improves the clarity and readability of the DataFrame. This is crucial for understanding and interpreting the data, especially when working with complex datasets.
Consistency Across Datasets: Renaming allows for standardization of column names across different datasets. This consistency simplifies the process of merging or comparing data from various sources.
Ease of Export: When exporting data to other formats such as Excel or CSV, having readable column names enhances the usability of the data for others. It makes the exported data more user-friendly and accessible.
Simplicity in Code: The provided example demonstrates that the process of renaming MultiIndex columns in Pandas is straightforward and can be achieved with a concise code snippet. This simplicity is beneficial for developers and data scientists.
Cons
Potential for Error: If not done carefully, renaming columns can introduce errors. The dictionary used for renaming must accurately map old column names to new ones. Mistakes in this mapping can lead to misinterpretation of data.
Overhead in Large Datasets: For exceptionally large datasets, the process of renaming MultiIndex columns may introduce a computational overhead. While Pandas is optimized for performance, it’s essential to be mindful of the potential impact on processing time for extensive datasets.
Error Handling
Input Validation: Implement input validation checks to ensure that the provided dictionary for renaming contains valid column names. This helps catch potential errors before the renaming process begins.
Backup Original Data: Before applying the rename operation, create a backup of the original DataFrame. This ensures that if an error occurs during the renaming process, you can revert to the original data without loss.
Try-Except Blocks: Implement try-except blocks to catch and handle exceptions during the renaming process. This can include catching key errors if a column specified for renaming does not exist in the DataFrame.
Logging: Use logging mechanisms to record the renaming operations and any potential errors. This information can be valuable for debugging and auditing purposes.
Testing: Before applying renaming to the entire dataset, conduct testing on a small subset to validate the correctness of the renaming logic. This reduces the risk of errors on the entire dataset.
Conclusion
Working with MultiIndex columns in Pandas can be challenging, especially when dealing with large datasets. Renaming MultiIndex columns can make your data more readable and easier to work with. In this blog post, we have explained how to rename MultiIndex columns in Pandas using the rename()
method.
By following the steps outlined in this blog post, you can easily rename MultiIndex columns in your DataFrame and make your data more understandable and useful. We hope that this guide has been helpful in your data analysis journey.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.